


Using event dispatching mechanism to achieve decoupling in PHP
Using event dispatching mechanism to achieve decoupling in PHP
In PHP development, decoupling is a very important concept. Decoupling refers to minimizing the dependencies of each module of the system so that each module of the system can be developed, tested and deployed independently. This can improve the maintainability and scalability of the system. The event dispatch mechanism is a commonly used decoupling method, which can achieve decoupling between modules in the system.
The event dispatch mechanism is a design pattern based on the observer pattern. In this mode, each module in the system can register the events it cares about and define corresponding processing functions. When an event occurs, the system will notify all modules that care about the event and let them execute the corresponding processing logic. Through the event dispatch mechanism, communication between different modules becomes very simple and flexible.
The following is a simple example to illustrate how to use the event dispatch mechanism to achieve decoupling in PHP.
First, we need to create an event manager class EventManager, which is used to manage the events registered by each module and the corresponding processing functions. The specific code is as follows:
class EventManager { private $events = []; public function registerEvent($eventName) { $this->events[$eventName] = []; } public function attach($eventName, $handler) { $this->events[$eventName][] = $handler; } public function detach($eventName, $handler) { foreach ($this->events[$eventName] as $key => $value) { if ($value == $handler) { unset($this->events[$eventName][$key]); break; } } } public function trigger($eventName, $data) { foreach ($this->events[$eventName] as $handler) { call_user_func($handler, $data); } } }
In the above code, the registerEvent method is used to register an event, the attach method is used to add a processing function to the event, the detach method is used to remove the processing function from the event, and the trigger method is used to trigger event and execute the corresponding processing function.
Next, we create a module A. Module A cares about event A and defines the processing function handleEventA. The specific code is as follows:
class ModuleA { public function handleEventA($data) { echo "Module A handles Event A: " . $data . " "; } }
Then, we create a module B. Module B cares about event B and defines the processing function handleEventB. The specific code is as follows:
class ModuleB { public function handleEventB($data) { echo "Module B handles Event B: " . $data . " "; } }
Finally, we use the above code to create the event manager object and two module objects, register the event and add the corresponding processing function. We then trigger the event through the event manager and pass the relevant data. The specific code is as follows:
$eventManager = new EventManager(); $moduleA = new ModuleA(); $moduleB = new ModuleB(); $eventManager->registerEvent('EventA'); $eventManager->attach('EventA', [$moduleA, 'handleEventA']); $eventManager->registerEvent('EventB'); $eventManager->attach('EventB', [$moduleB, 'handleEventB']); $data = "Hello, World!"; $eventManager->trigger('EventA', $data); $eventManager->trigger('EventB', $data);
Run the above code, you can see the output as follows:
Module A handles Event A: Hello, World! Module B handles Event B: Hello, World!
Through the above code, we can see that module A and module B register events with the event manager , and add corresponding processing functions to achieve decoupling of events. When an event occurs, the event manager will notify the corresponding module to execute the corresponding processing function. The communication between modules no longer directly depends on each other, achieving decoupling between modules.
To sum up, using the event dispatch mechanism to achieve decoupling in PHP is a very effective and flexible way. Through the registration and triggering of events, communication between different modules becomes simple and loosely coupled, improving the maintainability and scalability of the system. Therefore, the event dispatch mechanism should be fully utilized during the development process to decouple the various modules of the system.
The above is the detailed content of Using event dispatching mechanism to achieve decoupling in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


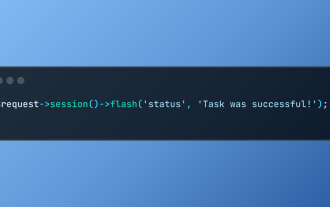
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
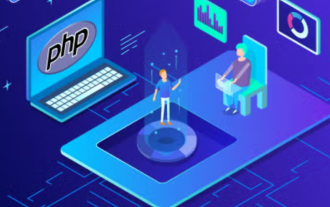
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
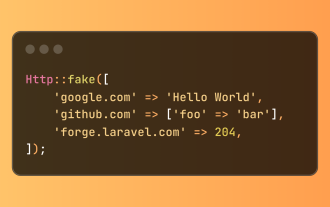
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
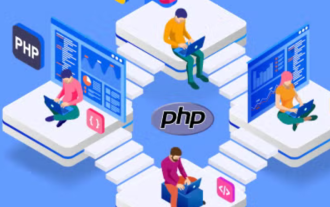
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
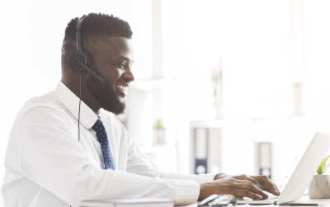
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
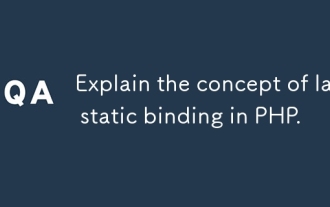
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
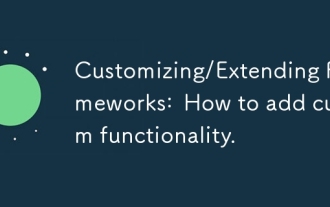
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
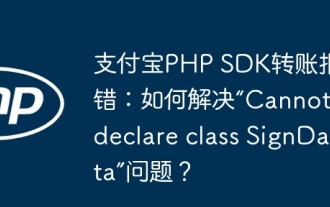
Alipay PHP...
