How to implement asynchronous message handling in PHP
How to implement asynchronous message processing in PHP
Introduction:
In modern web applications, asynchronous message processing is becoming more and more important. Asynchronous message processing can improve the performance and scalability of the system and improve the user experience. As a commonly used server-side programming language, PHP can also implement asynchronous message processing through some technologies. In this article, we will introduce some methods of implementing asynchronous message processing in PHP and provide code examples.
- Using message queue
Message queue is a way of decoupling system components, which allows different components to communicate asynchronously without communicating directly. In PHP, we can use some popular message queue services, such as RabbitMQ, Beanstalkd, etc. The following is a sample code using RabbitMQ:
<?php require_once __DIR__ . '/vendor/autoload.php'; use PhpAmqpLibConnectionAMQPStreamConnection; use PhpAmqpLibMessageAMQPMessage; // 建立与RabbitMQ的连接 $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); // 声明一个名为"hello"的队列 $channel->queue_declare('hello', false, false, false, false); // 定义一个回调函数来处理消息 $callback = function ($msg) { echo 'Received message: ' . $msg->body . PHP_EOL; }; // 消费消息 $channel->basic_consume('hello', '', false, true, false, false, $callback); // 监听消息队列 while ($channel->is_consuming()) { $channel->wait(); } $channel->close(); $connection->close();
In the above example, we first establish a connection with RabbitMQ and declare a queue named "hello". Then, we define a callback function to handle messages received from the queue. Finally, we loop through the message queue to listen to the message queue and process the received messages.
- Using asynchronous HTTP requests
In addition to using message queues, we can also use asynchronous HTTP requests to implement asynchronous message processing. PHP provides some libraries and frameworks to help us send asynchronous HTTP requests, such as Guzzle, ReactPHP, etc. Here is a sample code that uses Guzzle to send an asynchronous HTTP request:
<?php require_once __DIR__ . '/vendor/autoload.php'; use GuzzleHttpClient; use GuzzleHttpPromise; // 创建一个Guzzle客户端 $client = new Client(); // 创建异步请求 $promises = [ 'request1' => $client->getAsync('http://example.com/api/endpoint1'), 'request2' => $client->getAsync('http://example.com/api/endpoint2'), 'request3' => $client->getAsync('http://example.com/api/endpoint3'), ]; // 发送异步请求并等待所有请求完成 $results = Promiseunwrap($promises); // 处理请求结果 foreach ($results as $key => $response) { echo 'Response of ' . $key . ': ' . $response->getBody()->getContents() . PHP_EOL; }
In the above example, we first created a Guzzle client and created three asynchronous requests in the promises array. We then use the Promiseunwrap() method to send asynchronous requests and wait for all requests to complete. Finally, we loop through the request results and process them.
Conclusion:
Asynchronous message processing is very important to improve the performance and scalability of the system and improve the user experience. In PHP, we can implement asynchronous message processing using message queues or asynchronous HTTP requests. This article introduces code examples using RabbitMQ and Guzzle, I hope it will be helpful to readers.
Reference materials:
- RabbitMQ official documentation: https://www.rabbitmq.com/documentation.html
- Guzzle official documentation: https://docs .guzzlephp.org/en/stable/
The above code examples are for reference only, readers can adjust and optimize according to actual needs. Thank you for reading!
The above is the detailed content of How to implement asynchronous message handling in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


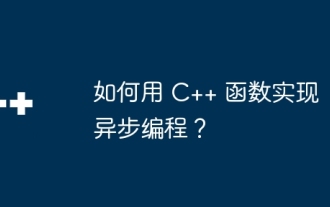
Summary: Asynchronous programming in C++ allows multitasking without waiting for time-consuming operations. Use function pointers to create pointers to functions. The callback function is called when the asynchronous operation completes. Libraries such as boost::asio provide asynchronous programming support. The practical case demonstrates how to use function pointers and boost::asio to implement asynchronous network requests.
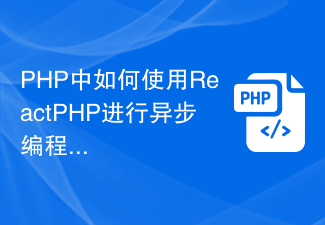
As web applications become more complex, programmers are forced to adopt asynchronous programming to handle large numbers of requests and I/O operations. PHP: HypertextPreprocessor is no exception. To meet this need, ReactPHP has become one of the most popular asynchronous programming frameworks for PHP. In this article, we will discuss how to do asynchronous programming in PHP using ReactPHP. 1. Introduction to ReactPHP ReactPHP is an event-driven programming
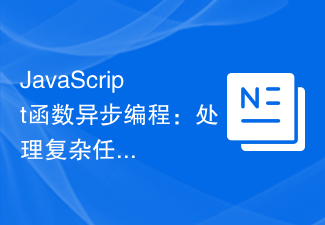
JavaScript Function Asynchronous Programming: Essential Skills for Handling Complex Tasks Introduction: In modern front-end development, handling complex tasks has become an indispensable part. JavaScript function asynchronous programming skills are the key to solving these complex tasks. This article will introduce the basic concepts and common practical methods of JavaScript function asynchronous programming, and provide specific code examples to help readers better understand and use these techniques. 1. Basic concepts of asynchronous programming In traditional synchronous programming, the code is
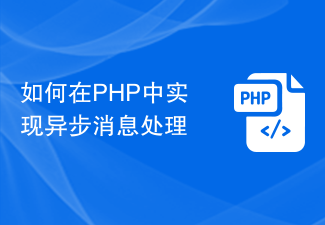
How to implement asynchronous message processing in PHP Introduction: In modern web applications, asynchronous message processing is becoming more and more important. Asynchronous message processing can improve the performance and scalability of the system and improve the user experience. As a commonly used server-side programming language, PHP can also implement asynchronous message processing through some technologies. In this article, we will introduce some methods of implementing asynchronous message processing in PHP and provide code examples. Using Message Queuing Message Queuing is a way of decoupling system components, allowing different components to
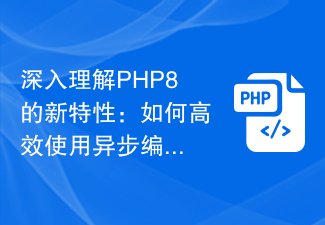
In-depth understanding of the new features of PHP8: How to use asynchronous programming and code efficiently? PHP8 is the latest major version of the PHP programming language, bringing many exciting new features and improvements. One of the most prominent features is support for asynchronous programming. Asynchronous programming allows us to improve performance and responsiveness when dealing with concurrent tasks. This article will take an in-depth look at PHP8’s asynchronous programming features and introduce how to use them efficiently. First, let’s understand what asynchronous programming is. In the traditional synchronous programming model, code follows a linear sequence
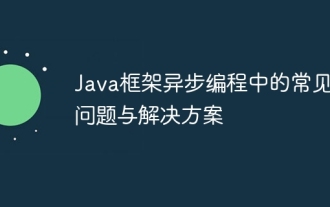
3 common problems and solutions in asynchronous programming in Java frameworks: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
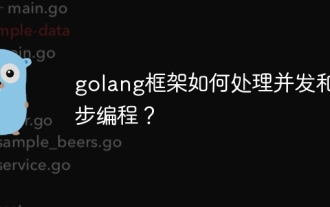
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
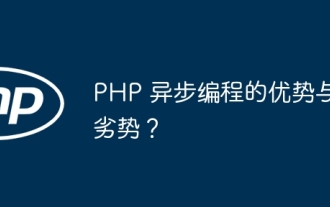
The advantages of asynchronous programming in PHP include higher throughput, lower latency, better resource utilization, and scalability. Disadvantages include complexity, difficulty in debugging, and limited library support. In the actual case, ReactPHP is used to handle WebSocket connections, demonstrating the practical application of asynchronous programming.
