


How to develop Amazon API using PHP to get product information
How to use PHP to develop Amazon API to obtain product information
In recent years, with the rapid development of e-commerce, more and more enterprises and developers have begun to use APIs of third-party cloud platforms to obtain and Use various data. As one of the world's largest e-commerce platforms, Amazon has rich product information data and provides APIs that allow developers to obtain product information on Amazon. This makes it easier for businesses and developers to develop their own applications and services.
This article will introduce how to use PHP to develop Amazon API to obtain product information, and provide corresponding code examples.
First, we need to create a developer account in the Amazon Developer Center and register an application to obtain an API key. After logging into the Amazon Developer Center, click the "My Application" menu, then click the "Create Application" button, fill in the name, description and other information of the application, and select an Amazon API, such as Product Advertising API. Once created, an API key will be generated.
Next, we need to install the AWS SDK for PHP, which is a development toolkit for accessing Amazon services. We can install the AWS SDK through Composer. We only need to execute the following command in the project directory:
composer require aws/aws-sdk-php
After the installation is complete, we can use the AWS SDK to access the Amazon API.
First, we need to create an instance of the Amazon class and set the Amazon API access credentials:
use AwsAwsClient; class Amazon { private $client; public function __construct($accessKey, $secretKey, $associateTag) { $this->client = new AwsClient([ 'version' => 'latest', 'region' => 'us-west-2', // 这里根据实际情况设置亚马逊API的区域 'credentials' => [ 'key' => $accessKey, 'secret' => $secretKey ] ]); $this->associateTag = $associateTag; } }
Next, we can define a method to get product information:
public function getProductInfo($asin) { $params = [ 'AssociateTag' => $this->associateTag, 'ItemId' => $asin, 'ResponseGroup' => 'ItemAttributes,OfferSummary' // 这里可以根据需要选择返回的信息 ]; $result = $this->client->itemLookup($params); return $result; }
Then, we can call this method to obtain product information:
$amazon = new Amazon('your_access_key', 'your_secret_key', 'your_associate_tag'); $asin = 'B00008OE6I'; // 这里可以替换为你要获取信息的产品ASIN $result = $amazon->getProductInfo($asin);
Finally, we can parse the returned results to obtain various information about the product:
$item = $result['Items']['Item']; echo '标题:' . $item['ItemAttributes']['Title'] . PHP_EOL; echo '价格:' . $item['OfferSummary']['LowestNewPrice']['FormattedPrice'] . PHP_EOL; echo '产地:' . $item['ItemAttributes']['Country'] . PHP_EOL; // 其他信息...
When using Amazon When using APIs, you also need to pay attention to the API call frequency and quota limits, as well as the legal use of Amazon product data. During the development and testing phases, it is recommended to use a sandbox environment for testing.
The above is a simple example of using PHP to develop Amazon API to obtain product information. Using Amazon API, we can easily obtain product information on Amazon and provide more data support for our applications and services. Hope this article is helpful to you!
The above is the detailed content of How to develop Amazon API using PHP to get product information. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


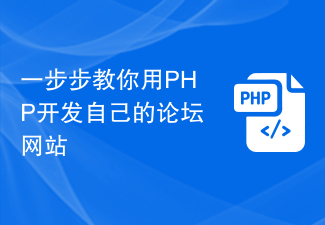
With the rapid development of the Internet and people's increasing demand for information exchange, forum websites have become a common online social platform. Developing a forum website of your own can not only meet your own personalized needs, but also provide a platform for communication and sharing, benefiting more people. This article will teach you step by step how to use PHP to develop your own forum website. I hope it will be helpful to beginners. First, we need to clarify some basic concepts and preparations. PHP (HypertextPreproces
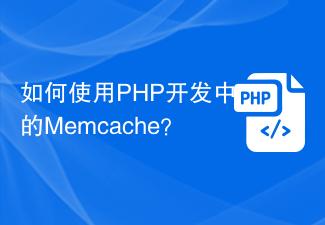
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
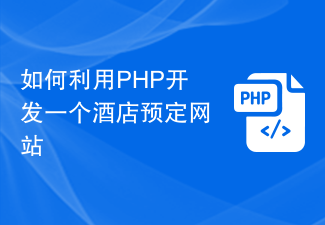
How to use PHP to develop a hotel booking website With the development of the Internet, more and more people are beginning to arrange their travels through online booking. As one of the common online booking services, hotel booking websites provide users with a convenient and fast way to book hotels. This article will introduce how to use PHP to develop a hotel reservation website, allowing you to quickly build and operate your own online hotel reservation platform. 1. System requirements analysis Before starting development, we need to conduct system requirements analysis first to clarify what the website we want to develop needs to have.
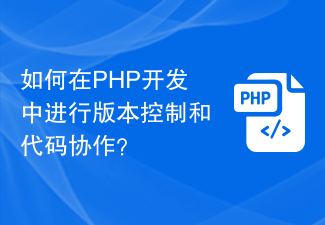
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
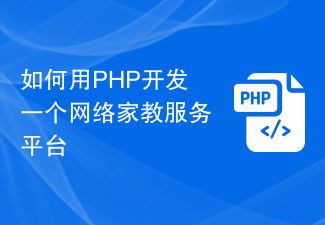
How to use PHP to develop an online tutoring service platform. With the rapid development of the Internet, online tutoring service platforms have attracted more and more people's attention and demand. Parents and students can easily find suitable tutors through such a platform, and tutors can also better demonstrate their teaching abilities and advantages. This article will introduce how to use PHP to develop an online tutoring service platform. First, we need to clarify the functional requirements of the platform. An online tutoring service platform needs to have the following basic functions: Registration and login system: users can
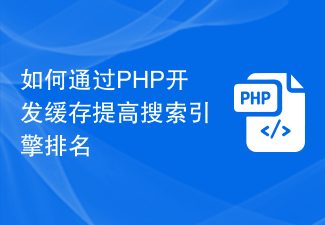
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
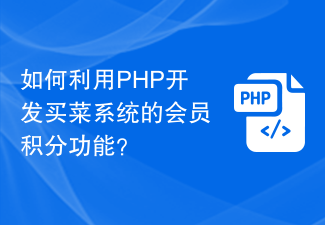
How to use PHP to develop the member points function of the grocery shopping system? With the rise of e-commerce, more and more people choose to purchase daily necessities online, including grocery shopping. The grocery shopping system has become the first choice for many people, and one of its important features is the membership points system. The membership points system can attract users and increase their loyalty, while also providing users with an additional shopping experience. In this article, we will discuss how to use PHP to develop the membership points function of the grocery shopping system. First, we need to create a membership table to store users
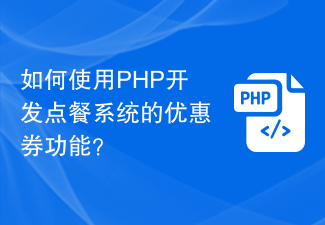
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
