


Real-time inventory management and early warning functions using PHP and MQTT
Use PHP and MQTT to realize real-time inventory management and early warning functions
1. Background introduction
In modern business operations, inventory management is a very important part. Accurate inventory information can help companies understand product quantities and changes in real time, allowing them to make more accurate purchasing and sales decisions. At the same time, the inventory warning function can predict inventory shortages in advance and help companies avoid negative consequences such as stockouts.
2. Technology selection
This article uses PHP as the server-side programming language, and combines it with the MQTT protocol to achieve real-time inventory management and early warning functions. As a commonly used web development language, PHP is easy to learn and use; the MQTT protocol is a lightweight messaging protocol suitable for Internet of Things and mobile application scenarios.
3. Inventory management function implementation
1. Database design
First, create a database named inventory and create a data table named products to store product inventory information. The data table includes the following fields:
- id: product ID
- name: product name
- quantity: product quantity
2. Page design and development
Create a file named index.php to display inventory information. In this file, we can list the names and quantities of all products and provide a form for the user to enter the product names and quantities.
3. Database connection and data reading
In the PHP file, we need to realize the connection with the database and read the product inventory information from the database. The following is a code example for connecting to the database and reading data:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "inventory"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 从数据库中读取产品的库存信息 $sql = "SELECT id, name, quantity FROM products"; $result = $conn->query($sql); if ($result->num_rows > 0) { echo "<table>"; echo "<tr><th>产品ID</th><th>产品名称</th><th>产品数量</th></tr>"; while($row = $result->fetch_assoc()) { echo "<tr><td>".$row["id"]."</td><td>".$row["name"]."</td><td>".$row["quantity"]."</td></tr>"; } echo "</table>"; } else { echo "暂无产品信息"; } $conn->close(); ?>
IV. Implementation of inventory early warning function
1. Set the inventory early warning threshold
In order to implement the inventory early warning function, we need to set an inventory early warning threshold . When the inventory of a product falls below this threshold, the system will issue an early warning message.
2. Send MQTT message
In the PHP file, we can use the MQTT protocol to send warning messages. The following is a code example for sending MQTT messages:
<?php require("phpMQTT.php"); $server = 'localhost'; //MQTT服务器地址 $port = 1883; //MQTT服务器端口号 $username = 'username'; //MQTT用户名 $password = 'password'; //MQTT密码 $mqtt = new phpMQTT($server, $port, "PHP MQTT Client"); if (!$mqtt->connect(true, NULL, $username, $password)) { exit(1); } $topic = 'inventory/alert'; //MQTT主题 $message = '产品A库存不足,请及时补货!'; //预警消息 // 发布MQTT消息 $mqtt->publish($topic, $message, 0, false); $mqtt->close(); ?>
3. Subscribe to MQTT messages
In order to receive MQTT alert messages, we need to subscribe to the corresponding MQTT topic in another PHP file and process the received messages . The following is a code example for subscribing to MQTT messages:
<?php require("phpMQTT.php"); $server = 'localhost'; //MQTT服务器地址 $port = 1883; //MQTT服务器端口号 $username = 'username'; //MQTT用户名 $password = 'password'; //MQTT密码 $mqtt = new phpMQTT($server, $port, "PHP MQTT Client"); if (!$mqtt->connect(true, NULL, $username, $password)) { exit(1); } $topic = 'inventory/alert'; //MQTT主题 // 定义接收消息的回调函数 function messageReceived($topic, $message) { echo "接收到预警消息:[{$topic}] {$message}<br/>"; } // 订阅MQTT主题并设置接收消息的回调函数 $mqtt->subscribe($topic, function($topic, $message){ messageReceived($topic, $message); }, 0); while ($mqtt->proc()) { } $mqtt->close(); ?>
5. Summary
By using PHP and MQTT protocols, we have achieved real-time inventory management and early warning functions. The MQTT protocol can be used to publish and subscribe to real-time messages, and timely send out warning messages when the inventory falls below the warning threshold. This can improve the efficiency of inventory management of enterprises and avoid problems such as stockouts. At the same time, through the design and operation of the database, accurate recording and query of inventory information can be achieved. In actual applications, the code can be modified and optimized according to specific needs to meet more specific business scenarios.
The above is the detailed content of Real-time inventory management and early warning functions using PHP and MQTT. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
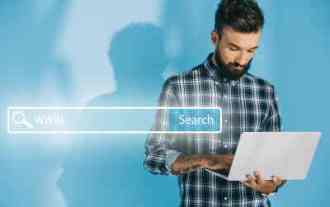
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
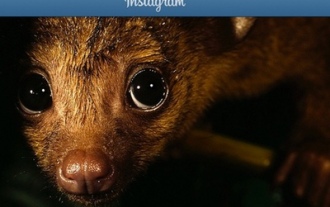
Following its high-profile acquisition by Facebook in 2012, Instagram adopted two sets of APIs for third-party use. These are the Instagram Graph API and the Instagram Basic Display API.As a developer building an app that requires information from a
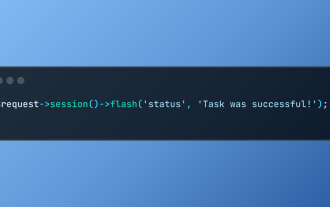
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
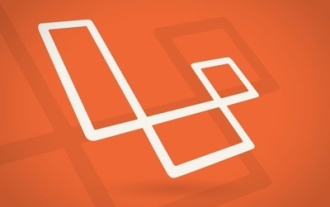
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
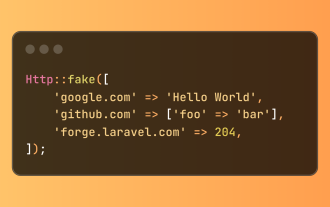
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
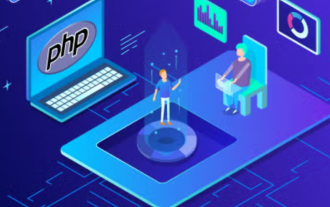
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
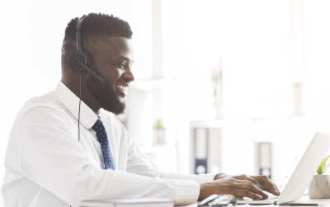
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
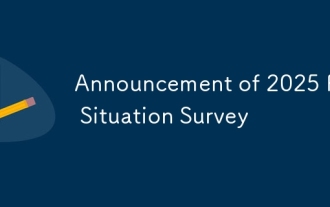
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
