How to realize image rotation using PHP and GD library
How to implement image rotation using PHP and GD libraries
Image rotation is a common image processing requirement. By rotating images, you can achieve some special effects or meet user needs. In PHP, you can use the GD library to implement the image rotation function. This article will introduce how to use PHP and the GD library to implement image rotation, with code examples.
First, make sure your PHP environment has the GD library extension installed. Enter php -m on the command line to check if there is a gd module. If not, you need to install it first.
The following is a simple code example to implement the image rotation function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
|
In the above code, the original image is first read through the imagecreatefromjpeg() function. If your image format is not JPEG, you need to use the corresponding function to read it.
Then, create a new canvas with the same size as the original image to store the rotated image. To ensure that the canvas can accommodate the rotated image, the width and height of the canvas need to be adjusted.
The next step is to implement image rotation. The imagerotate() function can be used to rotate the image according to the specified angle. Among them, the first parameter is the original image resource, the second parameter is the angle of rotation, and the third parameter is to set the background color (optional), here we set it to 0.
Finally, use the imagejpeg() function to save the rotated image to the specified path.
Finally, don’t forget to release resources and use the imagedestroy() function to destroy the original image and the rotated image.
This is just a simple example, you can modify and extend the code according to actual needs. For example, the rotation angle can be dynamically set through user input, or functions such as batch rotation of pictures can be implemented.
Summary:
This article introduces how to use PHP and GD library to achieve image rotation. By calling the functions provided by the GD library, we can easily implement the function of rotating images. I hope this article can help you when using PHP for image processing.
PHP and GD libraries are very powerful tools. Mastering them, you can implement various complex image processing operations. Not only limited to rotation, but also scaling, cropping, watermarking, and more. In actual projects, these image processing functions can bring a better user experience and enhance the attractiveness of the website.
The above is the detailed content of How to realize image rotation using PHP and GD library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


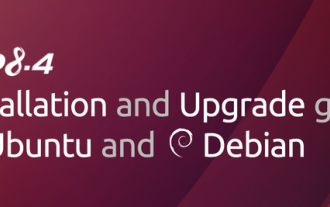
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
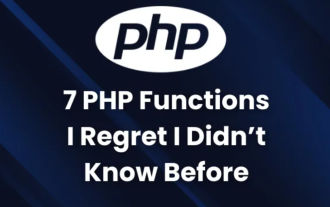
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
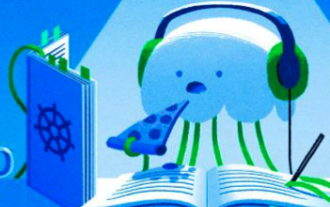
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
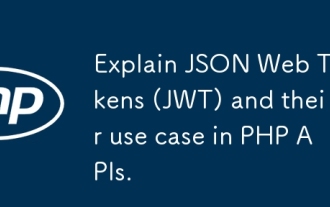
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
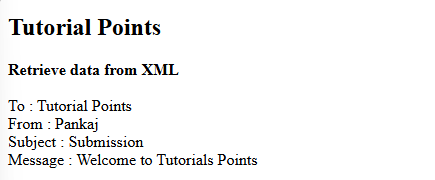
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
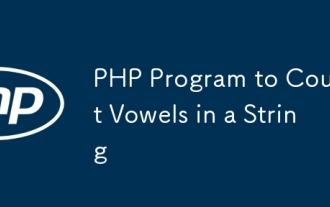
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
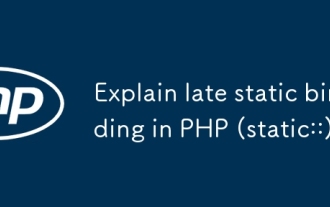
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
