


Practical use of regular expressions in Go language: How to match fixed-digit numeric verification codes
Go language regular expression practice: How to match fixed-digit numeric verification codes
Introduction:
In modern Internet applications, verification codes are a common security mechanism. In order to ensure the security of user data, many websites and applications will require users to enter a fixed-digit numeric verification code. In this article, we will use regular expressions in the Go language to implement the function of matching fixed-digit numeric verification codes.
Introduction to regular expressions:
Regular expression is a powerful pattern matching tool that can match specific patterns in strings by defining a set of rules. In Go language, we can use the built-in "regexp" package to operate regular expressions.
Implementation process:
First, we need to import the "regexp" package.
import ( "fmt" "regexp" )
Next, we can define a function called "MatchDigitCode", which receives two parameters: the verification code to be matched and the number of digits. The function is implemented as follows:
func MatchDigitCode(code string, digit int) bool { regex := fmt.Sprintf("^[0-9]{%d}$", digit) match := regexp.MustCompile(regex).MatchString(code) return match }
In the above code, we use the "Sprintf" function to construct a regular expression. Among them, "^" means matching the beginning of the string, "[0-9]" means matching numeric characters, "{%d}" means matching digit previous regular expressions, and "$" means matching the end of the string. Finally, use the "MatchString" function to determine whether the verification code matches.
Code Example:
The following is a complete example that demonstrates how to use regular expressions to match a fixed number of digits in a verification code.
package main import ( "fmt" "regexp" ) func MatchDigitCode(code string, digit int) bool { regex := fmt.Sprintf("^[0-9]{%d}$", digit) match := regexp.MustCompile(regex).MatchString(code) return match } func main() { code1 := "12345" code2 := "abcde" code3 := "67890" digit := 5 fmt.Printf("Code1: %s, Match: %v ", code1, MatchDigitCode(code1, digit)) fmt.Printf("Code2: %s, Match: %v ", code2, MatchDigitCode(code2, digit)) fmt.Printf("Code3: %s, Match: %v ", code3, MatchDigitCode(code3, digit)) }
In the above example, we defined three verification codes respectively, among which "code1" and "code3" are digital verification codes with 5 digits, and "code2" is not a 5-digit number. Verification code. By calling the "MatchDigitCode" function, we can determine that "code1" and "code3" are valid verification codes, while "code2" is an invalid verification code.
Summary:
This article introduces how to use regular expressions in Go language to match fixed-digit numeric verification codes. We used the functions in the "regexp" package to determine whether a string conforms to the specified regular expression rules. In this way, we can easily implement the verification function of the verification code.
The above is the detailed content of Practical use of regular expressions in Go language: How to match fixed-digit numeric verification codes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


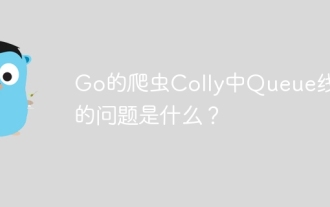
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
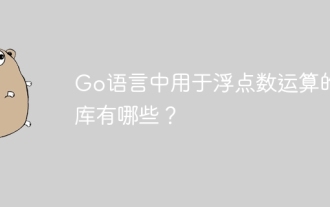
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
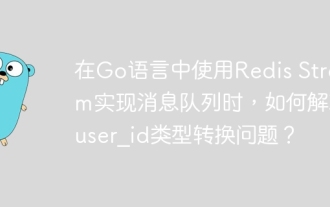
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
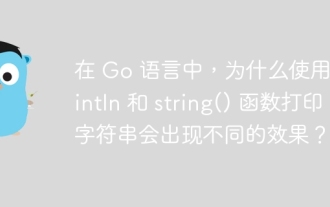
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
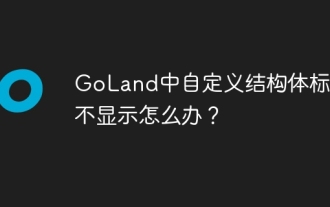
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
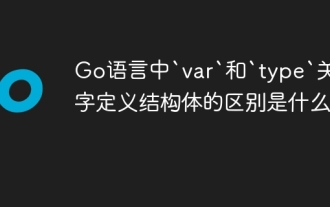
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
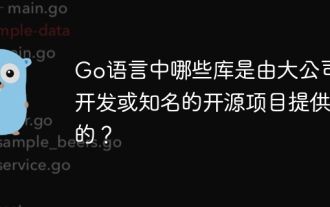
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
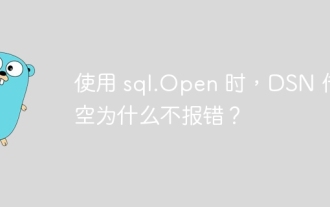
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
