


Go language regular expression skills: how to match consecutive numbers
Go language regular expression skills: How to match consecutive numbers
In the process of processing text, it is often necessary to use regular expressions for text matching and search and replacement operations. For some specific needs, such as matching consecutive numbers, we can use some techniques to achieve it.
In the Go language, by using the regular expression package regexp
, we can easily achieve this requirement. Two common ways of matching consecutive numbers will be introduced below, and sample codes will be given.
Method 1: The same numbers that appear continuously
For situations where we need to match the same numbers that appear continuously, we can use backreferences to achieve this. Backreferences can refer to content that has been matched previously. Specifically, they can be matched by using the form (d)
. Among them,
represents the content captured in the first bracket before the reference, and
represents repeated one or more times.
The sample code is as follows:
package main import ( "fmt" "regexp" ) func main() { text := "111222333444555" re := regexp.MustCompile(`(d)+`) matches := re.FindAllString(text, -1) for _, match := range matches { fmt.Println(match) } }
The running result is:
111 222 333 444 555
Method 2: Any number that appears continuously
If you want to match any number that appears continuously , we can use the expression d
to achieve this. Among them, d
means matching any numeric character, and
means repeating one or more times.
The sample code is as follows:
package main import ( "fmt" "regexp" ) func main() { text := "123456789" re := regexp.MustCompile(`d+`) matches := re.FindAllString(text, -1) for _, match := range matches { fmt.Println(match) } }
The running result is:
123456789
Through the above two methods, we can easily achieve the need to match consecutive numbers. Of course, in practical applications, we can also adjust the regular expression pattern according to specific situations to meet more complex matching needs.
I hope this article will help you understand how to match consecutive numbers in Go language!
The above is the detailed content of Go language regular expression skills: how to match consecutive numbers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
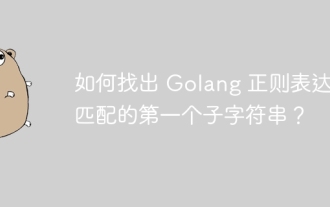
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].

How to use Go or Rust to call Python scripts to achieve true parallel execution? Recently I've been using Python...
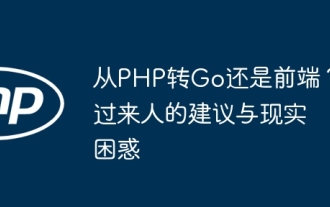
Confusion and the cause of choosing from PHP to Go Recently, I accidentally learned about the salary of colleagues in other positions such as Android and Embedded C in the company, and found that they are more...
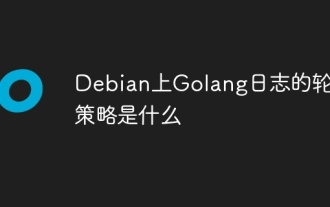
In Debian systems, Go's log rotation usually relies on third-party libraries, rather than the features that come with Go standard libraries. lumberjack is a commonly used option. It can be used with various log frameworks (such as zap and logrus) to realize automatic rotation and compression of log files. Here is a sample configuration using the lumberjack and zap libraries: packagemainimport("gopkg.in/natefinch/lumberjack.v2""go.uber.org/zap""go.uber.org/zap/zapcor
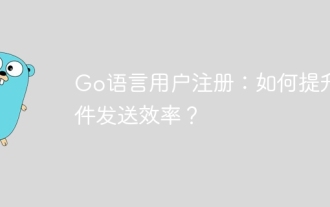
Optimization of the efficiency of email sending in the Go language registration function. In the process of learning Go language backend development, when implementing the user registration function, it is often necessary to send a urge...
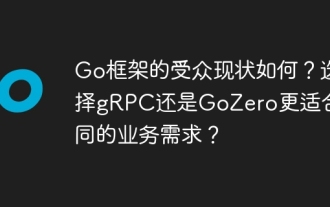
Analysis of the audience status of Go framework In the current Go programming ecosystem, developers often face choosing the right framework to meet their business needs. Today we...
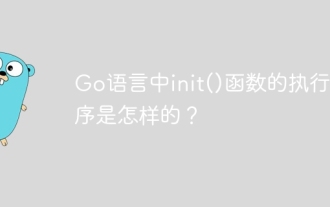
The execution order of the init() function in Go language In Go programming, the init() function is a special function, which is used to execute some necessary functions when package initialization...
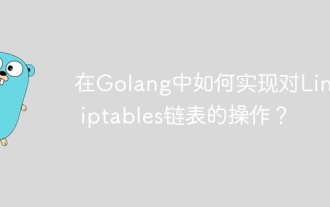
Using Golang to implement Linux...
