


How to extract HTML tag content using regular expressions in Go language
How to use regular expressions to extract HTML tag content in Go language
Introduction:
Regular expression is a powerful text matching tool, and it is also widely used in Go language. In the scenario of processing HTML tags, regular expressions can help us quickly extract the content we need. This article will introduce how to use regular expressions to extract the content of HTML tags in Go language, and give relevant code examples.
1. Introduce related packages
First, we need to import related packages: regexp and fmt. The regexp package provides support for regular expressions, and the fmt package is used for formatted output.
import ( "fmt" "regexp" )
2. Prepare HTML string
Next, we need to prepare a string containing HTML tags as a test sample. For example, we have an HTML string containing the
tag:
htmlStr := "<p>这是一个示例</p>"
3. Writing regular expressions
Before using regular expressions to extract the content of HTML tags, you need to write the corresponding regular expressions. Mode. Suppose we wish to extract the content between
tags, our regular expression could be <p>(.*?)</p>
. Among them, .*?
means matching any character, and ()
means a group to extract the matched content.
4. Use regular expressions to extract content
Using the related functions provided by the regexp package, we can easily use regular expressions to extract HTML tag content.
// 编译正则表达式 pattern, _ := regexp.Compile(`<p>(.*?)</p>`) // 提取内容 result := pattern.FindStringSubmatch(htmlStr) // 输出结果 fmt.Println(result[1])
In the above code, we first use the regexp.Compile
function to compile the regular expression we wrote before<p>(.*?)< /p>
.
Then, we use the pattern.FindStringSubmatch
function, taking the HTML string as a parameter to extract the content. This function will return a string array, where the first element is the complete matching string, and the following elements are the matching results of each group.
Finally, we output the results to the console through the fmt.Println
function.
5. Complete sample code
package main import ( "fmt" "regexp" ) func main() { // 准备HTML字符串 htmlStr := "<p>这是一个示例</p>" // 编译正则表达式 pattern, _ := regexp.Compile(`<p>(.*?)</p>`) // 提取内容 result := pattern.FindStringSubmatch(htmlStr) // 输出结果 fmt.Println(result[1]) }
Run the above code, we will get the output: This is an example
, this is what we successfully extracted from the HTML tag Content.
6. Notes
When using regular expressions to extract the content of HTML tags, there are several things to pay attention to:
- Need to write regular expressions correctly: Regular expressions Writing expressions is a complex process, and appropriate expressions need to be written according to specific needs. You can verify the accuracy of regular expressions using an online regular expression testing tool.
- Need to use grouping correctly: By using parentheses, we can define grouping in regular expressions. The grouped content can be accessed through the returned array.
- You need to pay attention to the format of the HTML string: When using regular expressions to extract the content of HTML tags, you need to ensure that the format of the HTML string complies with the specification. If the HTML string is not properly formatted, it may cause the match to fail.
To sum up, this article introduces how to use regular expressions to extract HTML tag content in Go language, and gives relevant sample code. I hope this article can help readers better understand and use regular expressions in Go language.
The above is the detailed content of How to extract HTML tag content using regular expressions in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


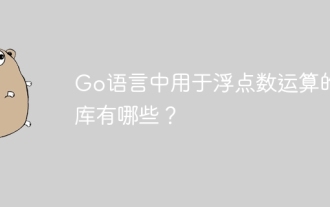
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
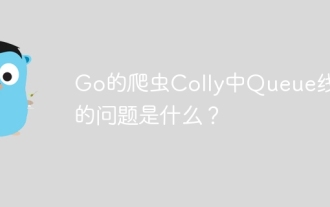
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
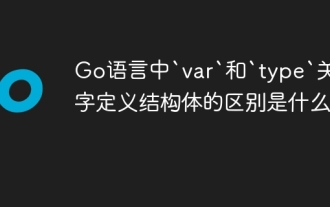
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
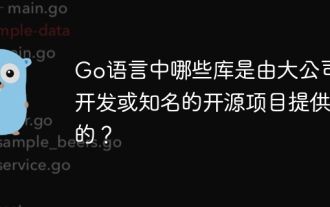
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
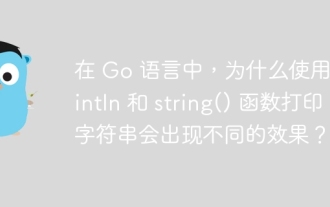
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
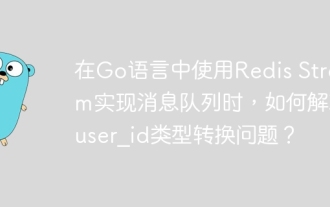
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
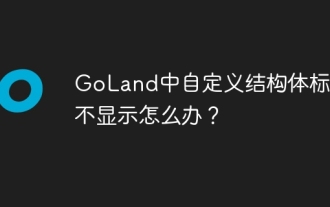
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...

AnexampleofastartingtaginHTMLis,whichbeginsaparagraph.StartingtagsareessentialinHTMLastheyinitiateelements,definetheirtypes,andarecrucialforstructuringwebpagesandconstructingtheDOM.
