Tutorial on making video puzzles with HTML5_html5 tutorial tips
A few days ago, my colleague showed me a special effect, which is a jigsaw puzzle. The difference is that the puzzle is animation. He asked me to make a DEMO, so I worked on it myself for a while, and it was indeed not difficult. It's easy to do with canvas. So this blog post is not suitable for experts. . . . I just wrote it casually for fun.
Rendering:
At least when I first saw this, I thought it was quite novel, so I thought of making it for fun. If you think the poster is out, please give me a thumbs up
Without further ado, let’s start with DEMO: Video Puzzle (You may have to wait a while to see the effect. I made a video link directly from w3school. You can drag and drop it. It's very simple, and may still have some bugs. After all, it's just a DEMO for fun. Just explain the principles.) Another point is that directly drawing the current frame of the video into the canvas does not seem to be supported on mobile devices. . . At least I looked at it on my iPad and found that I couldn’t draw. If anyone knows how to solve this problem, please answer it for me. I’d be very grateful
Principle: Each puzzle piece is a canvas, and an off-screen canvas is also required. First create a video tag
And hide the video, and then draw each frame into the off-screen canvas when playing the video (off-screen canvas is the hidden canvas, used to save data). The writing method is very simple:
, just use the drawImage method to draw it directly. Why use an off-screen canvas first? Because if you directly draw each frame of data into the canvas of all puzzle pieces at the same time, the browser will crash instantly. So use an off-screen canvas as a buffer. First save the data of the current frame to the canvas, and then draw the canvas into the canvas as the puzzle piece. Drawing canvas into canvas is also very simple, you can also use drawImage:
ctx2.drawImage(cs , -this.cols*this.w , -this.rows*this.h , vw , vh);
Then. . . . The principle is as simple as that. I would like to remind you later that when using requestAnimationFrame to loop through frames, you need to limit the speed. For example, as written below, I fetch every 30 milliseconds. It is recommended to take 30~50 milliseconds. If it is too low, the browser will crash easily. If it is too high, it will cause the browser to crash. If the video is stuck, the video will freeze:
function animate(){
var newTime = new Date();
if(newTime - lastTime > 30){
lastTime = newTime;
ctx.drawImage(video , 0 , 0 , vw , vh);
canvases. forEach(function(){
var ctx2 = this.cas.getContext('2d');
ctx2.drawImage(cs , -this.cols*this.w , -this.rows*this.h , vw , vh);
});
}
if("requestAnimationFrame" in window){
requestAnimationFrame(animate);
}
else if("webkitRequestAnimationFrame" in window ){
webkitRequestAnimationFrame(animate);
}
else if("msRequestAnimationFrame" in window){
msRequestAnimationFrame(animate);
}
else if("mozRequestAnimationFrame" in window ){
mozRequestAnimationFrame(animate);
}
}
Finally post all the code:
<script><br> var video = document.getElementById("video");<br> var cs = document.getElementById("liping");<br> var ctx = cs.getContext('2d')<br> var rows = 3,<br> cols = 3,<br> cb = document.querySelector(".allCanvas"),<br> vw = 600,<br> vh = 400,<br> canvases = [];</p> <p> function createCanvas(){<br> var num = rows*cols;<br> for(var i=0;i<cols;i ){<br> for(var j=0;j<rows;j ){<br> var canvas = new vCanvas(Math.random()*600, Math.random()*600 , vw/rows , vh/cols , j , i);<br> canvases.push(canvas);<br> }<br> }<br> }</p> <p> var vCanvas = function(x,y,w,h,cols,rows){<br> this.x = x;<br> this.y = y;<br> this.w = w;<br> this.h = h;<br> this.cols = cols;<br> this.rows = rows;<br> this.creat();<br> this.behavior();<br> }<br> vCanvas.prototype = {<br> creat:function(){<br> this.cas = document.createElement("canvas");<br> cb.appendChild(this.cas);<br> this.cas.className = "vcanvas";<br> this.cas.id = "vc_" (this.cols 1)*(this.rows 1);<br> this.cas.style.left = this.x "px";<br> this.cas.style.top = this.y "px";<br> this.cas.width = this.w;<br> this.cas.height = this.h;<br> },<br> behavior:function(){<br> this.cas.onmousedown = function(e){<br> e = e || window.event;<br> var that = this;<br> var om = {<br> x:e.clientX,<br> y:e.clientY<br> }<br> window.onmousemove = function(e){<br> e = e || window.event;<br> var nm = {<br> x:e.clientX,<br> y:e.clientY<br> }<br> that.style.left = parseInt(that.style.left.replace("px","")) (nm.x-om.x) "px";<br> that.style.top = parseInt(that.style.top.replace("px","")) (nm.y-om.y) "px";<br> om = nm;<br> }<br> window.onmouseup = function(){<br> this.onmousemove = null;<br> }<br> }<br> }<br> }</p> <p> Array.prototype.forEach = function(callback){<br> for(var i=0;i<this.length;i ){<br> callback.call(this[i]);<br> }<br> }</p> <p> var lastTime = 0;<br> function initAnimate(){<br> lastTime = new Date();<br> createCanvas();<br> animate();<br> }</p> <p> function animate(){<br> var newTime = new Date();<br> if(newTime - lastTime > 30){<br> lastTime = newTime;<br> ctx.drawImage(video , 0 , 0 , vw , vh);<br> canvases.forEach(function(){<br> var ctx2 = this.cas.getContext('2d');<br> ctx2.drawImage(cs , -this.cols*this.w , -this.rows*this.h , vw , vh);<br> });<br> }<br> if("requestAnimationFrame" in window){<br> requestAnimationFrame(animate);<br> }<br> else if("webkitRequestAnimationFrame" in window){<br> webkitRequestAnimationFrame(animate);<br> }<br> else if("msRequestAnimationFrame" in window){<br> msRequestAnimationFrame(animate);<br> }<br> else if("mozRequestAnimationFrame" in window){<br> mozRequestAnimationFrame(animate);<br> }<br> }</p> <p> video.play();<br> initAnimate();<br> </script>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


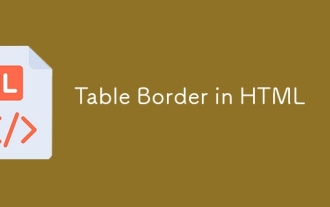
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
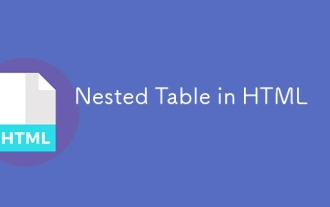
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
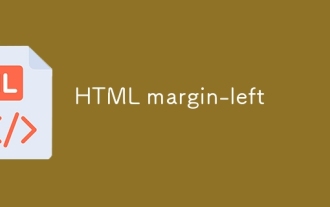
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
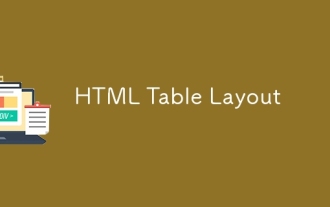
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
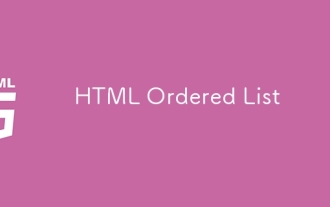
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
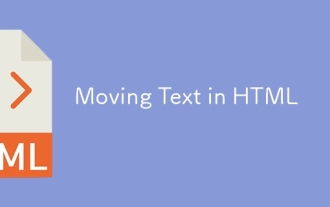
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
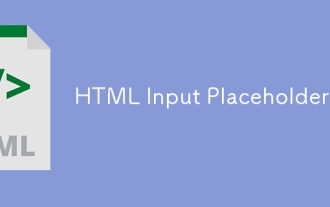
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
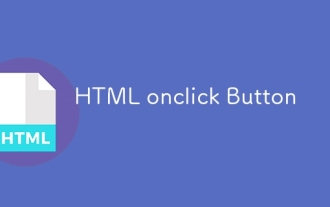
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
