


Interactive methods and techniques for PHP arrays and databases
Interaction methods and techniques between PHP arrays and databases
Introduction:
In PHP development, operating arrays is a basic skill, and interaction with databases is a common requirement. This article will introduce how to use PHP to manipulate arrays and interact with databases, and provide some practical code examples.
1. Basic operations of PHP arrays
In PHP, arrays are a very useful data type that can be used to store and operate a set of related data. The following are some common examples of array operations:
- Create an array:
$arr = array("apple", "banana", "orange"); - Access the array Element:
echo $arr[0]; // Output: apple - Add element to array:
$arr[] = "grape"; // Add element to the end of array
array_push($arr, "pineapple"); //Similarly, add elements to the end of the array - Delete array elements:
unset($arr[1]); //Delete the element at the specified index
array_pop($arr); // Delete the element at the end of the array -
Traverse the array:
foreach($arr as $key => $value) {echo "索引:".$key.",值:".$value;
Copy after login}
2. Interaction with the database
Interaction with the database is a common task in Web development. The following will introduce how to use PHP to perform basic database operations, with code examples.
Connect to the database:
$servername = "localhost";
$username = "root";
$password = "password ";
$dbname = "myDB";$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn-> connect_error) {
die("数据库连接失败: " . $conn->connect_error);
Copy after login}
?>Query data:
$sql = "SELECT id, name , email FROM users";
$result = $conn->query($sql);if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) { echo "ID: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. "<br>"; }
Copy after login} else {
echo "没有找到数据!";
Copy after login}
?>Insert data:
$sql = "INSERT INTO users (name , email) VALUES ('John Doe', 'john@example.com')";if ($conn->query($sql) === TRUE) {
echo "新记录插入成功!";
Copy after login} else {
echo "插入数据时出错: " . $conn->error;
Copy after login}
?>Update data:
$sql = "UPDATE users SET email='john.doe@example.com' WHERE id=1";if ($conn->query($sql) === TRUE) {
echo "数据更新成功!";
Copy after login} else {
echo "更新数据时出错: " . $conn->error;
Copy after login}
?>Delete data:
$sql = "DELETE FROM users WHERE id= 1";if ($conn->query($sql) === TRUE) {
echo "数据删除成功!";
Copy after login} else {
echo "删除数据时出错: " . $conn->error;
Copy after login}
?> ;
3. Conclusion
This article introduces the basic methods of operating arrays in PHP development, and provides some sample codes for interacting with the database. Mastering these methods and techniques will be of great help to PHP development and interaction with databases. I hope this article can inspire and help readers.
The above is the detailed content of Interactive methods and techniques for PHP arrays and databases. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
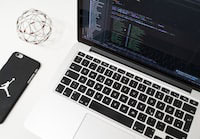
PDOPDO is an object-oriented database access abstraction layer that provides a unified interface for PHP, allowing you to use the same code to interact with different databases (such as Mysql, postgresql, oracle). PDO hides the complexity of underlying database connections and simplifies database operations. Advantages and Disadvantages Advantages: Unified interface, supports multiple databases, simplifies database operations, reduces development difficulty, provides prepared statements, improves security, supports transaction processing Disadvantages: performance may be slightly lower than native extensions, relies on external libraries, may increase overhead, demo code uses PDO Connect to mysql database: $db=newPDO("mysql:host=localhost;dbnam
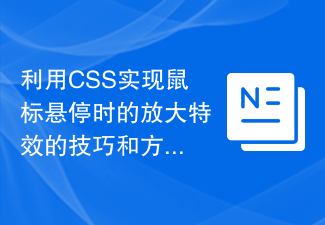
Tips and methods to use CSS to implement the magnification effect when the mouse is hovering The magnification effect when the mouse is hovering is a common web page animation that can add interactivity and attraction to the web page. This article will introduce some techniques and methods to achieve this special effect, and provide specific CSS code examples. Use the transform attribute of CSS to achieve transformation effects such as scaling, rotation, tilt, and translation of elements. We can use the scale() function to achieve the magnification effect when the mouse is hovering. head
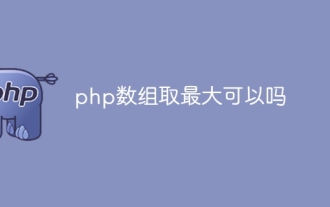
There are three ways to get the maximum value of a PHP array: 1. The "max()" function is used to get the maximum value in the array; 2. The "rsort()" function is used to sort the array in descending order and return the sort. the array after. You can get the maximum value by accessing the element with index 0; 3. Use loop traversal to compare the value of each element to find the maximum value.
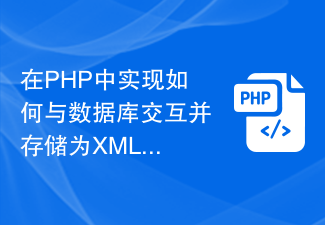
How to interact with the database and store it in XML format in PHP In web applications, interacting with the database is a very common and important operation. Storing the data obtained from the database in XML format is a convenient way to exchange data between different platforms and different languages. This article will introduce how to interact with the database in PHP and store the data in XML format. First, we need to establish a database connection. In PHP, this can be achieved using mysqli or PDO extensions
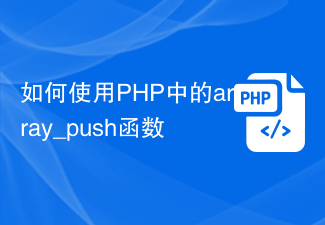
In PHP development, array is a very common data structure. In actual development, we often need to add new elements to the array. For this purpose, PHP provides a very convenient function array_push. The array_push function is used to add one or more elements to the end of an array. The syntax of this function is as follows: array_push(array, value1, value2,...) where array is the array to which elements need to be added, value1, valu
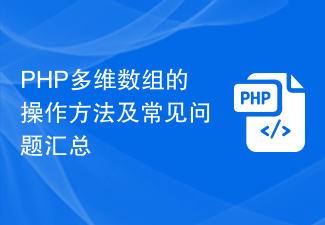
PHP is a programming language widely used in web development. It has a rich set of tools and function libraries, among which multidimensional arrays are one of its important data structures. Multidimensional arrays allow us to organize data into tables or matrices for easy data processing and manipulation. This article will introduce the basic operation methods and common problems of multi-dimensional arrays in PHP, and provide practical examples to illustrate. 1. Basic operation methods 1. Define multi-dimensional arrays We can define a multi-dimensional array through a variety of methods. Here are some common methods: $myArray
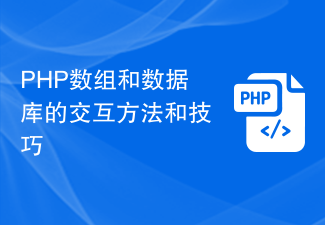
Interaction methods and techniques for PHP arrays and databases Introduction: In PHP development, operating arrays is a basic skill, and interaction with databases is a common requirement. This article will introduce how to use PHP to manipulate arrays and interact with databases, and provide some practical code examples. 1. Basic operations of PHP arrays In PHP, arrays are a very useful data type that can be used to store and operate a group of related data. Here are some common examples of array operations: Create an array: $arr=array
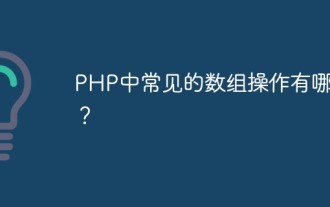
PHP is a widely used server-side programming language and a very important part of Internet application development. In PHP, array is a very common data type that can be used to store and manipulate a group of related data. In this article, we will introduce common array operations in PHP, hoping to be helpful to PHP developers. Creating an array In PHP, creating an array is very simple. You can use the array() function or the [] symbol to create it. For example, the following code will create an array with three elements:
