


How to use abstract classes and interfaces to manage and operate custom data types in PHP
How to use abstract classes and interfaces to manage and operate custom data types in PHP
Introduction:
In PHP development, we often need to manage and operate various types of data. In order to better organize and process this data, we can use abstract classes and interfaces to define our own data types and implement corresponding behaviors. This article will introduce how to use abstract classes and interfaces to manage and operate custom data types, and provide some code examples to help understanding.
1. The use of abstract classes
An abstract class is a class that cannot be instantiated. It is only used to define public properties and methods. By inheriting an abstract class, we can create subclasses with similar behavior and implement their own special logic. The following is an example of using abstract classes to manage different shapes:
abstract class Shape { protected $name; public function __construct($name) { $this->name = $name; } abstract public function area(); public function displayName() { echo "This shape is a " . $this->name . ". "; } } class Circle extends Shape { private $radius; public function __construct($name, $radius) { parent::__construct($name); $this->radius = $radius; } public function area() { return 3.14 * $this->radius * $this->radius; } } class Triangle extends Shape { private $base; private $height; public function __construct($name, $base, $height) { parent::__construct($name); $this->base = $base; $this->height = $height; } public function area() { return 0.5 * $this->base * $this->height; } } $circle = new Circle("circle", 5); $triangle = new Triangle("triangle", 4, 6); $circle->displayName(); echo "Area of circle: " . $circle->area() . " "; $triangle->displayName(); echo "Area of triangle: " . $triangle->area() . " ";
In the above code, we define an abstract class Shape, which has a public attribute name and an abstract method area(). Circle and Triangle are subclasses of Shape, and they respectively implement the area() method to calculate the areas of different shapes. We can output the name of the shape by calling the displayName() method, and calculate and output the area by calling the area() method.
2. The use of interfaces
An interface is a structure used to define a set of public methods of a class. By implementing interfaces, we can force a class to implement corresponding methods and ensure that these methods have uniform behavior in different classes. The following is an example of using interfaces to manage different products:
interface ProductInterface { public function getName(); public function getPrice(); } class Product implements ProductInterface { private $name; private $price; public function __construct($name, $price) { $this->name = $name; $this->price = $price; } public function getName() { return $this->name; } public function getPrice() { return $this->price; } } class Book implements ProductInterface { private $name; private $price; private $author; public function __construct($name, $price, $author) { $this->name = $name; $this->price = $price; $this->author = $author; } public function getName() { return $this->name; } public function getPrice() { return $this->price; } public function getAuthor() { return $this->author; } } $product = new Product("generic product", 10); $book = new Book("PHP basics", 20, "John Doe"); echo "Product: " . $product->getName() . ", Price: " . $product->getPrice() . " "; echo "Book: " . $book->getName() . ", Price: " . $book->getPrice() . ", Author: " . $book->getAuthor() . " ";
In the above code, we define an interface ProductInterface, which has two public methods getName() and getPrice(). Product and Book are classes that implement the ProductInterface interface. They implement the interface methods respectively to obtain the name and price of the product. By creating instances of Product and Book, we can call the corresponding methods to obtain information about the corresponding products.
Conclusion:
By using abstract classes and interfaces, we can better manage and operate custom data types. Abstract classes help us define public properties and methods and encapsulate similar behaviors into subclasses. Interfaces help us define the structure of a set of public methods and ensure that classes have the same behavior when implementing the interface. In PHP development, being good at using abstract classes and interfaces can improve the readability and maintainability of the code, making it easier for us to process various types of data.
The above is the detailed content of How to use abstract classes and interfaces to manage and operate custom data types in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
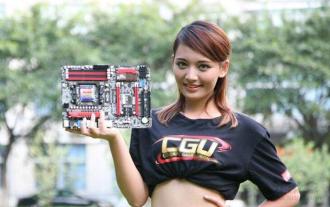
When we assemble the computer, although the installation process is simple, we often encounter problems in the wiring. Often, users mistakenly plug the power supply line of the CPU radiator into the SYS_FAN. Although the fan can rotate, it may not work when the computer is turned on. There will be an F1 error "CPUFanError", which also causes the CPU cooler to be unable to adjust the speed intelligently. Let's share the common knowledge about the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard. Popular science on the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard 1. CPU_FANCPU_FAN is a dedicated interface for the CPU radiator and works at 12V
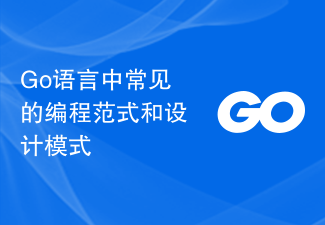
As a modern and efficient programming language, Go language has rich programming paradigms and design patterns that can help developers write high-quality, maintainable code. This article will introduce common programming paradigms and design patterns in the Go language and provide specific code examples. 1. Object-oriented programming In the Go language, you can use structures and methods to implement object-oriented programming. By defining a structure and binding methods to the structure, the object-oriented features of data encapsulation and behavior binding can be achieved. packagemaini
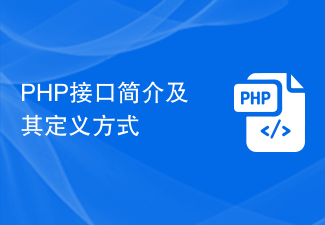
Introduction to PHP interface and how it is defined. PHP is an open source scripting language widely used in Web development. It is flexible, simple, and powerful. In PHP, an interface is a tool that defines common methods between multiple classes, achieving polymorphism and making code more flexible and reusable. This article will introduce the concept of PHP interfaces and how to define them, and provide specific code examples to demonstrate their usage. 1. PHP interface concept Interface plays an important role in object-oriented programming, defining the class application
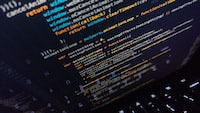
The reason for the error is in python. The reason why NotImplementedError() is thrown in Tornado may be because an abstract method or interface is not implemented. These methods or interfaces are declared in the parent class but not implemented in the child class. Subclasses need to implement these methods or interfaces to work properly. How to solve this problem is to implement the abstract method or interface declared by the parent class in the child class. If you are using a class to inherit from another class and you see this error, you should implement all the abstract methods declared in the parent class in the child class. If you are using an interface and you see this error, you should implement all methods declared in the interface in the class that implements the interface. If you are not sure which
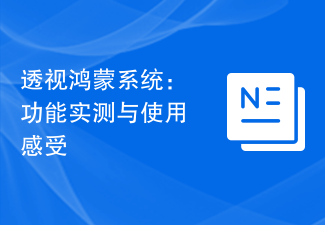
As a new operating system launched by Huawei, Hongmeng system has caused quite a stir in the industry. As a new attempt by Huawei after the US ban, Hongmeng system has high hopes and expectations. Recently, I was fortunate enough to get a Huawei mobile phone equipped with Hongmeng system. After a period of use and actual testing, I will share some functional testing and usage experience of Hongmeng system. First, let’s take a look at the interface and functions of Hongmeng system. The Hongmeng system adopts Huawei's own design style as a whole, which is simple, clear and smooth in operation. On the desktop, various

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
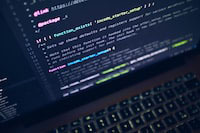
Interface Interface defines abstract methods and constants in Java. The methods in the interface are not implemented, but are provided by the class that implements the interface. The interface defines a contract that requires the implementation class to provide specified method implementations. Declare the interface: publicinterfaceExampleInterface{voiddoSomething();intgetSomething();} Abstract class An abstract class is a class that cannot be instantiated. It contains a mixture of abstract and non-abstract methods. Similar to interfaces, abstract methods in abstract classes are implemented by subclasses. However, abstract classes can also contain concrete methods, which provide default implementations. Declare abstract class: publicabstractcl
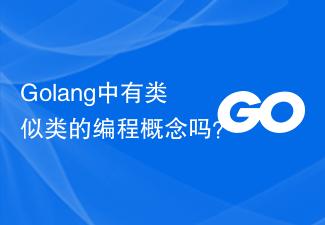
In Golang (Go language), although there is no concept similar to classes in traditional object-oriented programming languages, similar functions can be achieved through structures and methods. In Golang, we can define a structure to encapsulate data, and define methods for the structure to manipulate data. This method can realize the basic functions of classes in object-oriented programming. First, let's look at a simple example, defining a structure named Person, containing two fields: name and age, and the Person structure
