How to use provide and inject for component communication in Vue?
How to use provide and inject for component communication in Vue?
In Vue, communication between components is a very important issue. Vue provides a variety of methods to pass data and communicate between components. In some specific scenarios, using provide and inject is a very convenient and efficient way to achieve communication between components.
provide and inject are a pair of matching options that allow ancestor components to inject a dependency into all descendant components without manually passing it layer by layer. They can form a "dependency injection tree" between ancestors and descendants.
Components that provide dependencies use the provide option, and components that receive dependencies use the inject option.
Let's look at an example below. Suppose there is a parent component A and two child components B and C. We need to pass the data in the parent component A to the child components B and C.
The code of parent component A is as follows:
<template> <div> <child-b></child-b> <child-c></child-c> </div> </template> <script> import ChildB from './ChildB.vue' import ChildC from './ChildC.vue' export default { components: { ChildB, ChildC }, provide() { return { dataFromA: this.dataFromA } }, data() { return { dataFromA: 'Hello' } } } </script>
Child component B can receive the data provided by parent component A through the inject option. The code is as follows:
<template> <div> <p>Child B</p> <p>Data from A: {{ dataFromA }}</p> </div> </template> <script> export default { inject: ['dataFromA'] } </script>
Similarly, the child component Component C can also receive the data provided by parent component A through the inject option. The code is as follows:
<template> <div> <p>Child C</p> <p>Data from A: {{ dataFromA }}</p> </div> </template> <script> export default { inject: ['dataFromA'] } </script>
In this way, the data "dataFromA" provided by parent component A will be automatically injected into child components B and C. Components B and C can use this data directly.
It should be noted that provide and inject can only be used for communication between ancestor components and descendant components, not for communication between sibling components.
The above is a simple example of using provide and inject for component communication. Using provide and inject can effectively reduce the complexity of transferring data between components and improve the maintainability and reusability of the code. In some specific scenarios, using provide and inject is a very convenient and efficient way of component communication.
The above is the detailed content of How to use provide and inject for component communication in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


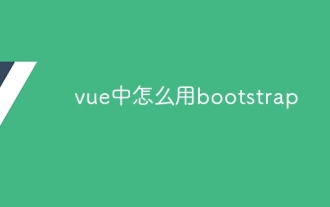
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
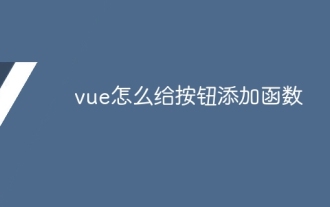
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
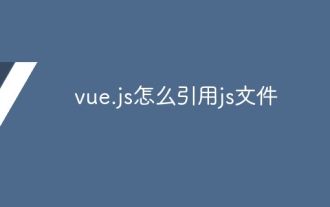
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
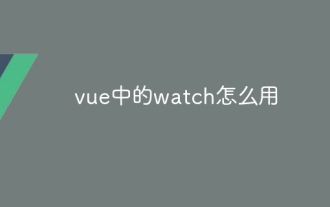
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
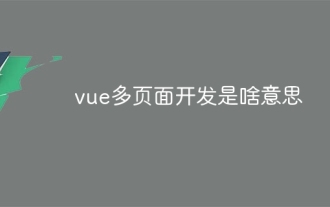
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
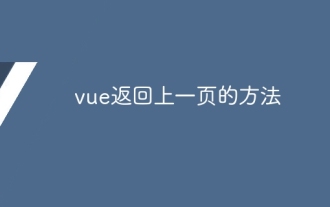
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
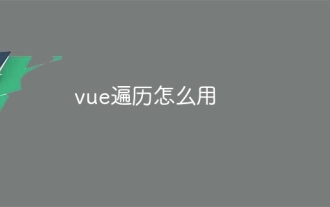
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
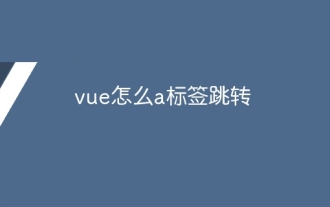
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
