


Tips for PHP developers: The clever combination of Alibaba Cloud OCR and text processing
Tips for PHP developers: The clever combination of Alibaba Cloud OCR and text processing
With the advent of the digital age, more and more companies and individuals have begun to pay attention to text processing and analysis. In PHP development, how to use existing technologies and services to achieve efficient OCR (Optical Character Recognition, optical character recognition) and text processing functions has become an urgent problem for developers to solve.
As a widely used scripting language, PHP has a wealth of third-party libraries and services to choose from. This article will focus on how to cleverly use Alibaba Cloud OCR service and text processing functions, and provide some tips for PHP developers.
1. Preparation
- Register an Alibaba Cloud account and obtain AccessKeyId and AccessKeySecret. These two parameters will be used to call the Alibaba Cloud API interface.
- Install aliyun-sdk-php library. This is the PHP SDK officially provided by Alibaba Cloud, which is used to conveniently make service calls. It can be installed through composer, or downloaded from github and introduced manually.
2. Use Alibaba Cloud OCR to realize image text recognition
Alibaba Cloud OCR service provides a fast and accurate way to extract text from images. The following is a basic sample code:
require_once '/path/to/aliyun-sdk-php/autoload.php'; use AlibabaCloudClientAlibabaCloud; use AlibabaCloudClientExceptionClientException; use AlibabaCloudClientExceptionServerException; use AlibabaCloudOcrOcr; use AlibabaCloudOcrOcrGeneralBasic; AlibabaCloud::accessKeyClient('YOUR_ACCESS_KEY_ID', 'YOUR_ACCESS_KEY_SECRET') ->regionId('cn-hangzhou') ->asDefaultClient(); try { $res = Ocr::v20191230()->generalBasic() ->jsonBody([ 'ImageURL' => 'https://example.com/image.jpg' ]) ->request(); print_r($res->getData()); } catch (ClientException $e) { echo $e->getErrorMessage() . PHP_EOL; } catch (ServerException $e) { echo $e->getErrorMessage() . PHP_EOL; }
In the above example, the required classes are first introduced as required. Then, use accessKey and accessKeySecret to initialize the aliyun-sdk-php client. Finally, call the generalBasic
interface of Alibaba Cloud OCR, pass in the URL of the image to be processed, and obtain the text recognition results.
3. Use text processing API to extract key information
Alibaba Cloud text processing service provides a series of functions, such as keyword extraction, entity recognition, sentiment analysis, etc., which can help developers more Process and analyze written information effectively. The following is a simple sample code:
require_once '/path/to/aliyun-sdk-php/autoload.php'; use AlibabaCloudClientAlibabaCloud; use AlibabaCloudClientExceptionClientException; use AlibabaCloudClientExceptionServerException; use AlibabaCloudNlpNlp; AlibabaCloud::accessKeyClient('YOUR_ACCESS_KEY_ID', 'YOUR_ACCESS_KEY_SECRET') ->regionId('cn-hangzhou') ->asDefaultClient(); try { $res = Nlp::v20180408()->namedEntityRecognition()->jsonBody([ 'Text' => '这是一段待处理的文本。', 'Language' => 'ZH' ])->request(); print_r($res->getData()); } catch (ClientException $e) { echo $e->getErrorMessage() . PHP_EOL; } catch (ServerException $e) { echo $e->getErrorMessage() . PHP_EOL; }
In the above example, the required classes are first introduced as required. Then, use accessKey and accessKeySecret to initialize the aliyun-sdk-php client. Finally, call the namedEntityRecognition
interface of Alibaba Cloud Text Processing, pass in the text and language type to be processed, and obtain the named entity recognition results.
4. Example of combining Alibaba Cloud OCR and text processing services
The following is a sample code that combines Alibaba Cloud OCR and text processing services:
require_once '/path/to/aliyun-sdk-php/autoload.php'; use AlibabaCloudClientAlibabaCloud; use AlibabaCloudClientExceptionClientException; use AlibabaCloudClientExceptionServerException; use AlibabaCloudOcrOcr; use AlibabaCloudNlpNlp; AlibabaCloud::accessKeyClient('YOUR_ACCESS_KEY_ID', 'YOUR_ACCESS_KEY_SECRET') ->regionId('cn-hangzhou') ->asDefaultClient(); try { $ocrRes = Ocr::v20191230()->generalBasic()->jsonBody([ 'ImageURL' => 'https://example.com/image.jpg' ])->request(); $text = ''; foreach ($ocrRes->getData()['Data']['OCRTexts']['OCRText'] as $ocrText) { $text .= $ocrText['Text'] . ' '; } $nlpRes = Nlp::v20180408()->keywordExtraction()->jsonBody([ 'Text' => $text, 'Language' => 'ZH' ])->request(); print_r($nlpRes->getData()); } catch (ClientException $e) { echo $e->getErrorMessage() . PHP_EOL; } catch (ServerException $e) { echo $e->getErrorMessage() . PHP_EOL; }
In the above In the example, the Alibaba Cloud OCR service is first called to extract the text from the image. Then, the recognized text is passed to Alibaba Cloud text processing service for keyword extraction. Finally, the keyword extraction results are output.
Summary:
This article introduces how to use Alibaba Cloud OCR and text processing services to achieve efficient image text recognition and text processing functions. By combining these two services, PHP developers can more easily process and analyze large amounts of text information, providing more convenient solutions for businesses and individuals. I believe these tips will be helpful to PHP developers.
The above is the detailed content of Tips for PHP developers: The clever combination of Alibaba Cloud OCR and text processing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


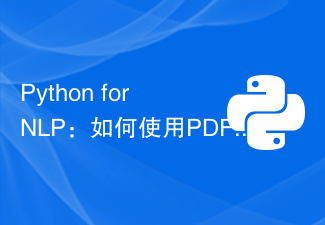
PythonforNLP: How to process text in PDF files using PDFMiner library? Introduction: PDF (Portable Document Format) is a format used to store documents, usually used for sharing and distributing electronic documents. In the field of natural language processing (NLP), we often need to extract text from PDF files for text analysis and processing. Python provides many libraries for processing PDF files, among which PDFMiner is a powerful
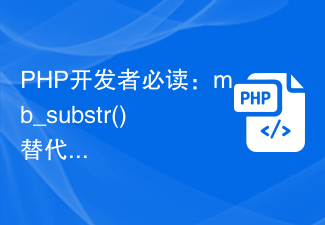
In PHP development, string interception is often used. In past development, we often used the mb_substr() function to intercept multi-byte characters. However, with the update of PHP versions and the development of technology, better alternatives have emerged that can handle the interception of multi-byte characters more efficiently. This article will introduce alternatives to the mb_substr() function and give specific code examples. Why you need to replace the mb_substr() function in earlier versions of PHP, m
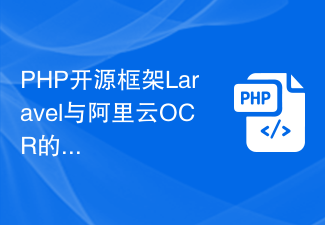
Introduction to the practical guide for the integration of the PHP open source framework Laravel and Alibaba Cloud OCR: With the development of the Internet, online image recognition has attracted more and more attention. Alibaba Cloud OCR (Optical Character Recognition, optical character recognition), as one of the leading OCR service providers in the market, provides powerful image recognition capabilities. As a popular PHP open source framework, Laravel provides a simple and efficient development method and is loved by the majority of developers.
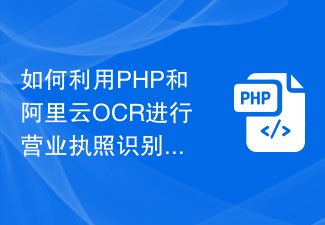
How to use PHP and Alibaba Cloud OCR for business license identification? Introduction: In today's digital era, rapid acquisition and processing of information are crucial to the survival and development of enterprises. The business license is the identity document of the enterprise and an important document for commercial activities. In order to better obtain and utilize the information on the business license, we can use Alibaba Cloud OCR service for automatic identification. This article will introduce in detail how to use PHP language and Alibaba Cloud OCR service to identify business licenses. 1. Introduction to Alibaba Cloud OCR Service Alibaba Cloud O
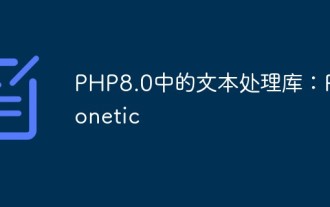
With the release of PHP8.0, many people are paying attention to its new features. One of its high-profile features is its text processing library, Phonetic. This library provides some useful methods such as phonetic symbol conversion, pinyin conversion, and approximate string matching. In this article, we will delve into the functionality and usage of this library. What is Phonetic? Phonetic is a library for processing text. It provides several methods to make text processing more convenient and accurate. This library integrates three main functions: audio

PHP technology sharing: Alibaba Cloud explores new areas of OCR and semantic analysis. With the continuous advancement of artificial intelligence technology and the widespread promotion of applications, text recognition (OCR) and semantic analysis are becoming increasingly important technical fields. As the leading cloud computing platform in China, Alibaba Cloud provides powerful OCR and semantic analysis APIs, providing developers with more convenient and faster development tools. This article will combine PHP language to deeply explore the application of Alibaba Cloud OCR and semantic analysis in actual projects, and attach corresponding code examples. 1. Alibaba Cloud
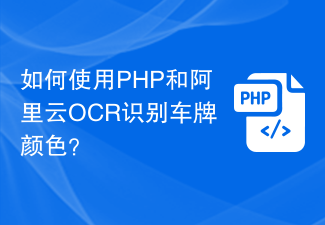
How to use PHP and Alibaba Cloud OCR to identify license plate color? In modern society, cars have become an important means of transportation in people's lives. Each vehicle has a unique license plate number, and the color of the license plate is also one of the important identifiers of the vehicle. In order to realize automated license plate color recognition, we can use the PHP programming language and Alibaba Cloud OCR service to implement this function. This article will introduce how to use PHP and Alibaba Cloud OCR to identify the license plate color, and provide code examples for readers to refer to and learn from. 1. Register for Alibaba Cloud OCR service first
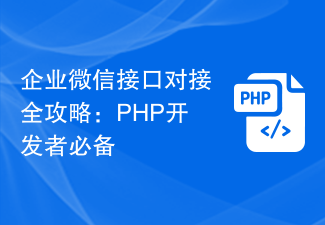
A complete guide to enterprise WeChat interface docking: a must-have for PHP developers. Under the current wave of enterprise informatization, more and more companies are beginning to use Enterprise WeChat as an internal communication and collaboration tool. As a developer, understanding and mastering the interface docking technology of Enterprise WeChat can provide enterprises with more customized functions and improve their work efficiency. This article will provide PHP developers with a comprehensive guide to enterprise WeChat interface docking, including interface calling methods and sample codes. 1. Introduction to Enterprise WeChat Enterprise WeChat is an instant messaging service launched by Tencent for enterprise users.
