


Vue and Axios implement performance monitoring and statistical analysis of front-end data requests
Vue and Axios implement performance monitoring and statistical analysis of front-end data requests
Front-end performance monitoring and statistical analysis play an important role in modern web application development, and it can help developers understand application performance bottlenecks and make corresponding optimizations. It is a common practice to use the Axios library to perform data requests in the Vue.js framework. This article will introduce how to combine Vue and Axios to implement performance monitoring and statistical analysis of front-end data requests, and give corresponding code examples.
First, we need to introduce the Axios library into the Vue project. In the main entry file of the project, introduce the Axios library through npm or CDN, and mount it on the Vue prototype to facilitate use in all components.
import Vue from 'vue' import axios from 'axios' Vue.prototype.$http = axios.create({ // 配置Axios相关参数,如请求的根URL、超时时间等 })
Next, we can define a statistical analysis class to record the performance indicators of data requests. The following is a simple example:
class PerformanceStats { constructor() { this.startTime = 0 this.endTime = 0 this.duration = 0 this.count = 0 } start() { this.startTime = performance.now() } end() { this.endTime = performance.now() this.duration += this.endTime - this.startTime this.count++ } getAverageDuration() { return this.duration / this.count } reset() { this.startTime = 0 this.endTime = 0 this.duration = 0 this.count = 0 } }
Before and after each data request, we can use Vue's life cycle hook function to record the performance indicators of the request. The following is an example component:
<template> <div> <!-- 根据需求添加具体的页面内容 --> </div> </template> <script> export default { data() { return { stats: new PerformanceStats() } }, methods: { fetchData() { this.stats.start() this.$http.get('/api/data') .then(response => { // 处理返回的数据 }) .finally(() => { this.stats.end() console.log('请求平均耗时:', this.stats.getAverageDuration()) this.stats.reset() }) } }, mounted() { this.fetchData() } } </script>
As you can see, in the fetchData method, we first call the start method of stats to record the start time, then use Axios to send a data request, and finally call the end method of stats after the request is completed. Record end time. The average time consumption can be obtained by calling the getAverageDuration method, and the stats are reset in finally so that the next request can be recorded.
Of course, we can expand the statistical analysis function according to specific needs. For example, you can record the maximum time-consuming, minimum time-consuming and other indicators of each request, or perform classified statistics based on different request types.
To sum up, Vue and Axios are commonly used tools in front-end development. Combining them can easily achieve performance monitoring and statistical analysis of front-end data requests. By recording the start and end time of requests and calculating indicators such as average time consumption, developers can better understand the performance of the application and make corresponding optimizations. I hope the code examples provided in this article will be helpful to your work!
The above is the detailed content of Vue and Axios implement performance monitoring and statistical analysis of front-end data requests. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
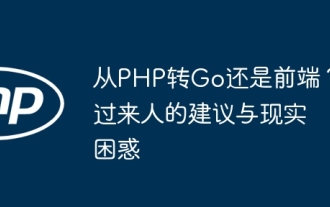
Confusion and the cause of choosing from PHP to Go Recently, I accidentally learned about the salary of colleagues in other positions such as Android and Embedded C in the company, and found that they are more...
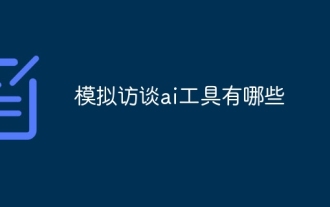
Mock interview AI tools are valuable tools for efficient candidate screening, saving recruiters time and effort. These tools include HireVue, Talview, Interviewed, iCIMS Video, and Eightfold AI. They provide automated, session-based assessments with benefits including efficiency, consistency, objectivity and scalability. When choosing a tool, recruiters should consider integrations, user-friendliness, accuracy, pricing, and support. Mock interviewing AI tools improve hiring speed, decision quality, and candidate experience.
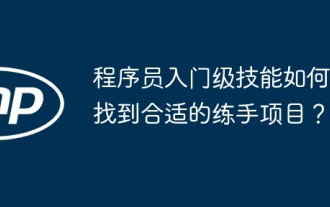
Programmers' "tickling" needs: From leisure to practice, this programmer friend has been a little idle recently and wants to improve his skills and achieve success through some small projects...
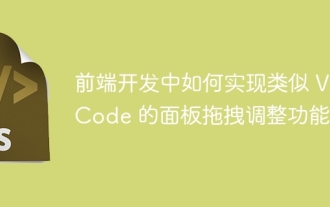
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
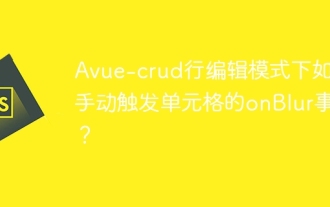
The onBlur event that implements Avue-crud row editing in the Avue component library manually triggers the Avue-crud component. It provides convenient in-line editing functions, but sometimes we need to...
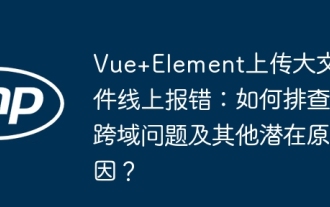
Vue Elementel-upload upload file online error reporting and troubleshooting using Vue and Element...
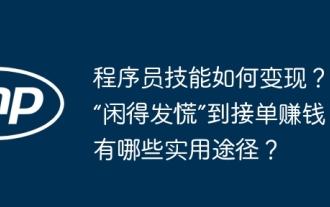
Programmers’ daily worries and skills monetization: from “I’m so idle” to “helpful” Recently, a programmer friend posted on a forum, expressing “I’m so idle…
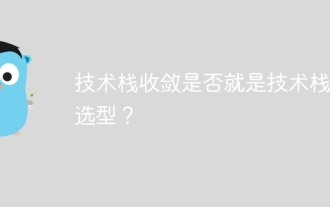
Title: The relationship between technology stack convergence and selection: Does technology stack convergence refer to the selection of technology stack? I saw an article that has a convergence technology stack...
