


PHP development practice: building highly scalable Modbus TCP communication applications
PHP Development Practice: Building a Highly Scalable Modbus TCP Communication Application
In the field of industrial automation, Modbus is a commonly used communication protocol for data transmission between devices. Modbus TCP is a Modbus variant based on the TCP/IP protocol, which realizes remote communication between devices through Ethernet. This article will introduce how to use PHP language to develop a highly scalable Modbus TCP communication application and provide relevant code examples.
First, we need to install a PHP extension library for communicating with Modbus devices. Here, we use the phpmodbus library, which provides a convenient set of functions for Modbus TCP communication. You can use Composer to install through the following command:
composer require phpmodbus/phpmodbus
After the installation is complete, we can start writing PHP code to implement the Modbus TCP communication function. The following is a simple example for reading the value of a register from a Modbus device:
<?php require_once('vendor/autoload.php'); use PHPModbusModbusMaster; // 设备的IP地址和端口号 $ip = '192.168.0.1'; $port = 502; // 创建一个ModbusMaster对象 $modbus = new ModbusMaster($ip, $port); // 读取保持寄存器的值(寄存器地址为0,数量为10) $result = $modbus->readMultipleRegisters(0, 10); // 输出结果 if (!empty($result)) { echo "读取成功:" . PHP_EOL; foreach ($result as $key => $value) { echo "寄存器" . $key . "的值:" . $value . PHP_EOL; } } else { echo "读取失败。" . PHP_EOL; } ?>
The above example code uses the ModbusMaster
class provided by the phpmodbus library to implement Modbus TCP communication. Multiple values can be read from registers through the readMultipleRegisters
function. The return result is an associative array, the key is the register address, and the value is the register value. We can process and use these values according to actual needs.
In addition to reading the value of the register, we can also perform write operations through the phpmodbus library, such as writing the value of the register, setting the status of the coil, etc. The following is a sample code for a write operation:
<?php // ... // 写入保持寄存器的值(寄存器地址为0,写入的值为100) $result = $modbus->writeSingleRegister(0, 100); // 输出结果 if ($result) { echo "写入成功。" . PHP_EOL; } else { echo "写入失败。" . PHP_EOL; } ?>
The above sample code uses the writeSingleRegister
function provided by the phpmodbus library to write the value of the register. After successful writing, the function returns true
, otherwise it returns false
. In this way, two-way communication can be carried out with Modbus devices to realize data reading and writing.
In addition to basic read and write operations, the phpmodbus library also provides other rich functions, such as reading coil status, writing values of multiple registers, etc. For specific usage methods and parameters, please refer to relevant documents and source code to meet the needs of actual projects.
In summary, by using the PHP language and the phpmodbus library, we can easily implement the Modbus TCP communication function. We can perform read and write operations according to actual needs to interact with Modbus devices. At the same time, the phpmodbus library provides rich functions and easy-to-use interfaces, making it easier and more convenient to develop highly scalable Modbus TCP communication applications.
Reference:
- phpmodbus library GitHub address: https://github.com/phpmodbus/phpmodbus
The above is the detailed content of PHP development practice: building highly scalable Modbus TCP communication applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

This article details methods to resolve event ID10000, which indicates that the Wireless LAN expansion module cannot start. This error may appear in the event log of Windows 11/10 PC. The WLAN extensibility module is a component of Windows that allows independent hardware vendors (IHVs) and independent software vendors (ISVs) to provide users with customized wireless network features and functionality. It extends the capabilities of native Windows network components by adding Windows default functionality. The WLAN extensibility module is started as part of initialization when the operating system loads network components. If the Wireless LAN Expansion Module encounters a problem and cannot start, you may see an error message in the event viewer log.
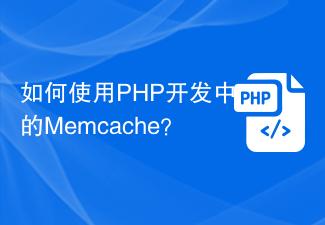
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
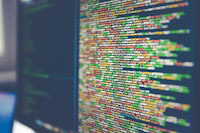
Using Prepared Statements Prepared statements in PDO allow the database to precompile queries and execute them multiple times without recompiling. This is essential to prevent SQL injection attacks, and it can also improve query performance by reducing compilation overhead on the database server. To use prepared statements, follow these steps: $stmt=$pdo->prepare("SELECT*FROMusersWHEREid=?");Bind ParametersBind parameters are a safe and efficient way to provide query parameters that can Prevent SQL injection attacks and improve performance. By binding parameters to placeholders, the database can optimize query execution plans and avoid performing string concatenation. To bind parameters, use the following syntax:
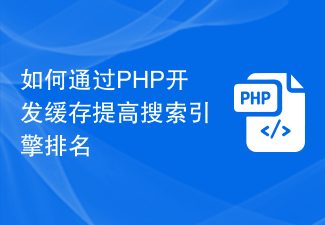
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
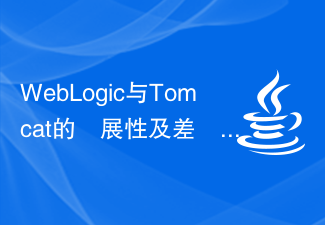
WebLogic and Tomcat are two commonly used Java application servers. They have some differences in scalability and functionality. This article will analyze the scalability of these two servers and compare the differences between them. First, let's take a look at WebLogic's scalability. WebLogic is a highly scalable Java application server developed by Oracle. It provides many advanced features, including transaction management, JDBC connection pooling, distributed caching, etc. WebLogic support
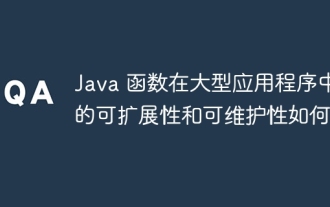
Java functions provide excellent scalability and maintainability in large applications due to the following features: Scalability: statelessness, elastic deployment and easy integration, allowing easy adjustment of capacity and scaling of deployment. Maintainability: Modularity, version control, and complete monitoring and logging simplify maintenance and updates. By using Java functions and serverless architecture, more efficient processing and simplified maintenance can be achieved in large applications.
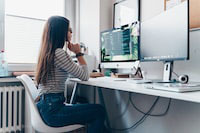
Java is a popular programming language for developing distributed systems and microservices. Its rich ecosystem and powerful concurrency capabilities provide the foundation for building robust, scalable applications. Kubernetes is a container orchestration platform that manages and automates the deployment, scaling, and management of containerized applications. It simplifies the management of microservices environments by providing features such as orchestration, service discovery, and automatic failure recovery. Advantages of Java and Kubernetes: Scalability: Kubernetes allows you to scale your application easily, both in terms of horizontal and vertical scaling. Resilience: Kubernetes provides automatic failure recovery and self-healing capabilities to ensure that applications remain available when problems arise. Agility
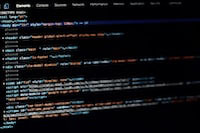
Advantages of JavaRESTfulAPI and Microservices JavaRESTfulAPI and microservices, as complementary technologies, offer a variety of advantages, including: Modularity: Microservices decompose applications into smaller independent components, improving code reusability and maintainability. Scalability: Microservices architecture allows applications to be easily scaled as needed without redeploying the entire system. Responsiveness: RESTful API follows a stateless protocol, ensuring high responsiveness and scalability. Integrating RESTful API with microservices Integrating RESTful API with microservices involves the following steps: 1. Define API endpoints: Identify the API endpoints that need to be exposed, and define the request and response formats for each endpoint. 2
