


How to use vue and Element-plus to implement data filtering and statistics
How to use Vue and Element Plus to implement data filtering and statistics
Introduction: Vue, as a popular front-end framework, combined with Element Plus, a powerful UI library, can easily implement data filtering and statistics Function. This article will introduce how to use Vue and Element Plus to implement this function, and show the specific implementation process through code examples.
1. Create a project and introduce Element Plus
First, use Vue CLI on the command line to create a new project:
vue create data-filter
Then enter the project and install Element Plus :
cd data-filter npm install element-plus
Introduce Element Plus into the main.js file:
import { createApp } from 'vue' import App from './App.vue' import ElementPlus from 'element-plus' import 'element-plus/lib/theme-chalk/index.css' createApp(App).use(ElementPlus).mount('#app')
2. Create data sources and filter components
In App.vue, we first create a data source and a component containing filter criteria. The data source can be an array, each element is an object containing various properties. Filter components can have multiple conditions, such as text input boxes, drop-down selection boxes, etc.
<template> <div> <div class="filters"> <el-input v-model="filterText" placeholder="请输入姓名进行筛选"></el-input> <el-select v-model="filterAge" placeholder="请选择年龄进行筛选"> <el-option label="18岁以下" value="18"></el-option> <el-option label="18-30岁" value="18-30"></el-option> <el-option label="30岁以上" value="30"></el-option> </el-select> </div> <div class="data-list"> <div v-for="item in filteredData" :key="item.id">{{ item.name }}</div> </div> </div> </template> <script> export default { data() { return { filterText: '', filterAge: '', data: [ { id: 1, name: '张三', age: 20 }, { id: 2, name: '李四', age: 25 }, { id: 3, name: '王五', age: 30 }, // ... ] } }, computed: { filteredData() { return this.data.filter(item => { return (item.name.includes(this.filterText) || item.age.toString() === this.filterAge) }) } } } </script>
In the above code, we defined a data source data in the data option, which contains some test data. The filter conditions include a text input box and a drop-down selection box, and the user can filter the data by entering a name and selecting an age. filteredData is a calculated property that is used to dynamically calculate data that meets the conditions based on the filtering conditions.
3. Writing statistical components
In addition to the filtering function, we can also perform data statistics through the chart component provided by Element Plus. In App.vue, we can write a statistical component to visually display the filtered data.
First, introduce echarts in the template, and then add a container containing the chart and a button to trigger the statistical function.
<template> <div> <div class="filters"> <!-- 筛选组件代码 --> </div> <div class="data-list"> <!-- 数据列表代码 --> </div> <div class="statistic-chart" ref="chart"></div> <el-button type="primary" @click="showStatisticChart">点击统计</el-button> </div> </template> <script> import echarts from 'echarts' export default { // ... methods: { showStatisticChart() { const chart = echarts.init(this.$refs.chart) const data = this.filteredData const names = data.map(item => item.name) const ages = data.map(item => item.age) const option = { tooltip: {}, legend: { data: ['年龄'] }, xAxis: { data: names }, yAxis: {}, series: [{ name: '年龄', type: 'bar', data: ages }] } chart.setOption(option) } } } </script>
In the showStatisticChart method, we use echarts to create a chart instance and pass in the chart container and filtered data. Then, define the style and data of the chart by setting options. In the above code, we show a simple histogram with the name on the x-axis and the age on the y-axis. Finally, call the chart.setOption method to apply the option to the chart.
4. Run the project
At this point, we have completed the coding of data filtering and statistical functions. Now run the project to see the effect.
npm run serve
Open the browser and enter http://localhost:8080 to see the running effect. Enter your name or select your age in the input box, and the data list will be updated in real time with data that meets the filtering conditions. Click the "Click Statistics" button, and a basic histogram will appear showing the age of each person.
Summary: Through Vue and Element Plus, we can easily implement data filtering and statistical functions. Using the UI components provided by Element Plus, we can quickly write pages with interactivity and visual effects. I hope this article will help you master how to use Vue and Element Plus to implement data filtering and statistics.
The above is the detailed content of How to use vue and Element-plus to implement data filtering and statistics. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
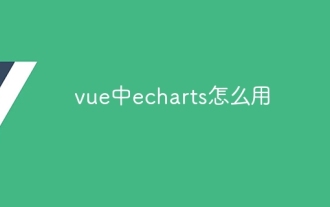
Using ECharts in Vue makes it easy to add data visualization capabilities to your application. Specific steps include: installing ECharts and Vue ECharts packages, introducing ECharts, creating chart components, configuring options, using chart components, making charts responsive to Vue data, adding interactive features, and using advanced usage.
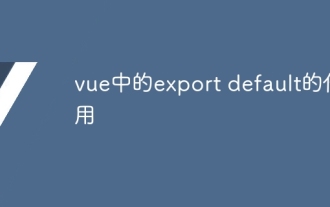
Question: What is the role of export default in Vue? Detailed description: export default defines the default export of the component. When importing, components are automatically imported. Simplify the import process, improve clarity and prevent conflicts. Commonly used for exporting individual components, using both named and default exports, and registering global components.
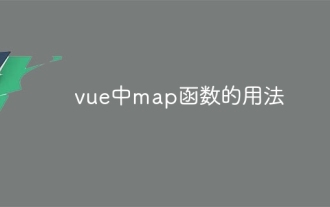
The Vue.js map function is a built-in higher-order function that creates a new array where each element is the transformed result of each element in the original array. The syntax is map(callbackFn), where callbackFn receives each element in the array as the first argument, optionally the index as the second argument, and returns a value. The map function does not change the original array.
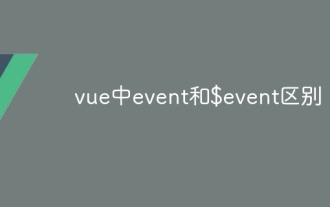
In Vue.js, event is a native JavaScript event triggered by the browser, while $event is a Vue-specific abstract event object used in Vue components. It is generally more convenient to use $event because it is formatted and enhanced to support data binding. Use event when you need to access specific functionality of the native event object.
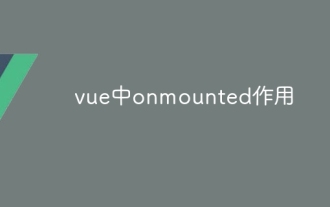
onMounted is a component mounting life cycle hook in Vue. Its function is to perform initialization operations after the component is mounted to the DOM, such as obtaining references to DOM elements, setting data, sending HTTP requests, registering event listeners, etc. It is only called once when the component is mounted. If you need to perform operations after the component is updated or before it is destroyed, you can use other lifecycle hooks.
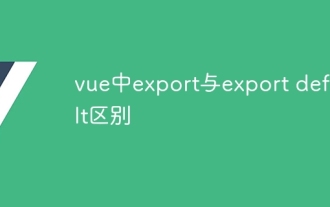
There are two ways to export modules in Vue.js: export and export default. export is used to export named entities and requires the use of curly braces; export default is used to export default entities and does not require curly braces. When importing, entities exported by export need to use their names, while entities exported by export default can be used implicitly. It is recommended to use export default for modules that need to be imported multiple times, and use export for modules that are only exported once.
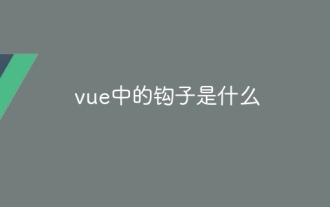
Vue hooks are callback functions that perform actions on specific events or lifecycle stages. They include life cycle hooks (such as beforeCreate, mounted, beforeDestroy), event handling hooks (such as click, input, keydown) and custom hooks. Hooks enhance component control, respond to component life cycles, handle user interactions and improve component reusability. To use hooks, just define the hook function, execute the logic and return an optional value.
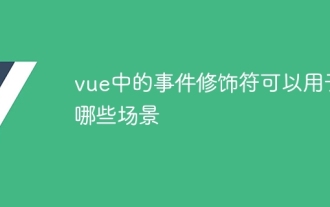
Vue.js event modifiers are used to add specific behaviors, including: preventing default behavior (.prevent) stopping event bubbling (.stop) one-time event (.once) capturing event (.capture) passive event listening (.passive) Adaptive modifier (.self)Key modifier (.key)
