


Golang's Template package: developing high-performance web applications
Golang's Template package: Develop high-performance Web applications
Introduction:
In Web development, the template engine is a very important component. It allows developers to combine dynamic data with static HTML templates to generate final web content. Golang's Template package provides an efficient and powerful way to work with templates. This article will introduce the basic usage of Golang's Template package, and show how to use it to develop high-performance web applications through some code examples.
- Introduction to Golang's Template package
Golang's Template package is a highly customizable template engine. It uses a syntax similar to the Go language and has a concise and flexible template definition method. It supports common template functions such as conditional statements, loop statements, variable definitions, etc., and also provides extended functions such as custom functions and custom methods. By using Golang's Template package, developers can separate dynamic data from HTML templates, improving code readability and maintainability. - Basic usage
The following is a simple code example that demonstrates how to use Golang's Template package to generate an HTML page. Suppose we have a simple data structure Person:
type Person struct { Name string Age int Email string }
We can define a template to insert the properties of the Person object into the corresponding location:
const tpl = ` <!DOCTYPE html> <html> <head> <title>User Info</title> </head> <body> <h1>User Info</h1> <p>Name: {{.Name}}</p> <p>Age: {{.Age}}</p> <p>Email: {{.Email}}</p> </body> </html> `
Then, we can use template.ParseFiles
The function parses the template file and creates a template object:
tmpl, err := template.New("userInfo").Parse(tpl)
Finally, we can combine the template with the data to generate the final HTML page:
var buf bytes.Buffer err = tmpl.Execute(&buf, person) if err != nil { log.Fatal(err) } fmt.Println(buf.String())
- Advanced Usage
Golang’s Template package also provides many advanced functions that can further improve development efficiency. The following are some common advanced usage examples:
a. Custom function
func multiply(a, b int) int { return a * b } tmpl, err := template.New("multiply").Funcs(template.FuncMap{ "multiply": multiply, }).Parse("{{multiply .A .B}}")
b. Conditional statement
tmpl, err := template.New("condition").Parse(` {{if .Visible}} <p>This is visible.</p> {{else}} <p>This is not visible.</p> {{end}} `)
c. Loop statement
type Book struct { Title string Author string } books := []Book{ {"Book 1", "Author 1"}, {"Book 2", "Author 2"}, {"Book 3", "Author 3"}, } tmpl, err := template.New("loop").Parse(` <ul> {{range .}} <li>{{.Title}} - {{.Author}}</li> {{end}} </ul> `)
Summary:
By using Golang’s Template package, developers can easily create high-performance web applications. This article introduces the basic usage of Golang's Template package and demonstrates its powerful functions through some code examples. I hope that readers can better master and apply Golang's Template package through the introduction of this article, thereby developing more high-performance web applications.
The above is the detailed content of Golang's Template package: developing high-performance web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


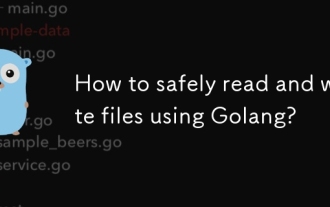
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
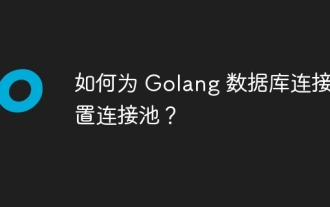
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
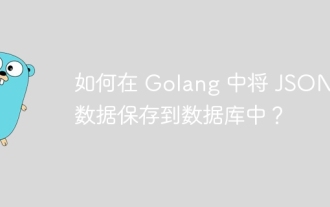
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
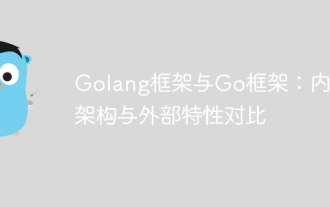
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
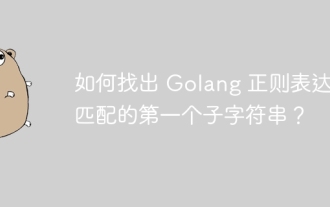
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
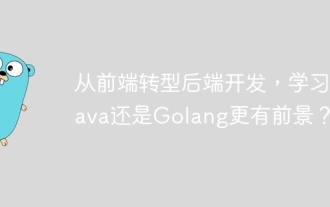
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
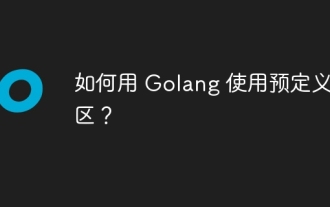
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
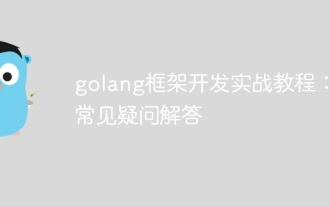
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
