Vue performance optimization tips and examples shared
Vue's performance optimization skills and examples are shared
Introduction:
Vue.js is one of the most popular JavaScript frameworks at the moment. With its concise syntax and flexible data binding capabilities, it is very popular on the front end. It has been widely used in development. However, as the project size grows and the data complexity increases, Vue's performance problems will gradually become apparent. This article will introduce you to some Vue performance optimization techniques and share specific code examples through examples.
1. Lazy loading of components
In large projects, the component files are huge. If all components are loaded at once, it will cause the page loading time to be too long. Vue's asynchronous component technology can help us load components on demand, thus improving the loading speed of the page. The following is an example:
// 定义异步组件 const AsyncComponent = () => ({ // 异步加载组件 component: import('./AsyncComponent.vue'), // 异步加载时显示的加载动画组件 loading: LoadingComponent, // 加载失败时显示的错误组件 error: ErrorComponent, // 组件加载完成后的延迟时间 delay: 200, // 设置超时时间,如果超过该时间组件未加载完成,显示加载失败组件 timeout: 3000 }) // 注册异步组件 Vue.component('async-component', AsyncComponent)
Wherever you need to use this component, you only need to make a simple call:
<template> <div> <async-component></async-component> </div> </template>
2. List performance optimization
In Vue development, we often A list is required to display data. But when the list data is too large, rendering the list will become very performance-intensive. In order to improve list performance, we can use the key attribute of Vue's v-for directive. This property helps Vue keep track of the identity of each node, allowing for efficient rendering.
<template> <div> <ul> <li v-for="(item, index) in list" :key="item.id"> {{ item.name }} </li> </ul> </div> </template> <script> export default { data() { return { list: [ { id: 1, name: '小明' }, { id: 2, name: '小红' }, // ... ] } } } </script>
By setting a unique key value for each list item, Vue will use this key to determine whether the node needs to be re-rendered, thus improving performance.
3. Performance Monitoring and Optimization
Vue provides some tools to monitor and diagnose performance problems. Among them, Vue Devtools is a very useful debugging tool that can help us analyze component performance issues. In addition, Vue also provides the performance testing tool perf, which can be used to detect and troubleshoot performance problems.
For example, we can use Vue Devtools to check the rendering time of the component:
// 在组件的mounted阶段进行监测 mounted() { console.time('component') this.$nextTick(() => { console.timeEnd('component') }) }
Calculate the rendering time of the component by using console.time and console.timeEnd in the mounted hook.
Conclusion:
This article introduces Vue’s performance optimization techniques and shares corresponding code examples. Through reasonable component lazy loading, list optimization and performance monitoring, we can improve the performance of Vue applications and provide a better user experience. I hope the above content is helpful to everyone.
The above is the detailed content of Vue performance optimization tips and examples shared. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
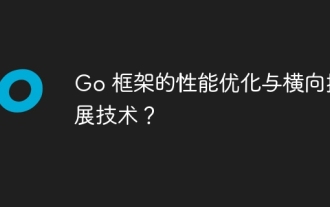
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.
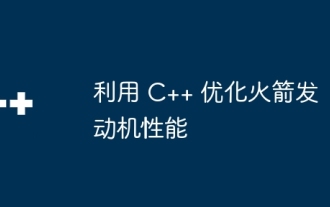
By building mathematical models, conducting simulations and optimizing parameters, C++ can significantly improve rocket engine performance: Build a mathematical model of a rocket engine and describe its behavior. Simulate engine performance and calculate key parameters such as thrust and specific impulse. Identify key parameters and search for optimal values using optimization algorithms such as genetic algorithms. Engine performance is recalculated based on optimized parameters to improve its overall efficiency.
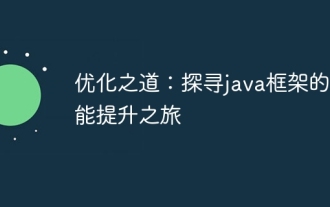
The performance of Java frameworks can be improved by implementing caching mechanisms, parallel processing, database optimization, and reducing memory consumption. Caching mechanism: Reduce the number of database or API requests and improve performance. Parallel processing: Utilize multi-core CPUs to execute tasks simultaneously to improve throughput. Database optimization: optimize queries, use indexes, configure connection pools, and improve database performance. Reduce memory consumption: Use lightweight frameworks, avoid leaks, and use analysis tools to reduce memory consumption.
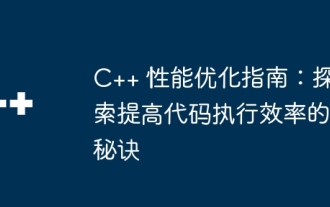
C++ performance optimization involves a variety of techniques, including: 1. Avoiding dynamic allocation; 2. Using compiler optimization flags; 3. Selecting optimized data structures; 4. Application caching; 5. Parallel programming. The optimization practical case shows how to apply these techniques when finding the longest ascending subsequence in an integer array, improving the algorithm efficiency from O(n^2) to O(nlogn).
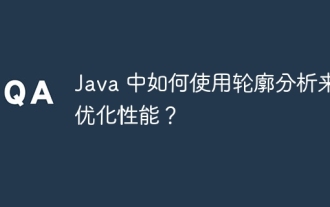
Profiling in Java is used to determine the time and resource consumption in application execution. Implement profiling using JavaVisualVM: Connect to the JVM to enable profiling, set the sampling interval, run the application, stop profiling, and the analysis results display a tree view of the execution time. Methods to optimize performance include: identifying hotspot reduction methods and calling optimization algorithms
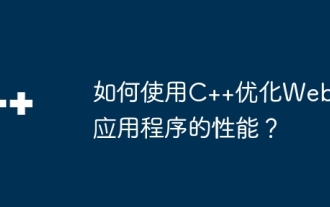
C++ techniques for optimizing web application performance: Use modern compilers and optimization flags to avoid dynamic memory allocations Minimize function calls Leverage multi-threading Use efficient data structures Practical cases show that optimization techniques can significantly improve performance: execution time is reduced by 20% Memory Overhead reduced by 15%, function call overhead reduced by 10%, throughput increased by 30%
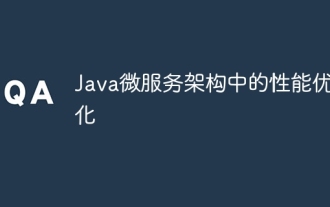
Performance optimization for Java microservices architecture includes the following techniques: Use JVM tuning tools to identify and adjust performance bottlenecks. Optimize the garbage collector and select and configure a GC strategy that matches your application's needs. Use a caching service such as Memcached or Redis to improve response times and reduce database load. Employ asynchronous programming to improve concurrency and responsiveness. Split microservices, breaking large monolithic applications into smaller services to improve scalability and performance.
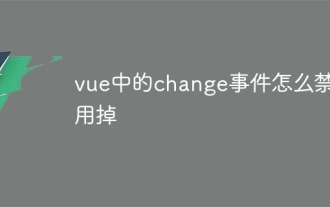
In Vue, the change event can be disabled in the following five ways: use the .disabled modifier to set the disabled element attribute using the v-on directive and preventDefault using the methods attribute and disableChange using the v-bind directive and :disabled
