How to write Modbus TCP communication code using PHP
How to use PHP to write Modbus TCP communication code
Modbus is a communication protocol used in the field of industrial automation and is widely used for data transmission between PLC (programmable logic controller) and other automation equipment . Modbus TCP is a variant of the Modbus protocol that uses the TCP/IP protocol stack as the transport layer to allow remote communication over the network.
This article will introduce how to use PHP to write Modbus TCP communication code and provide some code examples.
- Install the PHP extension that supports Modbus TCP
First, you need to install the PHP Modbus extension, which provides functions and methods for operating the Modbus protocol. You can find various extensions such as phpmodbus and phpmodbuscli on the PECL (PHP Extension Community Library) website. Depending on your operating system and PHP version, select and install the appropriate extension. - Create Modbus TCP connection
In PHP, you can use the modbus_new function to create a Modbus TCP connection. The following is the sample code:
<?php // 创建Modbus TCP连接 $modbus = modbus_new_tcp("192.168.1.1", 502); if(!$modbus) { die("无法创建Modbus TCP连接"); } ?>
In the above code, we use the modbus_new_tcp function to create a Modbus TCP connection, and the parameters include the IP address and port number of the remote device. If the connection creation fails, we use the die function to output an error message and terminate the program.
- Read Modbus Registers
Once the Modbus TCP connection is created, we can use the modbus_read_registers function to read the specified registers. The following is the sample code:
<?php // 读取Modbus寄存器 $address = 0; // 起始寄存器的地址 $count = 10; // 要读取的寄存器数量 $data = modbus_read_registers($modbus, $address, $count); if(!$data) { die("无法读取Modbus寄存器"); } ?>
In the above code, we use the modbus_read_registers function to read the specified number of registers starting from the specified address. If the read fails, we use the die function to output an error message and terminate the program.
- Write Modbus register
In addition to reading the register, we can also use the modbus_write_register function to write to the specified register. The following is the sample code:
<?php // 写入Modbus寄存器 $address = 0; // 要写入寄存器的地址 $value = 100; // 要写入的值 $result = modbus_write_register($modbus, $address, $value); if(!$result) { die("无法写入Modbus寄存器"); } ?>
In the above code, we use the modbus_write_register function to write the specified value to the specified register. If the write fails, we use the die function to output an error message and terminate the program.
- Close Modbus TCP connection
After completing Modbus communication, we should use the modbus_close function to close the Modbus TCP connection to release resources. The following is the sample code:
<?php // 关闭Modbus TCP连接 modbus_close($modbus); ?>
In the above code, we use the modbus_close function to close the Modbus TCP connection created earlier.
Summary
This article introduces how to use PHP to write Modbus TCP communication code. First, we need to install the PHP extension that supports Modbus TCP. We then create a Modbus TCP connection and perform data transfer by reading and writing registers. Finally, we close the Modbus TCP connection to release resources. I hope these code examples can help you implement Modbus TCP communication in PHP and find application in the field of industrial automation.
The above is the detailed content of How to write Modbus TCP communication code using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


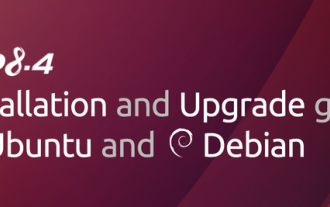
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
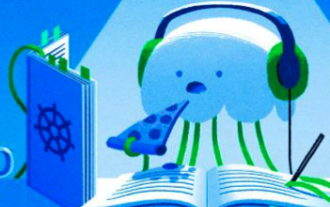
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
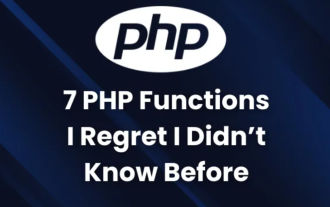
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
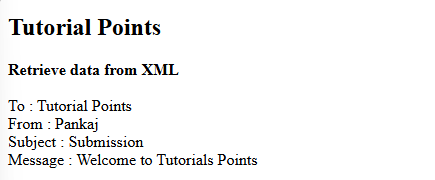
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
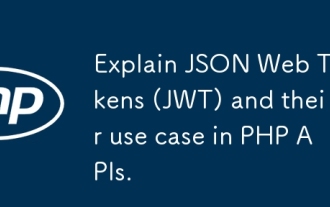
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
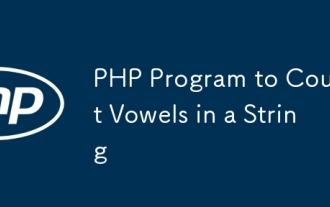
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
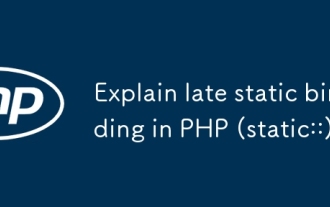
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
