


Golang and the Template package: creating personalized user interfaces
Golang and Template package: Create personalized user interface
In modern software development, the user interface is often the most direct way for users to interact with the software. In order to provide a user interface that is easy to use and beautiful, developers need flexible tools to create and customize the user interface. In Golang, developers can use the Template package to achieve this goal. This article will introduce the basic usage of Golang and the Template package, and show how to create a personalized user interface through code examples.
Template package is a package for template engine officially provided by Golang. It combines templates and data to generate the final output. By using the Template package, developers can separate business logic and views, better organize code and customize the interface.
First of all, we need to understand the two core concepts in the Template package: template and context. A template is a text file with placeholders surrounded by double curly braces, such as {{.}}. The context is a structure containing the values corresponding to the placeholders in the template.
The following is a simple code example that shows how to use the Template package to create a basic user interface:
package main import ( "os" "text/template" ) type User struct { Name string Age int Gender string } func main() { user := User{Name: "张三", Age: 25, Gender: "男"} tplText := "姓名: {{.Name}} 年龄: {{.Age}} 性别: {{.Gender}} " tpl, err := template.New("user").Parse(tplText) if err != nil { panic(err) } err = tpl.Execute(os.Stdout, user) if err != nil { panic(err) } }
In the above example, we first define a User structure, representing User information. Then we define a template string tplText, which contains three placeholders {{.Name}}, {{.Age}} and {{.Gender}}. Then we use template.New("user").Parse(tplText) to create a template named "user" and parse the template string into this template. Finally, we call tpl.Execute(os.Stdout, user) to pass the context user to the template for rendering, and output the result to standard output.
When we run the above code, we will get the following output:
姓名: 张三 年龄: 25 性别: 男
The above demonstrates a simple example. In fact, the Template package provides more functions, such as conditional statements and loop statements. Etc., developers can use these functions to create more complex user interfaces. Next we will show more uses of the Template package through a more complex example.
package main import ( "os" "text/template" ) type Product struct { Name string Price float64 } type User struct { Name string Products []Product } func main() { user := User{ Name: "张三", Products: []Product{ {"产品1", 10.0}, {"产品2", 20.0}, {"产品3", 30.0}, }, } tplText := `姓名: {{.Name}} 产品列表: {{range .Products}} - {{.Name}}: ¥{{.Price}} {{end}}` tpl, err := template.New("user").Parse(tplText) if err != nil { panic(err) } err = tpl.Execute(os.Stdout, user) if err != nil { panic(err) } }
In the above example, we defined a more complex data structure User and included a slice of Product. We use the range statement to traverse the Product slices and render each Product. The final template output is as follows:
姓名: 张三 产品列表: - 产品1: ¥10.0 - 产品2: ¥20.0 - 产品3: ¥30.0
Through the above example, we can see that the Template package has strong flexibility and scalability, and developers can customize the user interface according to their own needs. Whether it is a simple user interface or a complex interface, the Template package can help developers achieve personalized designs.
To summarize, this article introduces the Template package in Golang and how to use it to create personalized user interfaces. Through the Template package, developers can separate business logic and views, better organize code and implement interface customization. I hope this article can provide readers with some basic knowledge about Golang and the Template package, and play a certain guiding role in actual development.
The above is the detailed content of Golang and the Template package: creating personalized user interfaces. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


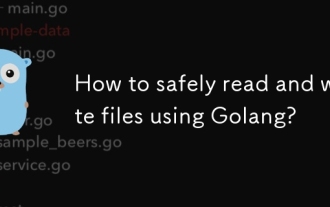
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
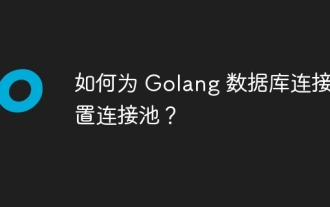
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
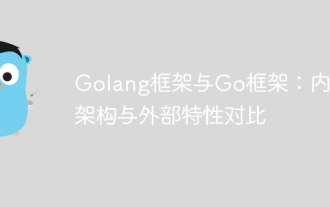
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
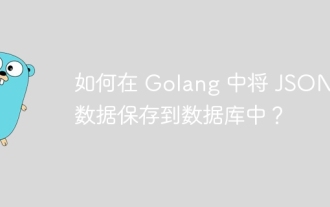
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
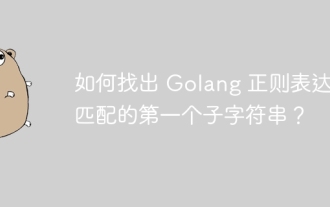
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
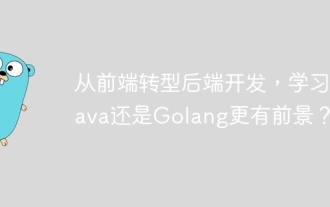
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
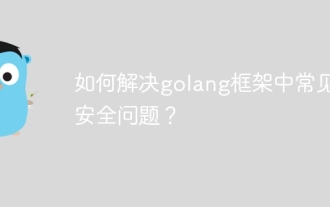
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
