How to use vue and Element-plus to group and sort data
How to use Vue and Element Plus to group and sort data
Vue is a popular JavaScript framework that can help us build front-end applications. Element Plus is a desktop component library based on Vue. It provides a rich set of UI components, allowing us to easily build beautiful and user-friendly interfaces. In this article, we will explore how to use Vue and Element Plus to group and sort data.
First, we need to prepare some basic code. We assume that Vue and Element Plus have been installed and the project has been configured accordingly. In the template of the Vue component, we can use the table component provided by Element Plus to display table data.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
|
In the above code, we use the table component to display table data. The data attribute of the table is bound to an array tableData, which contains the data we want to display. Next, we need to add some code for the grouping and sorting functionality.
The grouping function can be implemented through the slot-scope of the table. We can customize a column to group the data that needs to be grouped, and display the grouped information above the table.
1 2 3 4 5 6 |
|
In the above code, we use the label attribute to specify the column title as "Group", and use v-slot to define the content of the column. In v-slot, we can access the data of the current row and obtain group information through the getGroup method.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In the above code, we defined the getGroup method to make logical judgments about grouping based on the age attribute of the data. If the age is less than 25 years old, return the "youth group", otherwise return the "middle-aged group". By adding a custom content above the table, we can display grouping information dynamically.
The sorting function can be achieved by setting the sortable attribute of the table column. In the above code, we set the sortable attribute for the three columns of name, age and gender. In this way, the user can perform sorting operations by clicking the sorting icon at the head of the table.
The above code is just a simple example. We can customize the grouping and sorting logic according to actual business needs. By using the rich components and functions provided by Vue and Element Plus, we can easily implement grouping and sorting of data, as well as other complex front-end needs. I hope this article can help you in the development of Vue and Element Plus!
The above is the detailed content of How to use vue and Element-plus to group and sort data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










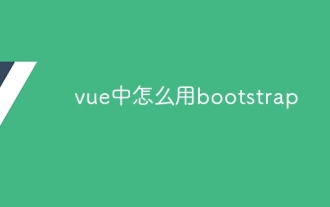
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
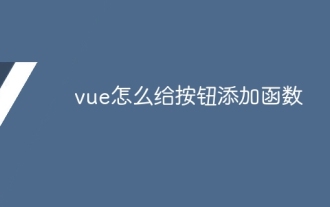
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
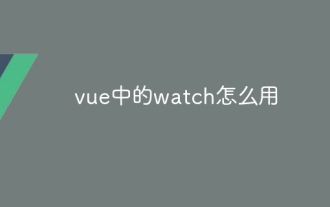
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
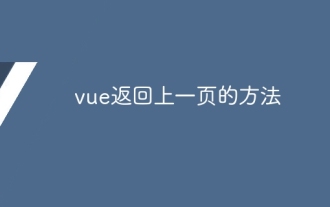
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
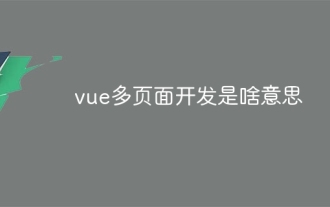
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema
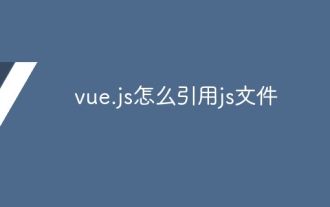
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
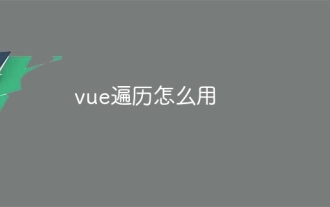
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
