Vue and Canvas: How to blur and sharpen images
Jul 18, 2023 pm 12:45 PMVue and Canvas: How to achieve image blurring and sharpening effects
Introduction:
With the development of web applications, image processing and special effects have become an increasingly important part of front-end development. . As a popular JavaScript framework, Vue.js also plays an important role in image processing. Canvas is a powerful technology in HTML5 that can be used for image processing. This article will introduce how to use Vue and Canvas to achieve image blurring and sharpening effects, and provide relevant code examples.
1. Image processing in Vue
As a responsive JavaScript framework, Vue.js provides many instructions and capabilities to process images. In image processing, we usually need to load the image first and then process it. The following is a simple Vue code for loading an image:
<template> <div> <input type="file" @change="onFileChange"> <img :src="imageUrl" alt="图像"> </div> </template> <script> export default { data() { return { imageUrl: '', }; }, methods: { onFileChange(event) { const file = event.target.files[0]; const reader = new FileReader(); reader.onload = (e) => { this.imageUrl = e.target.result; }; reader.readAsDataURL(file); }, }, }; </script>
In the above code, we use the <input type="file">
element to let the user select an image file . When the user selects a file, we use the FileReader
object to read the file content and convert it into a Base64-encoded string. After that, we assign this string to the imageUrl
variable to display the image on the page.
2. Image processing in Canvas
Canvas is a new element in HTML5, which can be used to implement functions such as image processing, animation, and visualization. In Canvas, we can achieve image processing effects by drawing 2D images and using various drawing methods. The following is a simple Vue and Canvas code example for displaying and processing the blur effect of an image:
<template> <div> <canvas ref="canvas" :width="canvasWidth" :height="canvasHeight"></canvas> </div> </template> <script> export default { data() { return { canvasWidth: 500, canvasHeight: 400, }; }, mounted() { const canvas = this.$refs.canvas; const context = canvas.getContext('2d'); const imageUrl = 'https://example.com/image.jpg'; // 图像地址 const image = new Image(); image.onload = () => { context.drawImage(image, 0, 0, this.canvasWidth, this.canvasHeight); this.applyBlurEffect(context); // 应用模糊效果 }; image.src = imageUrl; }, methods: { applyBlurEffect(context) { const imageData = context.getImageData(0, 0, this.canvasWidth, this.canvasHeight); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { const red = data[i]; const green = data[i + 1]; const blue = data[i + 2]; const alpha = data[i + 3]; // 简单的模糊算法,取上下左右四个像素的平均值 const blurRed = (data[i - 4] + data[i + 4] + data[i - this.canvasWidth * 4] + data[i + this.canvasWidth * 4]) / 4; const blurGreen = (data[i - 3] + data[i + 5] + data[i - this.canvasWidth * 4 + 1] + data[i + this.canvasWidth * 4 + 1]) / 4; const blurBlue = (data[i - 2] + data[i + 6] + data[i - this.canvasWidth * 4 + 2] + data[i + this.canvasWidth * 4 + 2]) / 4; data[i] = blurRed; data[i + 1] = blurGreen; data[i + 2] = blurBlue; } context.putImageData(imageData, 0, 0); }, }, }; </script>
In the above code, we create a Canvas element in Vue and obtain its 2D context. Then, we use the Image object to load the image, and after the image is loaded, call the drawImage
method to draw the image on the Canvas. Finally, we can apply the blur effect by calling the applyBlurEffect
method. In this method, we use the getImageData
method to obtain the image data, then blur the pixels, and finally draw the processed image data back to the Canvas.
3. Implementation of sharpening effect
In addition to the blurring effect, Canvas can also achieve the sharpening effect of the image. The following is a simple code example:
<template> <div> <canvas ref="canvas" :width="canvasWidth" :height="canvasHeight"></canvas> </div> </template> <script> export default { data() { return { canvasWidth: 500, canvasHeight: 400, }; }, mounted() { const canvas = this.$refs.canvas; const context = canvas.getContext('2d'); const imageUrl = 'https://example.com/image.jpg'; // 图像地址 const image = new Image(); image.onload = () => { context.drawImage(image, 0, 0, this.canvasWidth, this.canvasHeight); this.applySharpenEffect(context); // 应用锐化效果 }; image.src = imageUrl; }, methods: { applySharpenEffect(context) { const imageData = context.getImageData(0, 0, this.canvasWidth, this.canvasHeight); const data = imageData.data; const weights = [ 0, -1, 0, -1, 5, -1, 0, -1, 0, ]; for (let i = 0; i < data.length; i += 4) { const red = data[i]; const green = data[i + 1]; const blue = data[i + 2]; const alpha = data[i + 3]; let blurRed = 0; let blurGreen = 0; let blurBlue = 0; for (let j = -1; j <= 1; j++) { for (let k = -1; k <= 1; k++) { const index = i + (j * this.canvasWidth * 4) + (k * 4); const weight = weights[(j + 1) * 3 + (k + 1)]; blurRed += data[index] * weight; blurGreen += data[index + 1] * weight; blurBlue += data[index + 2] * weight; } } data[i] = red + blurRed; data[i + 1] = green + blurGreen; data[i + 2] = blue + blurBlue; } context.putImageData(imageData, 0, 0); }, }, }; </script>
In the above code, we define a 3*3 size sharpening matrix weights
, which is used to calculate the sharpness of each pixel . Then, for each pixel, we multiply the weight of the corresponding position in the surrounding 8 pixels, and add the value of the current pixel to obtain the sharpened pixel value. Finally, we draw the processed image data back into Canvas.
Summary:
This article introduces how to use Vue and Canvas to achieve image blurring and sharpening effects. Through the responsiveness of Vue.js and the powerful functions of Canvas, we can easily achieve various image processing effects. I hope the code examples in this article can help you better understand and apply image processing technology in Vue and Canvas.
The above is the detailed content of Vue and Canvas: How to blur and sharpen images. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
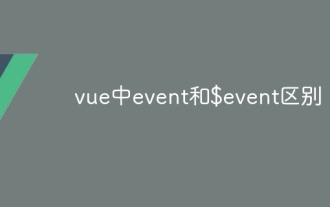
The difference between event and $event in vue
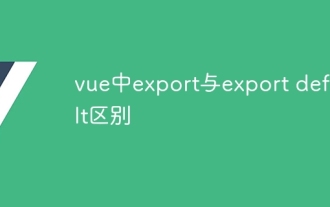
The difference between export and export default in vue
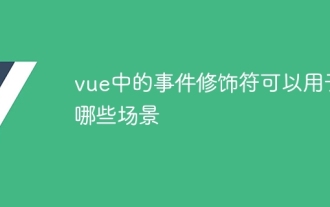
What scenarios can event modifiers in vue be used for?
