


How to optimize the application's status monitoring performance through Vue's watch attribute
How to optimize the application's status monitoring performance through Vue's watch attribute
Vue is a popular JavaScript framework for building user interfaces. During the development process, it is often necessary to monitor changes in data to make corresponding operations. Vue provides the watch attribute to monitor data. However, when there is a lot of data to be monitored, using watch may cause performance problems. This article will introduce how to improve the status monitoring performance of the application by optimizing the watch attribute.
1. Problem Analysis
Before understanding how to optimize the watch attribute, let’s first understand why there are performance problems. When the data that needs to be monitored changes, the watch attribute will immediately execute the corresponding callback function. If there is a lot of data to be monitored, each data change will trigger all callback functions in the watch attribute, causing performance problems.
2. Use deep monitoring
Vue’s watch attribute only monitors reference changes of objects or arrays by default, and does not deeply traverse the properties inside the object. If you need to monitor changes in the internal properties of an object, you can use deep monitoring. In the watch attribute, deep monitoring is implemented by setting the immediate and deep parameters.
watch: { obj: { immediate: true, deep: true, handler: function(val, oldVal) { // 监听到obj的变化后执行的操作 } } }
In the above code, setting the immediate parameter to true means that the handler function will be executed immediately when the component is created. The deep parameter is set to true to deeply monitor changes in the internal properties of obj. Through deep monitoring, the handler function will be executed only when the internal properties of obj change, avoiding unnecessary performance consumption.
3. Use calculated properties instead of watch
In addition to the watch property, Vue also provides calculated properties to monitor data. Computed properties are cached based on their dependencies and are only re-evaluated when dependencies change. In contrast, the watch attribute needs to traverse all monitored data, and there is a certain difference in performance.
For example, we have a requirement to display the length of the input content in the user input box in real time. This can be achieved using the watch attribute:
data: { inputText: '', textLength: 0 }, watch: { inputText: function(val) { this.textLength = val.length; } }
In the above code, the watch attribute monitors changes in inputText. When inputText changes, the length of the input content is assigned to textLength. But every time content is entered in the input box, the callback function of the watch attribute will be triggered, regardless of whether the content changes.
If you use calculated properties, you can monitor the length of the input content more efficiently:
data: { inputText: '' }, computed: { textLength: function() { return this.inputText.length; } }
In the above code, the calculated property textLength will only recalculate the length when the value of inputText changes, to avoid unnecessary calculations.
4. Use $nextTick to delay processing
Sometimes, we need to perform asynchronous operations after listening to data changes, such as sending requests or operating DOM elements. If you perform these operations directly in the callback function of the watch attribute, errors may occur because the DOM has not been updated.
Vue provides the $nextTick method to handle this situation. The $nextTick method will execute a delayed callback after the next DOM update cycle. By using the $nextTick method, you can ensure that data changes are monitored before performing asynchronous operations.
watch: { data: function(val, oldVal) { this.$nextTick(function() { // 异步操作代码 }); } }
In the above code, after the watch attribute detects changes in data, it uses $nextTick to asynchronously execute the operation code after the DOM is updated to ensure that the DOM has been updated.
Summary:
For situations where a large amount of data changes need to be monitored, the watch attribute can be optimized through deep monitoring, calculated properties and $nextTick to improve the application's status monitoring performance. Deep monitoring avoids unnecessary monitoring, calculated properties cache calculation results, and $nextTick ensures the correct execution of asynchronous operations. Proper use of these optimization methods can improve application performance and user experience.
The above is the detailed content of How to optimize the application's status monitoring performance through Vue's watch attribute. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


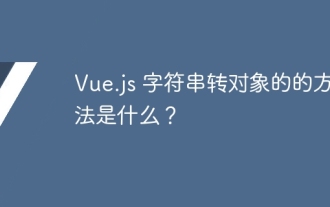
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.
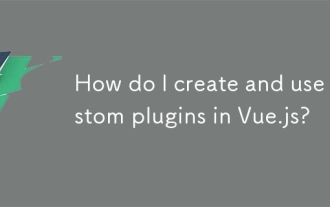
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
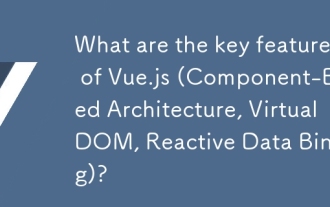
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
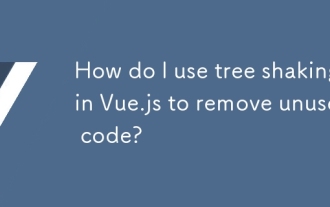
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159
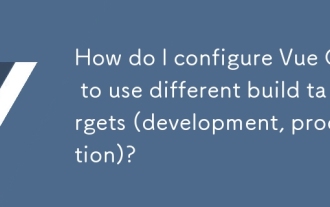
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
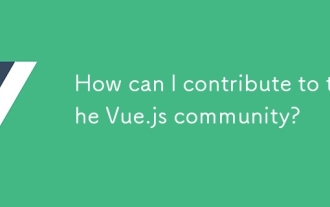
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje
