


How to use vue and Element-plus to achieve the linkage effect of paging and search
How to use Vue and Element-plus to achieve the linkage effect of paging and search
In modern web development, it is a very common requirement to achieve the linkage effect of paging and search. Vue and Element-plus are two frameworks and libraries widely used in front-end development. They provide a wealth of components and tools that can easily help us achieve this function. This article will use an example to demonstrate how to use Vue and Element-plus to achieve the linkage effect of paging and search.
First, we need to prepare a simple data list, such as an array containing multiple objects. To simulate a real scenario, we assume that each object has a name attribute and we will search by name. At the same time, we also need to consider the paging function, that is, each page displays a certain amount of data, and users can view more data by turning the pages.
In the HTML part, we will use the components provided by Element-plus to implement paging and search functions. First, we need an input box and a button for searching. The input box is bound to a variable searchText
, and a search function is triggered when the button is clicked. Secondly, we also need a paging component, including page numbers and page turning buttons. We will bind the current page number to a variable currentPage
, and trigger a function to jump to the page when the page turn button is clicked.
<template> <div> <input v-model="searchText" placeholder="请输入搜索内容" /> <el-button type="primary" @click="search">搜索</el-button> <div v-for="item in displayedItems" :key="item.id">{{ item.name }}</div> <el-pagination @current-change="handlePageChange" :current-page="currentPage" :page-sizes="[10, 20, 30, 40]" :page-size="pageSize" layout="total, sizes, prev, pager, next" :total="total" ></el-pagination> </div> </template>
Next, we define the relevant logic and data in the JavaScript part. First, we define the data array items
in the data
property of the Vue instance. Then, we define a calculated property displayedItems
, which will return the corresponding data based on the current page number and search content. At the same time, we also need to maintain a total
variable to represent the total amount of data, which is used to calculate paging logic.
<script> import { reactive } from 'vue' export default { data() { return { items: [ { id: 1, name: 'Apple' }, { id: 2, name: 'Banana' }, { id: 3, name: 'Orange' }, // ... ], searchText: '', currentPage: 1, pageSize: 10, total: 0, } }, computed: { displayedItems() { return this.items.filter(item => item.name.includes(this.searchText)) .slice((this.currentPage - 1) * this.pageSize, this.currentPage * this.pageSize) } }, methods: { search() { // 根据搜索内容重新计算分页和数据 this.currentPage = 1 }, handlePageChange(currentPage) { // 更新当前页码 this.currentPage = currentPage } } } </script>
In order for the code to run normally, we also need to import and register Element-plus components in this file. In Vue 3, you can use import { ElButton, ElInput, ElPagination } from 'element-plus'
to import related components, and then register them in the components
property. If you use the Vue 2 version, the import and registration methods are slightly different, please adjust according to your specific situation.
Finally, create a Vue application in the entry file and mount it on the page.
At this point, we have completed the linkage effect of paging and search using Vue and Element-plus. Users can search for data that meets the conditions through the input box and browse more data through the paging component. This example is just a simple demonstration, you can extend and optimize the function according to actual needs.
Summary: Using the components and tools provided by Vue and Element-plus, we can easily achieve the linkage effect of paging and search. By binding data and events, combined with computed properties and methods, we can easily handle user input and page jumps, and implement data interaction between different components. Hopefully the examples in this article will help you better understand and apply these techniques. If you want to learn more about the functions and usage of Vue and Element-plus, please refer to the official documentation and related tutorials.
The above is the detailed content of How to use vue and Element-plus to achieve the linkage effect of paging and search. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


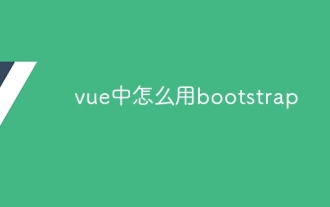
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
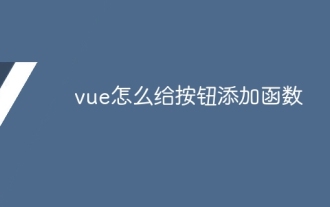
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
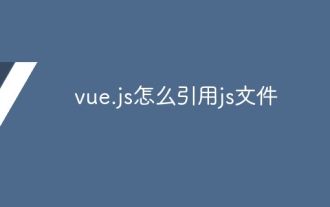
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
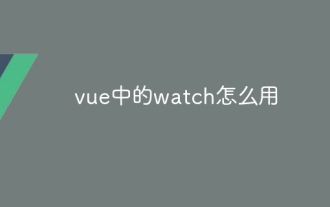
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
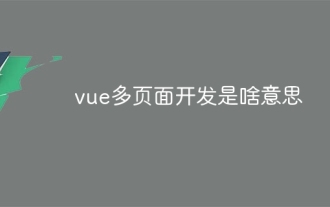
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
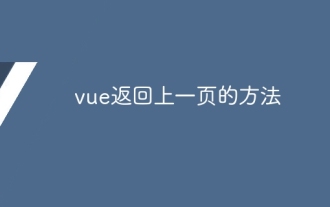
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
