


How to improve application performance through asynchronous updates of Vue's responsive system
How to improve application performance through asynchronous updates of Vue's responsive system
Modern web applications are becoming more and more complex and large. In order to maintain the performance of the application, unnecessary re-rendering should be reduced while updating data. is very critical. As a popular JavaScript framework, Vue.js provides a powerful responsive system and component-based architecture, which can help us efficiently build maintainable and high-performance applications.
Vue's reactive system is based on dependency tracking, which automatically tracks the properties and dependencies used in components and triggers updates when related data changes. However, since JavaScript is single-threaded, when there is a large amount of data that needs to be updated, synchronous updates may cause the application to block and freeze. In order to solve this problem, Vue provides an asynchronous update mechanism that can delay a batch of update operations to the next event loop to improve application performance.
In Vue, asynchronous updates are mainly implemented in the following two ways:
- Use the Vue.nextTick() method
The Vue.nextTick() method is a function of Vue Global method used to delay the execution of the callback function to the next event loop. After the data changes, we can use the Vue.nextTick() method to ensure that the DOM has been updated, and then perform some necessary operations.
The sample code is as follows:
// 异步更新数据 this.message = 'Hello, Vue.js!'; // 等待DOM更新完毕后执行回调 Vue.nextTick(function () { // 执行一些必要的操作 });
- Using Vue’s calculated properties and watch properties
Computed properties and watch properties are very powerful and flexible features in Vue. Used to respond to changes in data and perform some asynchronous operations.
Computed property is a function defined in the Vue component, which calculates a new value based on changes in some data. This function automatically caches the calculation results and will only recalculate them when the relevant data changes. We can perform some asynchronous operations in computed properties, such as requesting background data, etc.
The sample code is as follows:
// 在Vue组件中定义一个计算属性 computed: { asyncData: function() { // 执行异步操作,例如请求后台数据 return axios.get('/api/data').then((response) => { return response.data; }); } }
The watch attribute is another feature in Vue, which is used to monitor changes in data and execute the corresponding callback function. We can perform some asynchronous operations in the callback function to respond to data changes.
The sample code is as follows:
// 在Vue组件中定义一个watch属性 watch: { message: { handler: function(newVal, oldVal) { // 执行异步操作,例如发送事件埋点等 }, immediate: true // 立即触发回调函数 } }
By using Vue’s asynchronous update mechanism, we can delay some time-consuming and unnecessary operations to the next event loop, thereby reducing unnecessary Repeated calculations and rendering improve application performance. However, it should be noted that asynchronous updates are not suitable for all scenarios. We need to decide whether to use it based on specific business needs and performance requirements.
To summarize, asynchronous updates through Vue’s responsive system can fundamentally improve application performance. We can use features such as the Vue.nextTick() method, computed properties, and watch properties to defer time-consuming and unnecessary operations to the next event loop. This can reduce unnecessary re-rendering and optimize application response speed and user experience.
The above is the detailed content of How to improve application performance through asynchronous updates of Vue's responsive system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


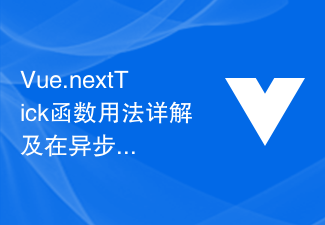
Detailed explanation of the usage of Vue.nextTick function and its application in asynchronous updates. In Vue development, we often encounter situations where data needs to be updated asynchronously. For example, data needs to be updated immediately after modifying the DOM or related operations need to be performed immediately after the data is updated. The .nextTick function provided by Vue emerged to solve this type of problem. This article will introduce the usage of the Vue.nextTick function in detail, and combine it with code examples to illustrate its application in asynchronous updates. 1. Vue.nex
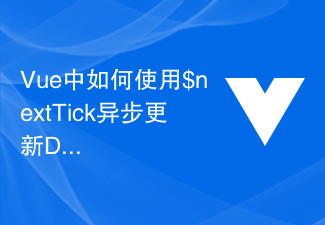
Vue is a popular JavaScript framework that is widely used to build single-page applications. It adopts responsive data binding and componentized architecture, and provides many convenient tools and methods. In Vue, when data changes, Vue automatically updates the view to reflect those changes. However, there are situations where we need to manipulate DOM elements immediately after the data is updated, such as when we need to add a new item to a list. At this time, we can use the $nextTick method provided by Vue to asynchronously update D
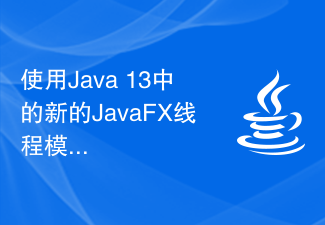
Use the new JavaFX thread model in Java13 to implement asynchronous updates of the UI interface. Introduction: In software development, the response speed of the user interface is very important to the user experience. In order to ensure the smoothness and timeliness of the interface, developers need to use an asynchronous way to update the user interface. In previous versions, JavaFX used the JavaFX application thread (JavaFXApplicationThread) to update the UI interface, but it was easy to
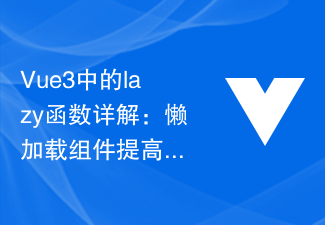
Detailed explanation of lazy function in Vue3: Application of lazy loading components to improve application performance In Vue3, using lazy loading components can significantly improve application performance. Vue3 provides lazy function for loading components asynchronously. In this article, we will learn more about how to use the lazy function and introduce some application scenarios of lazy loading components. The lazy function is one of the built-in features in Vue3. When using the lazy function, Vue3 will not load the component during the initial rendering, but will load it when the component is needed.
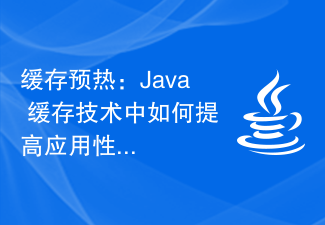
With the continuous development of Internet technology, a large number of users and massive data access have become common phenomena. In this case, Java caching technology emerged as an important solution. Java caching technology can help improve application performance, reduce access to the underlying database, shorten user waiting time, thereby improving user experience. This article will discuss how to use cache warming technology to further improve the performance of Java cache. What is Java cache? Caching is a common technique in software applications
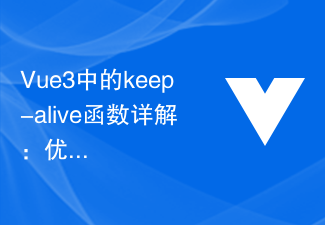
Detailed explanation of the keep-alive function in Vue3: Applications for optimizing application performance In Vue3, the keep-alive function becomes more powerful and can achieve more optimization functions. Through the keep-alive function, component status can be retained in memory to avoid repeated rendering of components and improve application performance and user experience. This article will introduce in detail the usage and optimization strategies of the keep-alive function in Vue3. 1. The keep-alive function is introduced in Vue3.

With the rapid development of the Internet industry, application performance and security have become the focus of increasing attention of enterprise developers. In the context of this demand, PHPHyperf microservice development technology has become a high-profile solution. This article will introduce practical techniques for PHPHyperf microservice development to improve application performance and security. 1. What is PHPHyperf microservice development? PHPHyperf is a high-performance coroutine framework developed based on Swoole extension. It has lightweight,
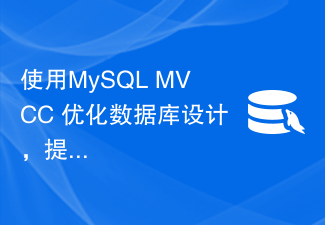
Use MySQLMVCC to optimize database design and improve application performance Summary: In today's Internet applications, database performance is crucial to the stable operation and response time of the system. As one of the most commonly used relational database management systems, MySQL uses multi-version concurrency control (MVCC) to improve concurrency performance and data consistency when designing the database. This article will introduce the basic principles of MVCC and its implementation in MySQL, and give some examples of optimizing database design. Basic principles of MVCC multi-version concurrency
