


How to dynamically update music classification lists through Vue and NetEase Cloud API
How to implement dynamic updating of music classification lists through Vue and NetEase Cloud API
Introduction:
In the modern music industry, dynamic updating of music classification lists is a very important function. Through Vue and NetEase Cloud API, we can easily implement this function. This article will show you how to use Vue and NetEase Cloud API to implement a dynamically updated music category list.
-
Preparation:
First, we need to introduce the axios library into the Vue project for sending HTTP requests. You can install axios through the following command:npm install axios
Copy after login
Then, we need to register a NetEase Cloud Music developer account and obtain the API access key.
- Create Vue component:
Create a Vue component named "MusicCategory" and declare some properties and methods that need to be used in this component. We need a data attribute to store the music category list and a method to request data from the NetEase Cloud API.
<template> <div> <ul> <li v-for="category in categories" :key="category.id">{{ category.name }}</li> </ul> </div> </template> <script> import axios from 'axios'; export default { data() { return { categories: [] }; }, mounted() { this.getMusicCategories(); }, methods: { getMusicCategories() { const apiUrl = '网易云API的地址/playlist/catlist'; const apiKey = '您的API访问密钥'; axios.get(apiUrl, { headers: { 'Content-Type': 'application/json', 'Authorization': apiKey } }) .then(response => { this.categories = response.data.categories; }) .catch(error => { console.log(error); }); } } }; </script>
In the above code, we sent a GET request to the NetEase Cloud API address/playlist/catlist through the axios library, and added the API access key in the request header. Then, we assign the returned data to the categories data attribute after a successful response. In the mounted life cycle hook function of the component, the getMusicCategories method is called to initialize the music category list.
- Using components:
Now, we can use the "MusicCategory" component anywhere in the Vue application. For example, we can introduce and use it in App.vue:
<template> <div id="app"> <h1>音乐分类列表</h1> <MusicCategory></MusicCategory> </div> </template> <script> import MusicCategory from './components/MusicCategory.vue'; export default { components: { MusicCategory } }; </script>
In the above code, we introduced the "MusicCategory" component in App.vue and use it as a subcomponent .
- Run the application:
Now, we can run the following command in the terminal to start the Vue application:
npm run serve
The Vue application will run on the default port 3000 . Visit http://localhost:3000 in the browser, you will see a page titled "Music Category List", and the page will display the music category list obtained from the NetEase Cloud API.
Summary:
Through Vue and NetEase Cloud API, we can easily implement the dynamic update function of music classification list. By using axios to send an HTTP request to obtain data, and saving the data in the data attribute of the Vue component, we can present a dynamically updated list of music categories on the page. This example shows how to use Vue and NetEase Cloud API to implement this function. I hope this article can help you quickly get started with Vue and use API to implement dynamic music classification lists.
The above is the detailed content of How to dynamically update music classification lists through Vue and NetEase Cloud API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


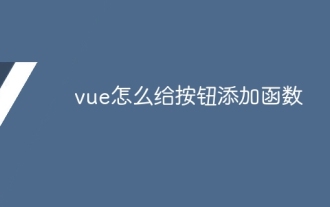
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
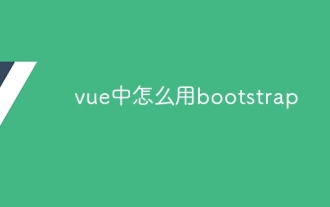
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
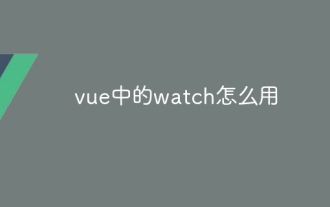
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
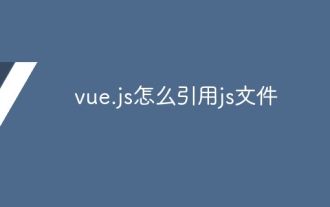
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
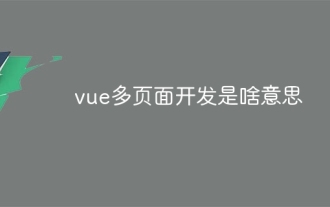
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
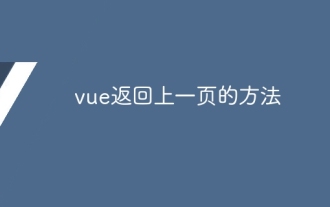
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
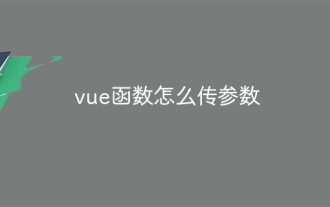
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
