How to customize SuiteCRM's sales team management through PHP
How to customize SuiteCRM’s sales team management through PHP
SuiteCRM is a powerful open source CRM system that provides a series of functions and tools to help companies effectively manage sales teams and improve sales performance. However, sometimes companies need to customize SuiteCRM according to their own business needs, especially sales team management functions.
In this article, we will explore how to customize SuiteCRM’s sales team management functions through PHP. We will use the API provided by SuiteCRM to perform data addition, deletion, modification and query operations, and combine some sample codes to illustrate the specific implementation method.
First, we need to understand the API of SuiteCRM. SuiteCRM provides two ways of data interaction: REST API and SOAP API. In this article, we will use REST API for data manipulation.
First, we need to create a new module in SuiteCRM to manage sales team information. We can use the module generator provided by SuiteCRM to create a new module, or manually add a new table directly in the database.
Next, we need to write some PHP code to achieve our needs. The following is a sample code that demonstrates how to create a sales team member through the REST API:
<?php // SuiteCRM的API URL $url = 'https://your-suitecrm-url.com/service/v4_1/rest.php'; // SuiteCRM的用户名和密码 $username = 'your-username'; $password = 'your-password'; // 销售团队的信息 $teamName = 'Sales Team'; $memberName = 'John Doe'; // 创建销售团队成员 $data = array( 'session' => '', 'module_name' => 'Teams', 'name_value_list' => array( array('name' => 'name', 'value' => $teamName), ), ); // 初始化cURL $curl = curl_init($url); // 设置cURL选项 curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'POST'); curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($data)); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Content-Length: ' . strlen(json_encode($data)), )); // 设置用户名和密码 curl_setopt($curl, CURLOPT_USERPWD, $username . ':' . $password); // 发送API请求 $response = curl_exec($curl); // 检查错误 if ($response === false) { die(curl_error($curl)); } // 解析API响应 $response = json_decode($response, true); // 检查是否创建成功 if ($response['id']) { // 创建成功 $teamId = $response['id']; // 将销售团队成员添加到团队中 $data = array( 'session' => '', 'module' => 'Teams', 'module_id' => $teamId, 'link_name' => 'team_members', 'related_id' => $memberId, ); curl_setopt($curl, CURLOPT_POSTFIELDS, http_build_query($data)); curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: application/x-www-form-urlencoded', 'Content-Length: ' . strlen(http_build_query($data)), )); // 发送API请求 $response = curl_exec($curl); // 检查错误 if ($response === false) { die(curl_error($curl)); } // 解析API响应 $response = json_decode($response, true); // 检查是否添加成功 if ($response['created'] == 1) { // 成功添加销售团队成员 echo 'Sales team member added successfully.'; } else { // 添加失败 echo 'Failed to add sales team member.'; } } else { // 创建失败 echo 'Failed to create sales team.'; } // 关闭cURL curl_close($curl); ?>
In the above code, we use the cURL library to send API requests and process and parse the API responses. We first created a sales team through the API, then created a sales team member and added it to the team.
In a similar way, we can use the API to perform operations such as query, edit, and deletion of the sales team to achieve our customized needs.
To sum up, customizing SuiteCRM’s sales team management functions through PHP is a relatively simple task. Through the API provided by SuiteCRM, we can easily perform data operations. In this process, we need to understand the API interface of SuiteCRM and write PHP code according to the specifications of the interface. I hope this article can help you realize the customized sales team management function of SuiteCRM.
The above is the detailed content of How to customize SuiteCRM's sales team management through PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


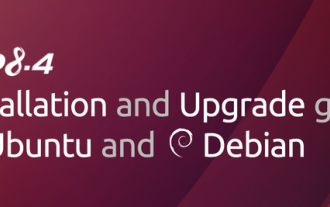
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
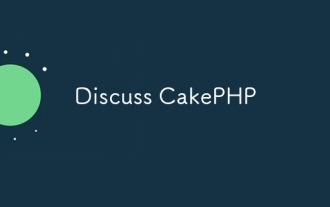
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
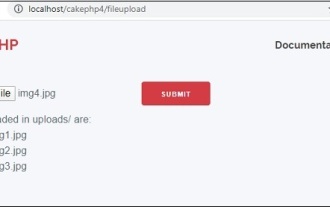
To work on file upload we are going to use the form helper. Here, is an example for file upload.
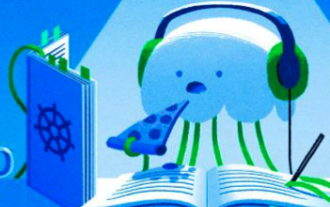
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
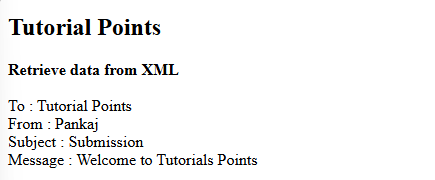
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
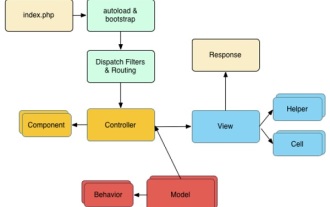
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
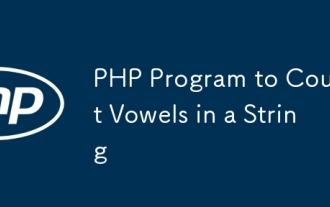
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
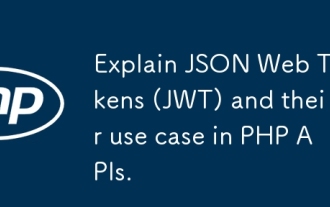
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
