


Vue and ECharts4Taro3 Advanced Tutorial: How to implement real-time charts with dynamic data updates
Vue and ECharts4Taro3 Advanced Tutorial: How to implement real-time charts with dynamic data updates
Introduction:
In modern front-end development, real-time charts are very important for data visualization. As a popular JavaScript framework, Vue provides concise and efficient data binding and component development capabilities. ECharts4Taro3 is a multi-terminal chart component library based on Taro3 and ECharts4 packages. This article will introduce how to use Vue and ECharts4Taro3 to implement real-time charts with dynamic data updates, and provide relevant code examples.
1. Preparation
Before starting, you need to ensure that the Vue CLI, Taro CLI and ECharts4Taro3 environment have been installed. First, use the following command to globally install Vue CLI and Taro CLI:
npm install -g @vue/cli npm install -g @tarojs/cli
Then, create a new Vue project, enter the project directory and install ECharts4Taro3:
vue create my-project cd my-project npm install echarts4taro3 --save
After the installation is complete, you can start writing Code for live charts.
2. Create a real-time chart component
First, create a component file named RealTimeChart.vue in the src/components directory. Then, introduce the necessary libraries and components into the component:
<template> <taro-echarts :ec="ec" /> </template> <script> import { ref, onMounted, onUnmounted } from 'vue' import TaroECharts from 'echarts4taro3' import moment from 'moment' export default { setup() { const ec = ref(null) const chart = ref(null) onMounted(() => { chart.value = TaroECharts.init(ec.value) // 初始化数据 const initOption = { // 配置初始化选项 } chart.value.setOption(initOption) // 开始定时更新数据 setInterval(() => { // 更新数据 const newData = { // 获取新的数据 } chart.value.setOption({ series: [{ data: newData }] }) }, 1000) }) onUnmounted(() => { chart.value.dispose() }) return { ec } } } </script>
In the above code, various necessary libraries and components in Vue are first imported, including ref, onMounted, onUnmounted, etc. Then, the necessary variables and references are created in the setup function. In the onMounted life cycle function, TaroECharts is used to initialize the chart, and the initialization option initOption is defined. Then, update the data every second via a timer and pass the new data to the real-time chart using the setOption method. Finally, clear the chart binding in the onUnmounted lifecycle function.
3. Use the real-time chart component in the main page
Create a page component named Home.vue in the src/views directory to display real-time charts. Introduce the RealTimeChart component into Home.vue:
<template> <div class="home"> <RealTimeChart /> </div> </template> <script> import RealTimeChart from '@/components/RealTimeChart.vue' export default { components: { RealTimeChart }, // ... } </script>
In the above code, introduce the RealTimeChart component through import and register it in the components section. Then, use components in the template to display real-time charts.
4. Compile and run the project
Next, use Taro CLI to compile and run the project. Run the following command in the project root directory:
taro build --watch
Then, run the following command in another terminal window to start the project:
taro dev:h5
At this time, the browser will automatically open and display Home .vue page. Real-time charts will be rendered on the page, with data refreshed every second.
Summary:
Through this article, we learned how to use Vue and ECharts4Taro3 to create real-time charts with dynamic data updates. First, we prepared the necessary environment and created the Vue project. Then, we created the RealTimeChart component and used Taro ECharts and Vue's related APIs to implement regular data updates. Finally, we introduced the RealTimeChart component into the main page and compiled and ran the project. I hope this article can help you understand and learn Vue and ECharts4Taro3.
Code sample reference: https://github.com/your-username/your-repo
The above is the detailed content of Vue and ECharts4Taro3 Advanced Tutorial: How to implement real-time charts with dynamic data updates. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


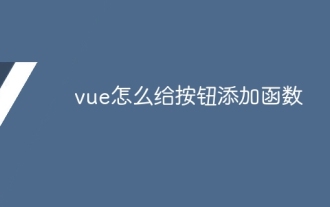
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
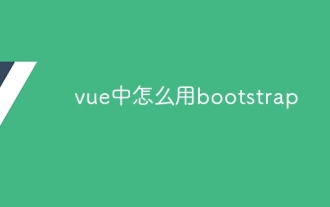
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
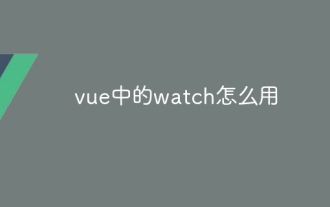
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
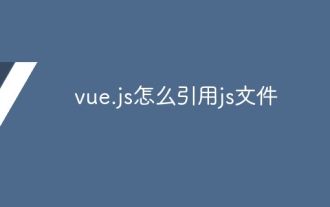
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
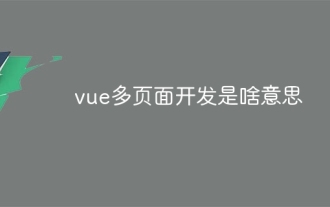
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
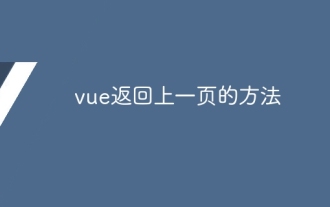
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
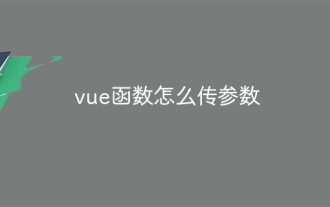
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
