


How to use routing in Vue to implement data transfer and state management between pages?
How to use routing to implement data transfer and state management between pages in Vue?
In Vue, routing (Router) is one of the core plug-ins for switching between pages. In addition to page jumps, routing can also be used to implement data transfer and status management. This article will introduce how to use Vue's routing plug-in (Vue Router) to realize data transfer and status management between pages, and provide corresponding code examples.
- Basic use of routing
Vue Router is the official routing plug-in of Vue.js, which can realize routing management of single-page applications. First, we need to install Vue Router using npm and introduce the corresponding modules.
// main.js import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) // 声明路由配置项 const routes = [ { path: '/page1', component: Page1 }, { path: '/page2', component: Page2 }, // ... ] // 创建路由实例 const router = new VueRouter({ routes }) // 创建Vue实例,并将路由实例注入 new Vue({ router, render: h => h(App) }).$mount('#app')
In the above code, first install the Vue Router plug-in through Vue.use(VueRouter)
. Then, declare the routing configuration items, where path
represents the path of the page, and component
represents the corresponding component. Finally, create a routing instance through new VueRouter()
and inject it into the Vue instance.
- Data transfer
To transfer data between pages, you can use routing parameters (params) or query parameters (query).
Use params to pass data:
// 跳转到page2页面时传递参数 router.push({ path: '/page2', params: { id: 1 } }) // 在page2页面中接收参数 export default { mounted() { console.log(this.$route.params.id) // 输出1 } }
Use query to pass data:
// 跳转到page2页面时传递参数 router.push({ path: '/page2', query: { id: 1 } }) // 在page2页面中接收参数 export default { mounted() { console.log(this.$route.query.id) // 输出1 } }
- State management
In addition to data transmission , routing can also be used to implement simple state management. In Vue Router, this can be achieved by adding the meta field to the routing configuration item.
// 声明路由配置项 const routes = [ { path: '/page1', component: Page1 }, { path: '/page2', component: Page2, meta: { requiresAuth: true } // 添加meta字段 }, // ... ] // 路由守卫 router.beforeEach((to, from, next) => { if (to.matched.some(record => record.meta.requiresAuth)) { // 判断是否需要认证 if (!isAuthenticated()) { // 未认证,跳转到登录页 next({ path: '/login', query: { redirect: to.fullPath } }) } else { // 已认证,继续跳转 next() } } else { next() } })
In the above code, we added the meta: { requiresAuth: true }
field to the routing configuration item of Page2, indicating that the page requires authentication. In the beforeEach hook function of the route, we make the authentication judgment. If the page requires authentication and is not authenticated, it will jump to the login page; if it has been authenticated or the page does not require authentication, it will continue to jump.
In this way, we can use Vue's routing plug-in to realize data transfer and status management between pages. Data can be passed to the target page through params and query and accessed in the target page. Simple status management can be achieved by adding meta fields to routing configuration items.
Summary
By using Vue Router, a powerful plug-in, we can easily implement routing management for single-page applications. By rationally utilizing routing parameters and query parameters, data transfer between pages can be achieved. At the same time, simple status management can be achieved by adding meta fields in routing configuration items. This provides us with convenience and flexibility in developing Vue applications.
The above is the detailed content of How to use routing in Vue to implement data transfer and state management between pages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


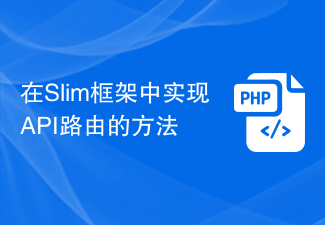
How to implement API routing in the Slim framework Slim is a lightweight PHP micro-framework that provides a simple and flexible way to build web applications. One of the main features is the implementation of API routing, allowing us to map different requests to corresponding handlers. This article will introduce how to implement API routing in the Slim framework and provide some code examples. First, we need to install the Slim framework. The latest version of Slim can be installed through Composer. Open a terminal and
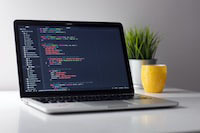
Apache Camel is an Enterprise Service Bus (ESB)-based integration framework that can easily integrate disparate applications, services, and data sources to automate complex business processes. ApacheCamel uses route-based configuration to easily define and manage integration processes. Key features of ApacheCamel include: Flexibility: ApacheCamel can be easily integrated with a variety of applications, services, and data sources. It supports multiple protocols, including HTTP, JMS, SOAP, FTP, etc. Efficiency: ApacheCamel is very efficient, it can handle a large number of messages. It uses an asynchronous messaging mechanism, which improves performance. Expandable
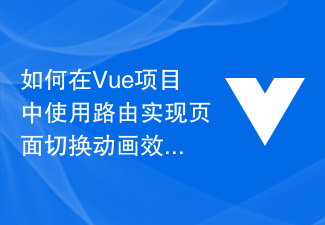
How to use routing to customize page switching animation effects in a Vue project? Introduction: In the Vue project, routing is one of the functions we often use. Switching between pages can be achieved through routing, providing a good user experience. In order to make page switching more vivid, we can achieve it by customizing animation effects. This article will introduce how to use routing to customize the page switching animation effect in the Vue project. Create a Vue project First, we need to create a Vue project. You can use VueCLI to quickly build
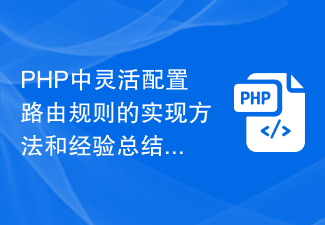
Implementation method and experience summary of flexible configuration of routing rules in PHP Introduction: In Web development, routing rules are a very important part, which determines the corresponding relationship between URL and specific PHP scripts. In the traditional development method, we usually configure various URL rules in the routing file, and then map the URL to the corresponding script path. However, as the complexity of the project increases and business requirements change, it will become very cumbersome and inflexible if each URL needs to be configured manually. So, how to implement in PHP
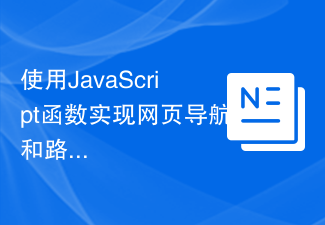
In modern web applications, implementing web page navigation and routing is a very important part. Using JavaScript functions to implement this function can make our web applications more flexible, scalable and user-friendly. This article will introduce how to use JavaScript functions to implement web page navigation and routing, and provide specific code examples. Implementing web page navigation For a web application, web page navigation is the most frequently operated part by users. When a user clicks on the page
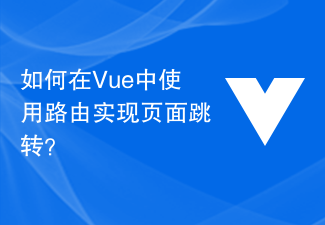
How to use routing to implement page jump in Vue? With the continuous development of front-end development technology, Vue.js has become one of the most popular front-end frameworks. In Vue development, page jump is an essential part. Vue provides VueRouter to manage application routing, and seamless switching between pages can be achieved through routing. This article will introduce how to use routing to implement page jumps in Vue, with code examples. First, install the vue-router plugin in the Vue project.
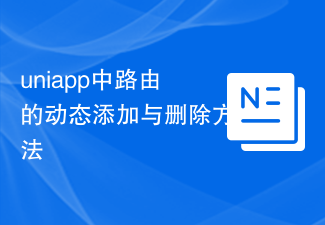
Uniapp is a cross-end framework based on Vue.js. It supports one-time writing and generates multi-end applications such as H5, mini programs, and APPs at the same time. It pays great attention to performance and development efficiency during the development process. In Uniapp, the dynamic addition and deletion of routes is a problem that is often encountered during the development process. Therefore, this article will introduce the dynamic addition and deletion of routes in Uniapp and provide specific code examples. 1. Dynamic addition of routes Dynamic addition of routes can be done according to actual needs when the page is loaded or after user operation.
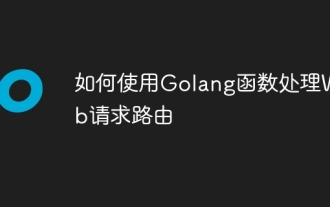
In Golang, using functions to handle web request routing is an extensible and modular method of building APIs. It involves the following steps: Install the HTTP router library. Create a router. Define path patterns and handler functions for routes. Write handler functions to handle requests and return responses. Run the router using an HTTP server. This process allows for a modular approach when handling incoming requests, improving reusability, maintainability, and testability.
