How to use keep-alive in vue to improve front-end development efficiency
How to use keep-alive in Vue to improve front-end development efficiency
The performance of front-end development has always been one of the focuses of developers. In order to improve user experience and page loading speed, we often have to consider how to optimize front-end rendering. As a popular front-end framework, Vue provides keep-alive components to solve the performance problems of inactive components. This article will introduce the use of keep-alive and show how it can improve front-end development efficiency in Vue through code examples.
- The role and principle of keep-alive
In Vue, the destruction and re-creation of components is a time-consuming process. If we have some inactive components that are not used frequently between switches or page views, but are recreated every time we switch, it will lead to performance degradation. The keep-alive component can cache instances of these inactive components, thereby avoiding unnecessary destruction and re-creation and improving rendering performance.
In principle, keep-alive caches the virtual DOM of inactive components in memory and directly restores the cached instance when the component switches without re-creating it. This can reduce the time and overhead of page rendering, thereby improving the efficiency of front-end development.
- Using keep-alive
Using keep-alive in Vue is very simple, just wrap the component you want to cache outside the component. Here is an example:
<template> <div> <h1>首页</h1> <keep-alive> <router-view></router-view> </keep-alive> </div> </template> <script> export default { name: 'App', } </script>
In the above code, we used the <keep-alive>
tag outside the <router-view>
tag, which means We will cache instances of <router-view>
.
- Keep-alive properties
In addition to the basic usage methods, keep-alive also provides some properties that can more flexibly control cached components.
include
: Used to specify the name of the component to be cached, supporting strings or regular expressions. For example,include="Home,About"
means that only components named "Home" and "About" will be cached.exclude
: Used to specify component names that do not need to be cached. It also supports strings or regular expressions. For example,exclude="Login,Register"
means that components named "Login" and "Register" are not cached.max
: Used to specify the maximum number of components to be cached. When the number of cached components exceeds the limit, the oldest cached component will be destroyed. For example,max="10"
means that the maximum cache is 10 components.
Here is an example that demonstrates how to use the include
and exclude
attributes:
<template> <div> <h1>首页</h1> <keep-alive :include="['Home', 'About']" :exclude="['Login', 'Register']"> <router-view></router-view> </keep-alive> </div> </template> <script> export default { name: 'App', } </script>
- keep-alive life cycle
keep-alive itself also has life cycle hook functions, which can be used to monitor the status changes of cache components. The main life cycle hook functions are:
activated
: called when the cache component is activated, usually triggered when the component enters the cache for the first time or is restored from the cache.deactivated
: Called when the cache component is deactivated, usually triggered when the component leaves the cache or is destroyed from the cache.
Here is an example showing how to use the activated
and deactivated
hook functions:
<template> <div> <h1>首页</h1> <keep-alive :include="['Home', 'About']" @activated="onActivated" @deactivated="onDeactivated"> <router-view></router-view> </keep-alive> </div> </template> <script> export default { name: 'App', methods: { onActivated() { console.log('组件被激活') }, onDeactivated() { console.log('组件被停用') }, }, } </script>
By listening to these life cycle hook functions, We can handle some specific events, such as performing certain actions when the cache component is reactivated.
Summary:
Using keep-alive components is an effective way to optimize Vue application performance. By caching instances of inactive components, we can avoid unnecessary destruction and re-creation, thereby improving front-end development efficiency. Reasonable use of keep-alive in the application, combined with related attributes and life cycle hook functions, can better optimize page rendering and user experience.
I hope this article can help everyone understand and use keep-alive in Vue.
The above is the detailed content of How to use keep-alive in vue to improve front-end development efficiency. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


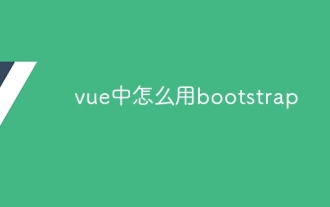
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
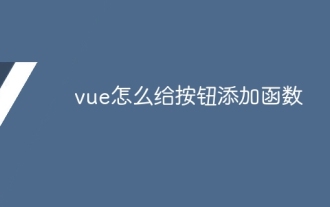
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
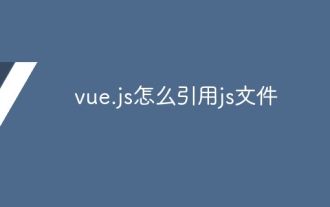
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
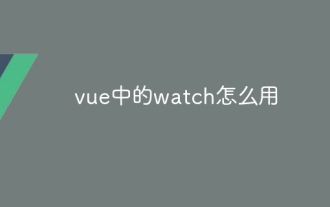
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
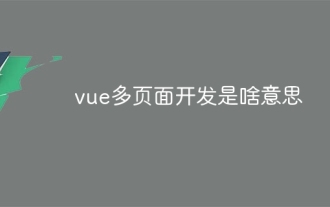
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
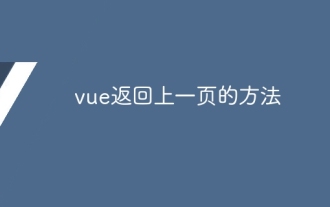
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
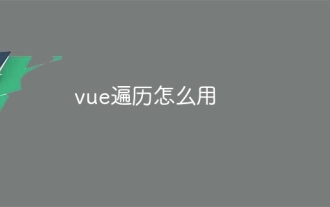
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
