


How does PHP ZipArchive implement PDF compression function for files in compressed packages?
How does PHP ZipArchive implement PDF compression function for files in compressed packages?
Introduction:
In modern society, electronic documents have become an indispensable part of our daily life and work. Among them, PDF format documents are widely used in various scenarios because of their cross-platform, easy-to-read, and content-protected characteristics. However, as the number of documents increases, the size of PDF documents also gradually increases. In some cases, multiple PDF files need to be packaged into a compressed package and compressed to reduce the file size. This article will introduce how to use PHP's ZipArchive library to compress PDF files in compressed packages.
1. Install ZipArchive library
Before using ZipArchive, you need to ensure that PHP has installed the corresponding library. You can install it by following these steps:
- Open a terminal or command line tool.
-
Run the following command to install the ZipArchive library:
$ sudo apt-get install php-zip
Copy after login - After the installation is complete, restart the PHP server.
2. Create a compressed package and add PDF files
The following is a simple sample code for creating a compressed package and adding PDF files to it. Assume that we already have a folder containing multiple PDF files. We need to package these PDF files into a compressed package and compress it.
<?php // 创建一个压缩包 $zip = new ZipArchive; $zipFilename = 'compressed.zip'; if ($zip->open($zipFilename, ZipArchive::CREATE) === TRUE) { // 需要压缩的PDF文件所在的文件夹路径 $pdfFolder = '/path/to/pdf/folder'; // 遍历文件夹中的PDF文件,并添加到压缩包 if ($handle = opendir($pdfFolder)) { while (false !== ($file = readdir($handle))) { if ($file != "." && $file != "..") { $pdfPath = $pdfFolder . '/' . $file; // 只处理PDF文件 if(pathinfo($pdfPath, PATHINFO_EXTENSION) === 'pdf') { // 将PDF文件添加到压缩包中 $zip->addFile($pdfPath, $file); } } } closedir($handle); } // 关闭压缩包 $zip->close(); echo '压缩包已创建成功,文件名为:' . $zipFilename; } else { echo '创建压缩包失败'; } ?>
3. Use ZipArchive to compress PDF files
The following is a sample code for compressing PDF files in compressed packages through the ZipArchive library. By using the PHP ZipArchive library, we can read, compress each PDF file, and write the compressed content into a new compressed archive file.
<?php // 打开一个已有的压缩包 $zip = new ZipArchive; $zipFilename = 'compressed.zip'; if ($zip->open($zipFilename, ZipArchive::CREATE) === TRUE) { // 遍历压缩包中的文件 for ($i = 0; $i < $zip->numFiles; $i++) { // 获取压缩包中的文件名 $filename = $zip->getNameIndex($i); // 检查文件是否为PDF文件 if (pathinfo($filename, PATHINFO_EXTENSION) === 'pdf') { // 获取文件内容 $fileContent = $zip->getFromIndex($i); // 对文件内容进行压缩处理 $compressedContent = compressPDF($fileContent); // 将压缩后的内容写入到压缩包中 $zip->deleteIndex($i); $zip->addFromString($filename, $compressedContent); } } // 关闭压缩包 $zip->close(); echo '压缩完成'; } else { echo '打开压缩包失败'; } function compressPDF($content) { // 实现PDF文件压缩逻辑,此处省略具体实现步骤 // 返回压缩后的内容 return $compressedContent; } ?>
Summary:
By using the PHP ZipArchive library, we can easily implement the compression function of PDF files in the compressed package. We can create a compressed package and add PDF files to it; we can also read PDF files in existing compressed packages and compress them. In the specific compression logic implementation, we can use a third-party PDF processing library, such as Ghostscript, to compress PDF files.
The above is the detailed content of How does PHP ZipArchive implement PDF compression function for files in compressed packages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


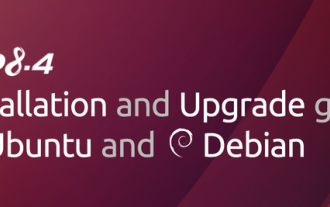
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
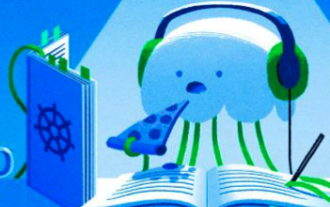
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
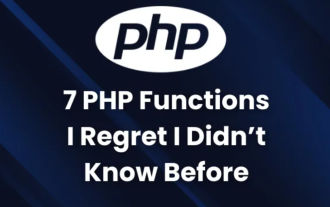
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
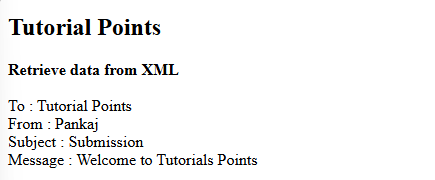
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
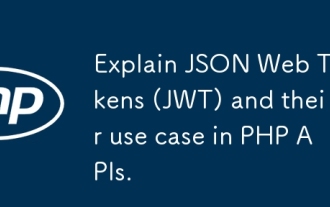
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
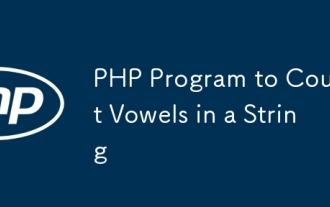
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
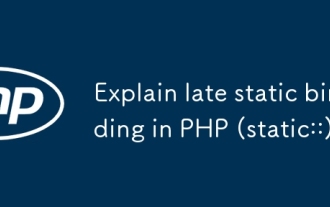
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
