


How to use Goroutines to implement elegant concurrent programming patterns
How to use Goroutines to implement elegant concurrent programming patterns
In modern software development, faced with processing a large number of concurrent tasks, we often need to use concurrent programming patterns to improve the efficiency and responsiveness of the program. Goroutines in the Go language provide us with an elegant concurrent programming method. This article will introduce how to use Goroutines to implement elegant concurrent programming patterns, accompanied by code examples.
Goroutines are a lightweight thread in the Go language. Multiple Goroutines can be created in the program, and each Goroutines can run in an independent execution environment. Goroutines are managed by the runtime of the Go language, which can be automatically scheduled and managed, allowing us to focus more on writing business logic.
To use Goroutines to implement concurrent programming, we first need to understand how to create and start a Goroutines. In the Go language, we can use the keyword "go" plus a function call to create a Goroutines and start its execution. Here is a simple example:
package main import ( "fmt" "time" ) func main() { go hello() time.Sleep(time.Second) } func hello() { fmt.Println("Hello, Goroutine!") }
In the above example, we called go hello()
to create a Goroutines and start its execution. In the main function, we also use time.Sleep(time.Second)
to wait for one second to ensure that the program can end normally.
In actual concurrent programming, we usually face the situation of multiple Goroutines accessing shared resources at the same time. In this case, we need to use a mutex (Mutex) to protect access to shared resources. Here is an example of using a mutex for thread-safe access:
package main import ( "fmt" "sync" "time" ) var mutex sync.Mutex var count int func main() { for i := 0; i < 10; i++ { go increment() } time.Sleep(time.Second) } func increment() { mutex.Lock() defer mutex.Unlock() count++ fmt.Println("Count:", count) }
In the above example, we create a mutex using sync.Mutex
and then Use
mutex.Lock() and
mutex.Unlock() in the increment
function to protect access to the count variable. In the main function, we create multiple Goroutines and call the increment function at the same time to increase the value of the count variable. Through the protection of the mutex lock, the thread safety of count is ensured.
In addition to mutex locks, the Go language also provides other concurrency primitives, such as condition variables, read-write locks, etc., which can be selected according to actual needs.
In addition, communication between Goroutines is another important aspect of implementing concurrent programming. In the Go language, we can use channels to implement data transfer and synchronization between Goroutines. The following is an example of using channels for data transfer:
package main import ( "fmt" "time" ) func main() { ch := make(chan int) go producer(ch) go consumer(ch) time.Sleep(time.Second) } func producer(ch chan<- int) { for i := 0; i < 10; i++ { ch <- i time.Sleep(time.Millisecond * 500) } close(ch) } func consumer(ch <-chan int) { for i := range ch { fmt.Println("Received:", i) } }
In the above example, we create a channel ch
, and then pass it to the channel in the producer
function Send data in, use the consumer
function to receive data from the channel and print it. Through the sending and receiving operations of the channel, data transmission and synchronization between Goroutines are realized.
In addition to the mutex locks and channels in the above examples, the Go language also provides many rich concurrent programming tools and libraries, such as atomic operations, timers, concurrency-safe data structures, etc., which can be implemented according to actual needs. Select.
In summary, by using Goroutines and related concurrency primitives, we can implement elegant concurrent programming patterns and improve program performance and responsiveness. However, it should be noted that for concurrent programming, special attention needs to be paid to handling race conditions and resource contention issues to avoid introducing potential concurrency security issues. When writing concurrent code, it is recommended to conduct careful design and testing to ensure the correctness and stability of the program.
References:
- Go Concurrency Patterns: https://talks.golang.org/2012/concurrency.slide
- Go by Example: Goroutines: https ://gobyexample.com/goroutines
- Go Concurrency Patterns: Timing out, moving on: https://blog.golang.org/concurrency-timeouts
The above is the detailed content of How to use Goroutines to implement elegant concurrent programming patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
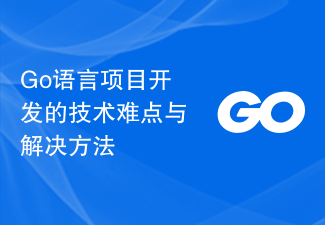
Technical Difficulties and Solutions in Go Language Project Development With the popularization of the Internet and the development of informatization, the development of software projects has received more and more attention. Among many programming languages, Go language has become the first choice of many developers because of its powerful performance, efficient concurrency capabilities and simple and easy-to-learn syntax. However, there are still some technical difficulties in the development of Go language projects. This article will explore these difficulties and provide corresponding solutions. 1. Concurrency control and race conditions The concurrency model of Go language is called "goroutine", which makes
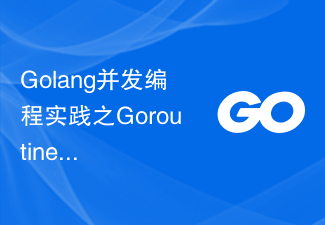
Introduction to the application scenario analysis of Goroutines in Golang concurrent programming practice: With the continuous improvement of computer performance, multi-core processors have become mainstream. In order to make full use of the advantages of multi-core processors, we need to use concurrent programming technology to implement multi-threaded operations. In the Go language, Goroutines (coroutines) are a very powerful concurrent programming mechanism that can be used to achieve efficient concurrent operations. In this article, we will explore the application scenarios of Goroutines and give some examples.
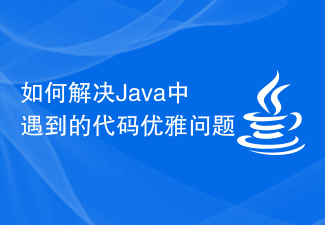
How to solve code elegance problems encountered in Java Code elegance is the goal pursued by every programmer. An elegant code can not only improve the readability and maintainability of the code, but also improve development efficiency and code quality. In Java development, we often encounter some code elegance problems. This article will explore how to solve these problems. Reasonable use of naming conventions Naming is very important when writing Java code. Reasonable naming conventions can make code more readable and better express the intent of the code. we can follow
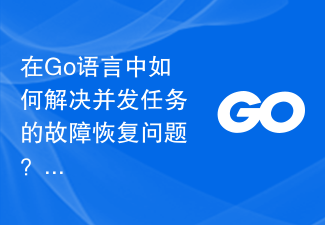
How to solve the problem of failure recovery of concurrent tasks in Go language? In modern software development, the use of concurrent processing can significantly improve the performance of the program. In the Go language, we can achieve efficient concurrent task processing by using goroutine and channels. However, concurrent tasks also bring some new challenges, such as handling failure recovery. This article will introduce some methods to solve the problem of concurrent task failure recovery in Go language and provide specific code examples. Error handling in concurrent tasks When processing concurrent tasks,
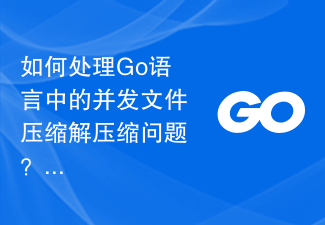
How to deal with concurrent file compression and decompression in Go language? File compression and decompression is one of the tasks frequently encountered in daily development. As file sizes increase, compression and decompression operations can become time-consuming, so concurrency becomes an important means of improving efficiency. In the Go language, you can use the features of goroutine and channel to implement concurrent processing of file compression and decompression operations. File compression First, let's take a look at how to implement file compression in the Go language. Go language standard
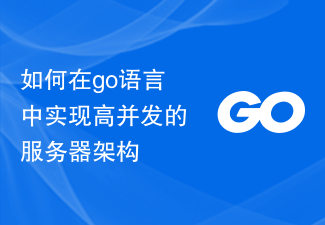
How to implement high-concurrency server architecture in Go language Introduction: In today's Internet era, the concurrent processing capability of the server is one of the important indicators to measure the performance of a system. Servers with high concurrency capabilities can handle a large number of requests, maintain system stability, and provide fast response times. In this article, we will introduce how to implement a highly concurrent server architecture in the Go language, including concepts, design principles, and code examples. 1. Understand the concepts of concurrency and parallelism. Before starting, let’s sort out the concepts of concurrency and parallelism. Concurrency refers to multiple
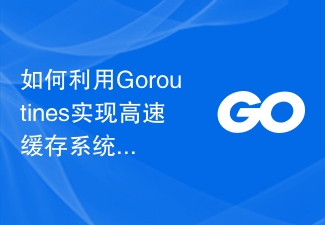
How to use Goroutines to implement a caching system. In modern software development, caching is one of the common ways to improve system performance. Using Goroutines to implement a cache system can improve the system's response speed and throughput without blocking the main thread. This article will introduce how to use Goroutines to create a simple and efficient caching system and provide corresponding code examples. 1. What are Goroutines? Goroutines is a lightweight tool provided by the Go language.
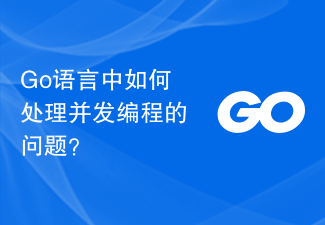
How to deal with concurrent programming issues in Go language? In today's software development, multitasking has become the norm. Concurrent programming can not only improve the efficiency of the program, but also make better use of computing resources. However, concurrent programming also introduces some problems, such as race conditions, deadlocks, etc. As an advanced programming language, Go language provides some powerful mechanisms and tools to deal with concurrent programming issues. GoroutineGoroutine is one of the core mechanisms for handling concurrency in the Go language. Gorout
