How to use context to implement request retry strategy in Go
How to use context to implement request retry strategy in Go
Introduction:
When building a distributed system, network requests will inevitably encounter some failures. In order to ensure the reliability and stability of the system, we usually use a retry strategy to handle these failed requests to increase the success rate of the request. In the Go language, we can use the context package to implement the request retry strategy. This article will introduce how to use the context package in Go to implement a request retry strategy, with code examples.
1. What is context package?
The context package is a standard package provided by the Go language for processing request context information. Through the context package, we can pass the context information of the request during the request processing process, and control the cancellation, timeout and deadline of the request. In addition to these basic functions, the context package can also be used to implement request retry strategies.
2. Implementation of retry strategy
To implement the request retry strategy in Go, we usually use a for loop to try multiple requests until the request succeeds or the maximum retry is reached. number of attempts. In each request, we can use the context's timeout or deadline to control the time limit of each request. The following is a sample code:
package main import ( "context" "errors" "fmt" "net/http" "time" ) func main() { url := "http://example.com/api" maxRetries := 3 err := retryRequest(context.Background(), url, maxRetries) if err != nil { fmt.Println("Request failed:", err) } else { fmt.Println("Request succeeded!") } } func retryRequest(ctx context.Context, url string, maxRetries int) error { for i := 0; i < maxRetries; i++ { err := makeRequest(ctx, url) if err == nil { return nil } fmt.Println("Request failed:", err) } return errors.New("Request failed after maximum retries") } func makeRequest(ctx context.Context, url string) error { req, err := http.NewRequest("GET", url, nil) if err != nil { return err } ctx, cancel := context.WithTimeout(ctx, time.Second*5) defer cancel() req = req.WithContext(ctx) resp, err := http.DefaultClient.Do(req) if err != nil { return err } defer resp.Body.Close() if resp.StatusCode != http.StatusOK { return errors.New("Response status code is not OK") } return nil }
In the above code example, we first define the URL that needs to be requested and the maximum number of retries. Then implement the request retry strategy through the retryRequest
function, which will retry each time the request fails until the request succeeds or the maximum number of retries is reached. In each request, we use the makeRequest
function to send the HTTP request. By calling the context.WithTimeout
method, we set the timeout for each request to 5 seconds.
Through the above code examples, we can flexibly adjust the number of retries, timeout time and specific request logic to meet the needs of different scenarios.
Conclusion:
By using the context package, we can easily implement the request retry strategy. With the functions provided by the context package, we can control the timeout, deadline, and cancellation of requests, etc. This not only increases system reliability and stability, but also provides a better user experience. I hope this article can help you implement request retry strategy in Go language.
The above is the detailed content of How to use context to implement request retry strategy in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


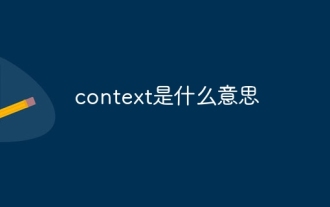
Context is the environment and status information when the program is executed. It can include a variety of information, such as the value of variables, the call stack of functions, the execution location of the program, etc., allowing the program to make corresponding decisions based on different contexts. and perform corresponding operations.
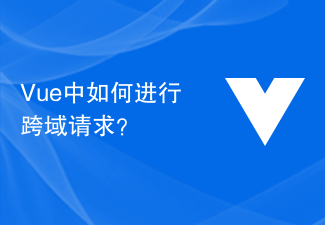
Vue is a popular JavaScript framework for building modern web applications. When developing applications using Vue, you often need to interact with different APIs, which are often located on different servers. Due to cross-domain security policy restrictions, when a Vue application is running on one domain name, it cannot communicate directly with the API on another domain name. This article will introduce several methods for making cross-domain requests in Vue. 1. Use a proxy A common cross-domain solution is to use a proxy
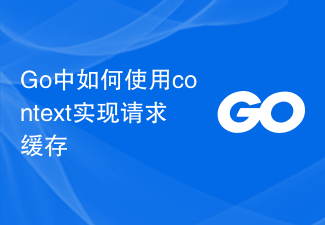
How to use context to implement request caching in Go Introduction: When building web applications, we often need to cache requests to improve performance. In the Go language, we can use the context package to implement the request caching function. This article will introduce how to use the context package to implement request caching, and provide code examples to help readers better understand. What is context? : In the Go language, the context package provides a way to pass between multiple goroutines
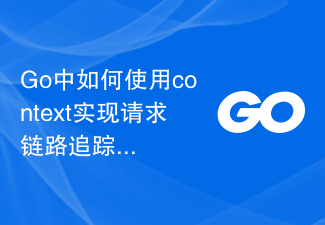
How to use context to implement request link tracking in Go. In the microservice architecture, request link tracking is a very important technology that is used to track the delivery and processing of a request between multiple microservices. In the Go language, we can use the context package to implement request link tracking. This article will introduce how to use context for request link tracking and give code examples. First, we need to understand the basic concepts and usage of the context package. The context package provides a mechanism
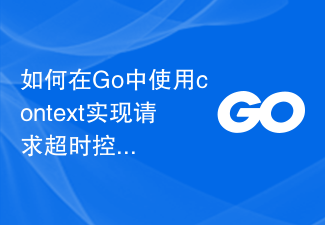
How to use context to implement request timeout control in Go Introduction: When we make network requests, we often encounter request timeout problems. A network request that does not respond for a long time will not only waste server resources, but also affect overall performance. In order to solve this problem, the Go language introduced the context package, which can be used to implement request timeout control. This article will introduce how to use the context package to implement request timeout control in Go, and attach corresponding code examples. 1. Understand the context package co
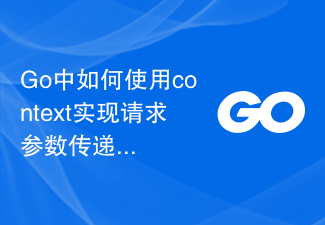
The context package in the Go language is used to pass request context information in the program. It can pass parameters, intercept requests and cancel operations between functions across multiple Goroutines. To use the context package in Go, we first need to import the "context" package. Below is an example that demonstrates how to use the context package to implement request parameter passing. packagemainimport("context"
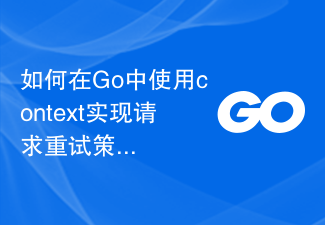
How to use context to implement request retry strategy in Go Introduction: When building a distributed system, network requests will inevitably encounter some failures. In order to ensure the reliability and stability of the system, we usually use a retry strategy to handle these failed requests to increase the success rate of the request. In the Go language, we can use the context package to implement the request retry strategy. This article will introduce how to use the context package in Go to implement a request retry strategy, with code examples. 1. What is
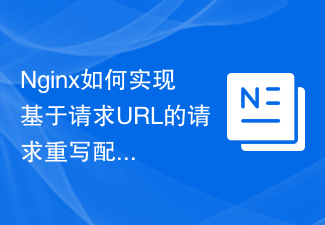
Nginx is a lightweight, high-performance web server that not only supports advanced functions such as reverse proxy and load balancing, but also has powerful request rewriting capabilities. In actual web applications, in many cases the request URL needs to be rewritten to achieve better user experience and search engine optimization effects. This article will introduce how Nginx implements request rewriting configuration based on the request URL, including specific code examples. Rewrite syntax In Nginx, you can use the rewrite directive to perform request rewriting. its basic language
