


Vue and Canvas: How to implement multi-level graphics drawing and operations
Vue and Canvas: How to implement multi-level graphics drawing and manipulation
Introduction:
In modern web development, Vue.js, as a popular JavaScript framework, is often used to build interactive front-end application. Canvas is a newly added element in HTML5, which provides a method to draw graphics through scripts. This article will introduce how to use Canvas in Vue.js to achieve multi-level graphics drawing and operations.
- Create Vue component:
First, we need to create a Vue component to display Canvas on the page. In the component, we need to define a Canvas element and assign it a unique id.
<template> <div> <canvas :id="canvasId"></canvas> </div> </template> <script> export default { data() { return { canvasId: 'myCanvas' // 指定Canvas的id } }, mounted() { this.initCanvas() }, methods: { initCanvas() { const canvas = document.getElementById(this.canvasId) this.context = canvas.getContext('2d') } } } </script>
- Draw the underlying graphics:
Next, we can draw the underlying graphics in Canvas. For example, we can draw a rectangle and fill it with a red background.
methods: { initCanvas() { const canvas = document.getElementById(this.canvasId) this.context = canvas.getContext('2d') // 绘制底层图形 this.context.fillStyle = 'red' this.context.fillRect(0, 0, canvas.width, canvas.height) } }
- Drawing upper-layer graphics:
To draw upper-layer graphics in Canvas, we can use the mouse or other interactive events to trigger. For example, when the user clicks on the Canvas, we can draw a circle.
methods: { initCanvas() { const canvas = document.getElementById(this.canvasId) this.context = canvas.getContext('2d') // 绘制底层图形 this.context.fillStyle = 'red' this.context.fillRect(0, 0, canvas.width, canvas.height) // 绑定事件监听器 canvas.addEventListener('click', this.drawCircle) }, drawCircle(event) { const canvas = document.getElementById(this.canvasId) const rect = canvas.getBoundingClientRect() const x = event.clientX - rect.left const y = event.clientY - rect.top // 绘制圆形 this.context.beginPath() this.context.arc(x, y, 50, 0, 2 * Math.PI) this.context.fillStyle = 'blue' this.context.fill() } }
- Clear Canvas:
In some cases, we may need to clear all graphics on Canvas. To achieve this, we can add a clear button, and when the user clicks the button, we can clear the content on the Canvas.
<template> <div> <canvas :id="canvasId"></canvas> <button @click="clearCanvas">清空</button> </div> </template> <script> export default { data() { return { canvasId: 'myCanvas' // 指定Canvas的id } }, mounted() { this.initCanvas() }, methods: { initCanvas() { const canvas = document.getElementById(this.canvasId) this.context = canvas.getContext('2d') // 绘制底层图形 this.context.fillStyle = 'red' this.context.fillRect(0, 0, canvas.width, canvas.height) // 绑定事件监听器 canvas.addEventListener('click', this.drawCircle) }, drawCircle(event) { const canvas = document.getElementById(this.canvasId) const rect = canvas.getBoundingClientRect() const x = event.clientX - rect.left const y = event.clientY - rect.top // 绘制圆形 this.context.beginPath() this.context.arc(x, y, 50, 0, 2 * Math.PI) this.context.fillStyle = 'blue' this.context.fill() }, clearCanvas() { const canvas = document.getElementById(this.canvasId) this.context.clearRect(0, 0, canvas.width, canvas.height) } } } </script>
Conclusion:
Through the above steps, we have learned how to use Canvas to achieve multi-level graphics drawing and operations in Vue.js. We can use Canvas to draw the underlying graphics, and then draw the upper-level graphics through interactive events. We can also clear the content on the Canvas. This provides us with a simple and effective way to create interactive graphics in web applications.
The above is the detailed content of Vue and Canvas: How to implement multi-level graphics drawing and operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
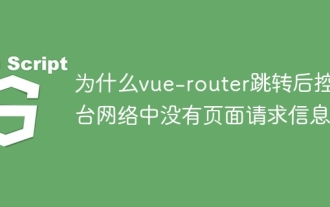
Why is there no page request information on the console network after vue-router jump? When using vue-router for page redirection, you may notice a...
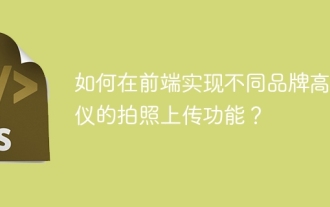
How to implement the photo upload function of different brands of high-photographers on the front end When developing front-end projects, you often encounter the need to integrate hardware equipment. for...
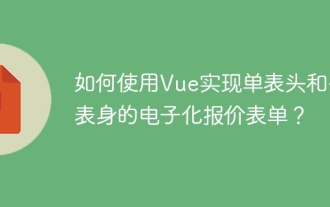
How to implement electronic quotation forms with single header and multi-body in Vue. In modern enterprise management, the electronic processing of quotation forms is to improve efficiency and...
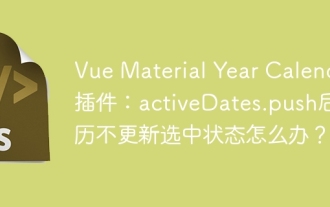
About VueMaterialYear...
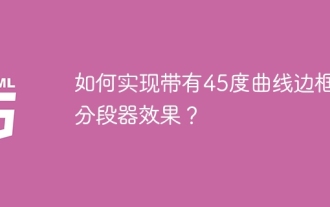
Tips for Implementing Segmenter Effects In user interface design, segmenter is a common navigation element, especially in mobile applications and responsive web pages. ...
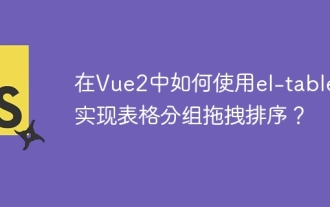
Implementing el-table table group drag and drop sorting in Vue2. Using el-table tables to implement group drag and drop sorting in Vue2 is a common requirement. Suppose we have a...
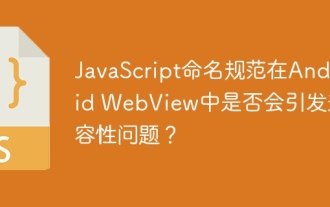
JavaScript Naming Specification and Android...
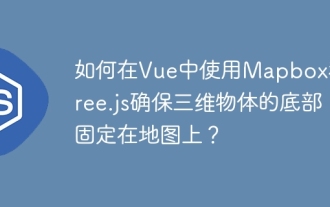
How to use Mapbox and Three.js in Vue to adapt three-dimensional objects to map viewing angles. When using Vue to combine Mapbox and Three.js, the created three-dimensional objects need to...
