


How do PHP and swoole achieve efficient data communication and synchronization?
How do PHP and swoole achieve efficient data communication and synchronization?
In Web development, data communication and synchronization are a very important part. PHP is a widely used scripting language, and swoole is a high-performance PHP extension that can provide asynchronous, multi-threading, multi-process and other advanced features, thus greatly improving the performance and efficiency of PHP. This article will introduce how to use PHP and swoole to achieve efficient data communication and synchronization.
1. Getting started with swoole
Before using swoole, we need to install the swoole extension first. It can be installed through the command line tool. Just execute the following command in the command line:
$ pecl install swoole
After the installation is completed, we can use php --ri swoole
to view swoole related information. Confirm whether swoole is installed successfully.
2. Data Communication
In actual development, we often need to carry out data transmission and communication between different programs. swoole provides rich APIs and functions to achieve efficient data communication. The following is a simple example that demonstrates how to use swoole's Server and Client classes for simple data communication.
- Server code
<?php $server = new SwooleServer('127.0.0.1', 9501); $server->on('connect', function ($server, $fd) { echo "Client: Connect. "; }); $server->on('receive', function ($server, $fd, $fromId, $data) { echo "Received data from client: $data "; $server->send($fd, "Server received: $data"); }); $server->on('close', function ($server, $fd) { echo "Client: Close. "; }); $server->start();
- Client code
<?php $client = new SwooleClient(SWOOLE_SOCK_TCP); $client->connect('127.0.0.1', 9501); $client->send("Hello Server"); echo $client->recv(); $client->close();
In the above code, the server creates a TCP server , and listen to the local port 9501. When the client connects successfully, the server will output the "Client: Connect." message. When the client sends data to the server, the server will receive the data and output the "Received data from client: $data" message. Then the server will return the data to the client as it is, and output "Client: Close." information to indicate that the connection is closed.
After the client connects to the server, it sends a "Hello Server" message, then waits for the message returned by the server through the recv() method, and prints it out. Finally the client closes the connection.
The above is a simple example that demonstrates how to use swoole to implement simple data communication. In actual development, more complex data communication logic can be designed according to specific needs.
3. Data synchronization
Data synchronization means that the data between multiple programs is consistent, and the modification of the data can take effect immediately. In traditional PHP development, because PHP is a single-threaded scripting language, it is less efficient when processing a large number of concurrent requests. However, using swoole can improve the efficiency of data synchronization through asynchronous and multi-process features.
The following is a simple example that demonstrates how to use swoole to implement multi-process concurrent processing of requests:
<?php $server = new SwooleHttpServer('127.0.0.1', 9501, SWOOLE_BASE); $server->set([ 'worker_num' => 4, ]); $server->on('request', function ($request, $response) { $pid = posix_getpid(); echo "Worker $pid handle request. "; sleep(1); // 模拟耗时操作 $response->header('Content-Type', 'text/plain'); $response->end("Hello, Swoole!"); }); $server->start();
In the above code, we created an HTTP server and set up 4 workers The process is used to handle requests. When a request comes in, each worker process will output "Worker $pid handle request." information, and then simulate a time-consuming operation through the sleep() function. Finally, a simple "Hello, Swoole!" response is returned.
This example demonstrates how to use multiple processes to handle requests, and improves concurrent processing capabilities through asynchronous methods. In actual development, we can adjust the number of worker processes according to needs to obtain the best performance.
In summary, through swoole extension, we can achieve efficient data communication by using the Server and Client classes, and improve the efficiency of data synchronization through multi-process and asynchronous methods. Through proper design and use of swoole features, we can optimize the performance and efficiency of web applications. I hope this article will help you understand and use PHP and swoole to achieve efficient data communication and synchronization.
Reference:
- Swoole Documentation: https://www.swoole.co.uk/docs
The above is the detailed content of How do PHP and swoole achieve efficient data communication and synchronization?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


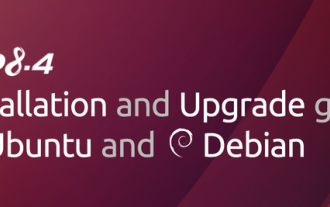
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
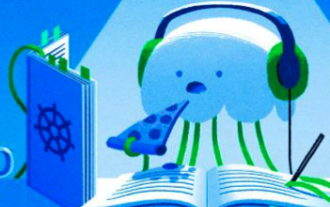
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
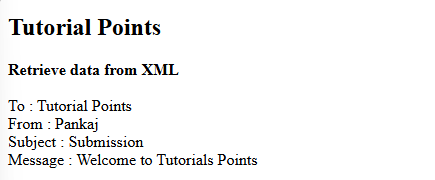
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
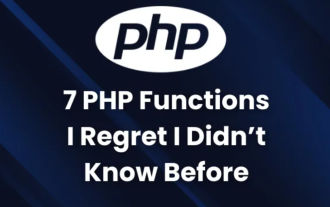
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
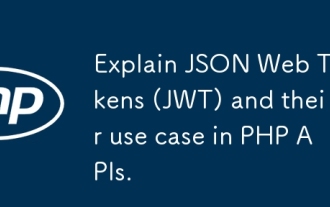
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
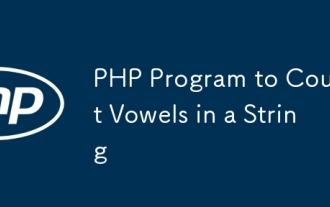
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
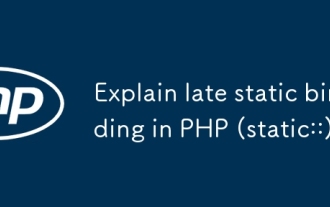
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
