How to use Vue Router to implement dynamic routing tabs?
How to use Vue Router to implement dynamic routing tabs?
Vue Router is the officially recommended routing management plug-in for Vue.js. It provides a simple and flexible way to manage application routing. In our projects, sometimes we need to implement the function of switching multiple pages in the same window, just like tabs in a browser. This article will introduce how to use Vue Router to implement such a dynamic routing tab.
First, we need to install the Vue Router plug-in. You can use the npm or yarn command to install:
npm install vue-router
or
yarn add vue-router
After the installation is complete, create a router folder in the root directory of the project, and create an index under the folder .js files are used to define routing-related configurations. In the index.js file, we need to introduce Vue and Vue Router, and create a new Vue Router instance:
import Vue from 'vue' import Router from 'vue-router' Vue.use(Router) const router = new Router({ routes: [] }) export default router
Next, in our Vue component, we can use < The router-link>
component is used to create navigation links, and the <router-view>
component is used to display the corresponding components. On this basis, we can achieve the switching effect of tabs.
First, we create a <TabBar>
component as the navigation bar to display the tab page:
<template> <div> <router-link v-for="tab in tabs" :key="tab.name" :to="tab.to" active-class="active" class="tab-item" > {{tab.title}} </router-link> </div> </template> <script> export default { data() { return { tabs: [ { title: '首页', to: '/' }, { title: '新闻', to: '/news' }, { title: '关于', to: '/about' } ] } } } </script> <style scoped> .tab-item { padding: 10px; margin-right: 10px; cursor: pointer; } .active { background-color: #eee; } </style>
Then, in our routing configuration file index.js , we can configure the corresponding routes and associate them with components. We can set a unique name for each navigation link and associate its routing path with the corresponding component:
import Vue from 'vue' import Router from 'vue-router' Vue.use(Router) const router = new Router({ routes: [ { path: '/', name: 'Home', component: () => import('@/views/Home.vue') }, { path: '/news', name: 'News', component: () => import('@/views/News.vue') }, { path: '/about', name: 'About', component: () => import('@/views/About.vue') } ] }) export default router
Finally, in our root component App.vue, we can use <router-view>
component to display the corresponding component, and use the <TabBar>
component in the navigation bar to achieve the tab switching effect:
<template> <div id="app"> <tab-bar></tab-bar> <router-view></router-view> </div> </template> <script> import TabBar from '@/components/TabBar.vue' export default { components: { TabBar } } </script>
Through the above Configuration, we can achieve a tab-like effect in the Vue application. When we click on the navigation link, Vue Router will find the corresponding component based on the routing configuration and display it in <router-view>
.
To sum up, with the powerful functions of Vue Router, we can easily implement dynamic routing tabs. By flexibly configuring routing, we can achieve rich and diverse page switching effects in Vue applications, bringing a better interactive experience to users.
The above is the detailed content of How to use Vue Router to implement dynamic routing tabs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
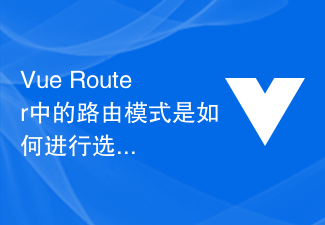
VueRouter is the routing manager officially provided by Vue.js. It can help us implement page navigation and routing functions in Vue applications. When using VueRouter, we can choose different routing modes according to actual needs. VueRouter provides three routing modes, namely hash mode, history mode and abstract mode. The following will introduce in detail the characteristics of these three routing modes and how to choose the appropriate routing mode. Hash mode (default
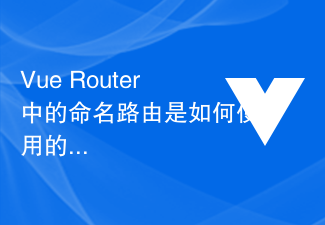
How to use named routes in VueRouter? In Vue.js, VueRouter is an officially provided routing manager that can be used to build single-page applications. VueRouter allows developers to define routes and map them to specific components to control jumps and navigation between pages. Named routing is one of the very useful features. It allows us to specify a name in the routing definition, and then jump to the corresponding route through the name, making the routing jump more convenient.
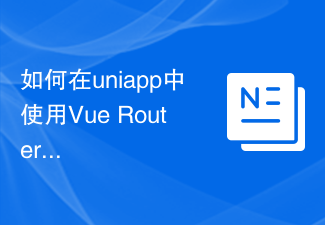
How to use VueRouter for routing jumps in uniapp Using VueRouter for routing jumps in uniapp is a very common operation. This article will introduce in detail how to use VueRouter in the uniapp project and provide specific code examples. 1. Install VueRouter Before using VueRouter, we need to install it first. Open the command line, enter the root directory of the uniapp project, and then execute the following command to install
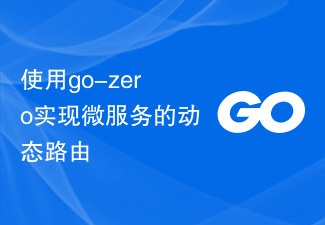
With the popularity of cloud computing and containerization technology, microservice architecture has become a mainstream solution in modern software development. Dynamic routing technology is an essential part of the microservice architecture. This article will introduce how to use the go-zero framework to implement dynamic routing of microservices. 1. What is dynamic routing? In a microservice architecture, the number and types of services may be very large. How to manage and discover these services is a very tricky task. Traditional static routing is not suitable for microservice architecture because the number of services and runtime status change dynamically.
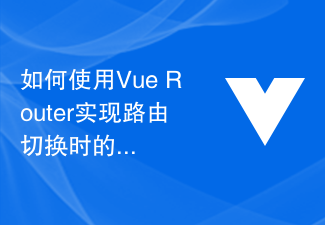
How to use VueRouter to achieve transition effect when routing switching? Introduction: VueRouter is a routing management library officially recommended by Vue.js for building SPA (SinglePageApplication). It can achieve switching between pages by managing the correspondence between URL routing and components. In actual development, in order to improve user experience or meet design needs, we often use transition effects to add dynamics and beauty to page switching. This article will introduce how to use
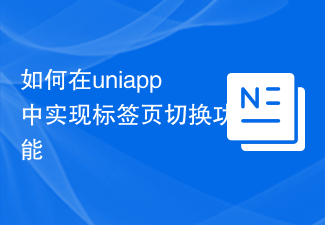
How to implement tab switching function in uniapp 1. Introduction In mobile application development, tab switching is one of the common and important functions. As a cross-platform development framework, Uniapp can develop applications running on multiple platforms at the same time. This article will introduce how to implement the tab switching function in Uniapp and provide some sample code for reference. 2. Use the uni-swiper component Uniapp provides the uni-swiper component, which can easily implement the tab switching function.
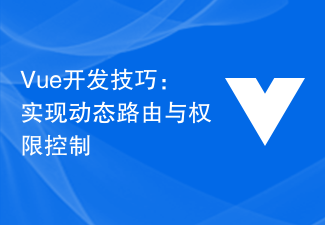
Vue development skills: Implementing dynamic routing and permission control Introduction: In modern web applications, dynamic routing and permission control are essential functions. For large applications, the implementation of these two functions can significantly improve user experience and security. This article will introduce how to use the Vue framework to implement development techniques for dynamic routing and permission control. We will illustrate the specific application of these techniques with examples. 1. Dynamic routing Dynamic routing refers to dynamically creating and parsing routes based on user roles or other conditions when the application is running. pass
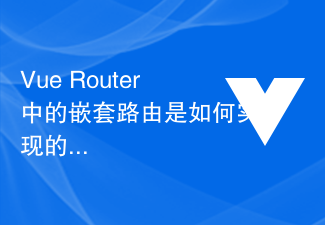
How is nested routing implemented in VueRouter? Vue.js is a popular JavaScript framework for building user interfaces. VueRouter is an official plug-in for Vue.js, used to build a routing system for single-page applications. VueRouter provides a simple and flexible way to manage navigation between different pages and components of your application. Nested routing is a very useful feature in VueRouter, which can easily handle complex page structures.
