


How to use routing to implement international multi-language switching in Vue?
How to use routing to implement international multi-language switching in Vue?
When developing a multi-language website, one of our important needs is to be able to switch website content according to the language selected by the user. Vue.js is a popular JavaScript framework. By using the Vue Router plug-in, we can easily implement routing functions. In this article, I will introduce how to use routing to implement international multi-language switching in Vue.
First, we need to install the Vue Router plug-in. It can be installed through the npm command:
npm install vue-router
After the installation is completed, we can introduce Vue Router into the Vue project and configure routing. In the main.js file, we can introduce and use Vue Router like this:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) const routes = [ { path: '/', name: 'Home', component: () => import('@/views/Home.vue') }, // 其他路由配置... ] const router = new VueRouter({ mode: 'history', base: process.env.BASE_URL, routes }) export default router
Next, we need to prepare the text resource files corresponding to each language. Create a new lang folder in the src directory and create multiple language files in it, such as en.js and zh.js. These language files need to expose an object that contains the corresponding text resource:
// en.js export default { home: 'Home', about: 'About', contact: 'Contact' } // zh.js export default { home: '首页', about: '关于我们', contact: '联系我们' }
Next, use routing and internationalization functions in the Vue component. Where text needs to be displayed, we can obtain the text corresponding to the current language through the $router object:
<template> <div> <nav> <router-link :to="{ name: 'Home' }">{{ $t('home') }}</router-link> <router-link :to="{ name: 'About' }">{{ $t('about') }}</router-link> <router-link :to="{ name: 'Contact' }">{{ $t('contact') }}</router-link> </nav> <router-view></router-view> </div> </template> <script> export default { name: 'App', methods: { $t(key) { const lang = this.$router.currentRoute.meta.lang return this.$options.lang[lang][key] || '' } }, metaInfo() { return { lang: 'en' } } } </script>
In the above code, we obtain the text resource by calling the $t
method . The $t
method first obtains the current language from $router.currentRoute.meta.lang
, and then obtains the corresponding text resource from the $options.lang
object. If the corresponding text is not found, an empty string will be returned.
In order to access the $t
methods and text resource objects in the component, we need to define $t
in the methods
attribute of the Vue instance method, and exposes the text resource object to the component through the lang
property of the $options
object.
Finally, we need to add a beforeEach
hook function in the routing configuration to switch the current language based on the language selected by the user:
// 在router/index.js中的router配置中添加 router.beforeEach((to, from, next) => { const lang = to.query.lang || 'en' // 设置当前路由的meta信息,在组件中可以通过this.$router.currentRoute.meta.lang获取 to.meta.lang = lang next() })
In the above code, beforeEach
The hook function uses to.query.lang
to get the language selected by the user. If no language is selected, English is used by default. The language information is then stored in the meta
attribute of the current route via to.meta.lang
for use in the component.
At this point, we have completed the entire process of using routing to implement international multi-language switching in Vue. By using the Vue Router plug-in and some simple configurations, we can easily implement the multi-language switching function of the website. I hope this article will help you implement multi-language switching in your Vue project.
The above is the detailed content of How to use routing to implement international multi-language switching in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
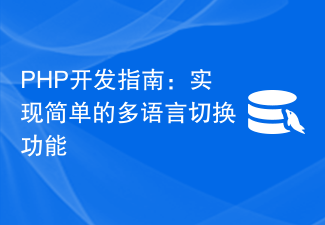
PHP Development Guide: Implementing Simple Multi-Language Switching Function Introduction: With the development of the Internet, more and more websites and applications need to support multi-language functions. In web development, implementing multi-language switching function is a very important task. This article will introduce how to use PHP to implement a simple multi-language switching function, and provide code examples for developers to refer to. 1. Preparation work Before starting to implement the multi-language switching function, we need to do some preparation work. First, we need to determine the supported languages and create the corresponding languages
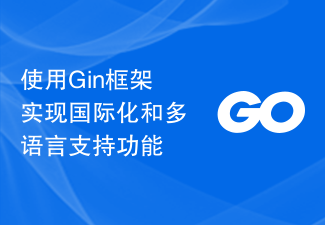
With the development of globalization and the popularity of the Internet, more and more websites and applications have begun to strive to achieve internationalization and multi-language support functions to meet the needs of different groups of people. In order to realize these functions, developers need to use some advanced technologies and frameworks. In this article, we will introduce how to use the Gin framework to implement internationalization and multi-language support capabilities. The Gin framework is a lightweight web framework written in Go language. It is efficient, easy to use and flexible, and has become the preferred framework for many developers. besides,
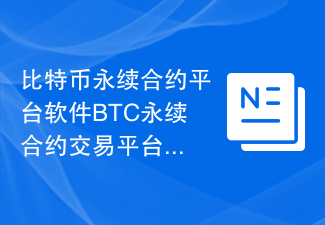
Bitcoin perpetual contract platform software includes binance, CryptoGro, UniswapV3, Bit pro, AMGEX, dYdX, PKEX, currency system, BearBit, and Deepcoin. The relevant introductions of each platform are as follows, interested friends can take a look. Complete list of BTC perpetual contract exchanges 1. Binance: Many investors are familiar with and use the Binance official website exchange. Founded in 2013, Binance’s official website provides services such as fiat currency trading, currency-to-crypto trading, and contract trading. After 8 years of development, Binance’s official website still remains among the top ten virtual currency exchanges, proving its continued strength in the field of blockchain trading. 2. CryptoG
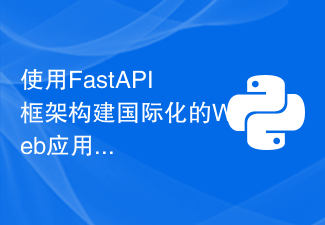
Use the FastAPI framework to build international Web applications. FastAPI is a high-performance Python Web framework that combines Python type annotations and high-performance asynchronous support to make developing Web applications simpler, faster, and more reliable. When building an international Web application, FastAPI provides convenient tools and concepts that can make the application easily support multiple languages. Below I will give a specific code example to introduce how to use the FastAPI framework to build

ThinkPHP6 multi-language switching: realizing international applications With the rapid development of the Internet and the process of globalization, more and more websites and applications need to support multi-language functions to meet the needs of users in different countries and regions. When using ThinkPHP6 to develop web applications, achieving multi-language switching is an important task. This article will introduce how to implement international applications in ThinkPHP6 to provide users with a convenient multi-language experience. Why do you need multi-language switching? In the context of globalization, users use the Internet
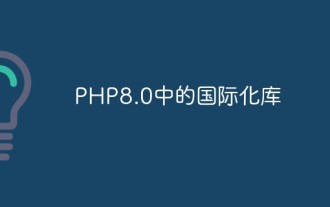
Internationalization library in PHP8.0: UnicodeCLDR and Intl extensions With the process of globalization, the development of cross-language and cross-region applications has become more and more common. Internationalization is an important part of achieving this goal. In PHP8.0, UnicodeCLDR and Intl extensions were introduced, both of which provide developers with better internationalization support. UnicodeCLDRUnicodeCLDR(CommonLocaleDat
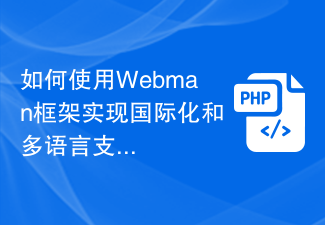
Nowadays, with the continuous development of Internet technology, more and more websites and applications need to support multi-language and internationalization. In web development, using frameworks can greatly simplify the development process. This article will introduce how to use the Webman framework to achieve internationalization and multi-language support, and provide some code examples. 1. What is the Webman framework? Webman is a lightweight PHP-based framework that provides rich functionality and easy-to-use tools for developing web applications. One of them is internationalization and multi-
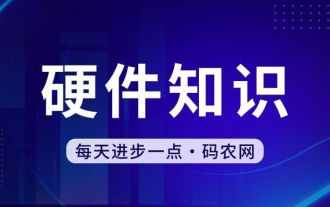
What functions does a laptop keyboard have? 1. F1: If you are in a selected program and need help, press F1. In addition, it can also be adjusted to mute. F2: If a file or folder is selected in the explorer, pressing F2 will rename the selected file or folder. You can also lower the volume. 2. The keyboard is divided into four areas: function key area, main keyboard area, status indication area, and control key area. The keys for individual letters on the keyboard are used for typing. The shift and ctrl keys are the two keys in the red circle that adjust the pinyin key when pressed at the same time. When you press it at the same time it will appear: Pinyin, Alphabet, Five-Character Pen. 3. The most numerous key area in the middle of the keyboard, including numeric keys, letter keys and symbol keys, controls
