


How to achieve efficient concurrent network programming through Goroutines
How to achieve efficient concurrent network programming through Goroutines
With the booming development of the Internet, more and more applications need to handle a large number of network requests. Under the traditional serial programming model, processing a large number of network requests will lead to performance degradation and prolonged response time. By using concurrent programming, tasks can be decomposed into multiple subtasks and multiple threads can be used to process these subtasks simultaneously, thereby significantly improving program performance.
Goroutines are a lightweight coroutine in the Go language, which can achieve efficient concurrent programming. In the Go language, we can use Goroutines to handle multiple network requests at the same time and communicate between them through Channel.
First, we need to import the net
and fmt
packages and create a function that handles client requests.
package main import ( "fmt" "net" ) func handleClient(conn net.Conn) { defer conn.Close() // 处理客户端请求 // ... }
Then, we can create a listener to accept client connections and use the go
keyword to create a new Goroutine to handle each client connection.
func main() { ln, err := net.Listen("tcp", ":8080") if err != nil { fmt.Println("Error listening:", err.Error()) return } defer ln.Close() fmt.Println("Listening on :8080") for { conn, err := ln.Accept() if err != nil { fmt.Println("Error accepting connection:", err.Error()) continue } go handleClient(conn) } }
The above code creates a TCP server listening on the local port 8080. For each client connection, a new Goroutine is created to handle it.
In the handleClient
function, we can handle client requests and communicate over the network connection.
func handleClient(conn net.Conn) { defer conn.Close() // 读取客户端请求 buffer := make([]byte, 1024) _, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } request := string(buffer) fmt.Println("Received request:", request) // 处理客户端请求 response := "Hello, World!" // 发送响应给客户端 _, err = conn.Write([]byte(response)) if err != nil { fmt.Println("Error writing:", err.Error()) return } }
In this example, we simply read the client request and send a "Hello, World!" response.
By using Goroutines and Channels, we can handle multiple client connections at the same time, greatly improving the concurrency and performance of the server. This is a powerful feature of the Go language, which provides a simple and efficient way to implement concurrent programming.
Of course, in actual development, we also need to consider some other factors, such as error handling, connection pool management, etc. But the above examples have shown you the basic principles of efficient concurrent network programming through Goroutines.
In general, by using Goroutines and Channels, we can easily implement efficient concurrent network programming. This allows us to handle large volumes of network requests, providing a better user experience and higher system performance. If you haven't tried concurrent programming in Go yet, I encourage you to start using Goroutines to write efficient concurrent programs.
The above is the detailed content of How to achieve efficient concurrent network programming through Goroutines. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
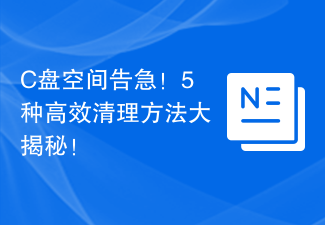
C drive space is running out! 5 efficient cleaning methods revealed! In the process of using computers, many users will encounter a situation where the C drive space is running out. Especially after storing or installing a large number of files, the available space of the C drive will decrease rapidly, which will affect the performance and running speed of the computer. At this time, it is very necessary to clean up the C drive. So, how to clean up C drive efficiently? Next, this article will reveal 5 efficient cleaning methods to help you easily solve the problem of C drive space shortage. 1. Clean up temporary files. Temporary files are temporary files generated when the computer is running.
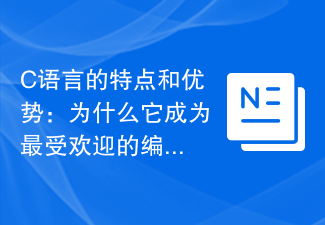
Features and Advantages of C Language: Why is it one of the most popular programming languages? As a general-purpose high-level programming language, C language has many unique features and advantages, which is why it has become one of the most popular programming languages. This article will explore the characteristics and advantages of C language, as well as its wide application in various fields. First of all, C language has concise syntax and clear structure. Compared with other programming languages, the syntax of C language is relatively simple and easy to understand and learn. It uses the characteristics of natural language to enable programmers to

Python and C++ are two popular programming languages, each with its own advantages and disadvantages. For people who want to learn programming, choosing to learn Python or C++ is often an important decision. This article will explore the learning costs of Python and C++ and discuss which language is more worthy of the time and effort. First, let's start with Python. Python is a high-level, interpreted programming language known for its ease of learning, clear code, and concise syntax. Compared to C++, Python
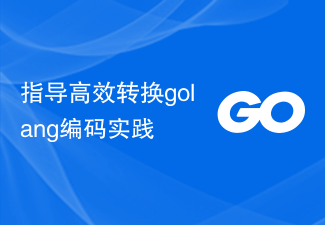
Title: Efficient Practice Guide for Go Language Encoding Conversion In daily software development, we often encounter the need to convert text in different encodings. As an efficient and modern programming language, Go language provides a rich standard library and built-in functions, making it very simple and efficient to implement text encoding conversion. This article will introduce practical guidelines on how to perform encoding conversion in the Go language and provide specific code examples. 1.UTF-8 encoding and string conversion In Go language, strings use UTF-8 encoding by default
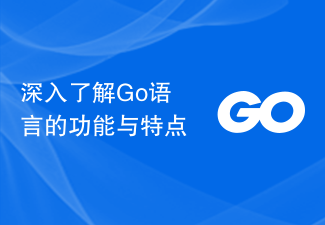
Functions and features of Go language Go language, also known as Golang, is an open source programming language developed by Google. It was originally designed to improve programming efficiency and maintainability. Since its birth, Go language has shown its unique charm in the field of programming and has received widespread attention and recognition. This article will delve into the functions and features of the Go language and demonstrate its power through specific code examples. Native concurrency support The Go language inherently supports concurrent programming, which is implemented through the goroutine and channel mechanisms.
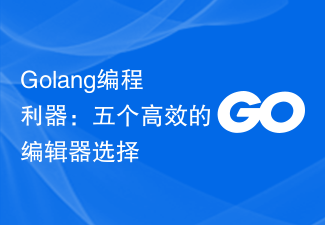
In today's era of rapid development of the Internet, programming has become more and more important. As a new programming language, Golang is particularly important in this era. Likewise, choosing an efficient editor is also very important to improve programming efficiency. In this article, we will introduce five efficient editors to provide better support for Golang programming. VisualStudioCode is an open source project produced by Microsoft. VisualStudioCode has become the editor of choice for many developers.
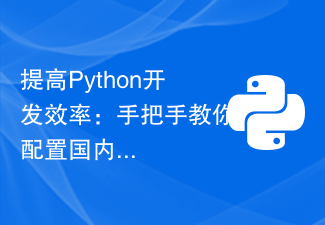
Starting from scratch, we will teach you step by step how to configure the domestic source of pip to make your Python development more efficient. During the development process of Python, we often use pip to manage third-party libraries. However, due to domestic network environment problems, using the default pip source often results in slow download speeds or even inability to connect. In order to make our Python development more efficient, it is necessary to configure a domestic source. So, let us now configure the pip domestic source step by step! First, we need to find pip
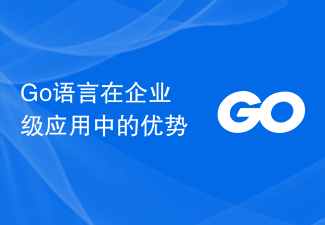
One of the reasons why thinking about using Go language for enterprise-level application development is gaining more and more attention is its excellent performance and concurrency features. This article will explore the advantages of Go language in enterprise-level application development and give specific code examples to illustrate these advantages. 1. Concurrent programming capabilities Go language achieves efficient concurrent programming through the goroutine mechanism, which gives it obvious advantages in handling concurrent tasks. Using goroutine, we can easily create concurrent tasks and execute them through channels
