How to use Vue and Element-UI to implement drag-and-drop sorting function
How to use Vue and Element-UI to implement drag-and-drop sorting function
Foreword:
In web development, drag-and-drop sorting function is a common and practical function. This article will introduce how to use Vue and Element-UI to implement the drag-and-drop sorting function, and demonstrate the implementation process through code examples.
1. Environment setup
- Installing Node.js
Before starting, you need to install Node.js. You can visit https://nodejs.org/ to download and install the version corresponding to the operating system. - Install Vue CLI
Vue CLI is a tool used to quickly build Vue projects. Open the command line tool and execute the following command to install Vue CLI:
npm install -g @vue/cli
- Create a Vue project
Execute the following in the command line Command to create a new Vue project:
vue create drag-sort-demo
Follow the prompts to select configuration options and wait for the project to be created.
- Install Element-UI
Enter the Vue project directory you just created, and execute the following command on the command line to install Element-UI:
npm install element-ui
2. Implement drag-and-drop sorting function
- Import Element-UI
Import the styles and components of Element-UI in the entry file src/main.js of the Vue project:
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
- Create demo component
Create a new component DragSortDemo.vue in the src/components directory to demonstrate the drag-and-drop sorting function. Edit the DragSortDemo.vue file and add the following code:
<div class="drag-sort-demo">
<el-collapse-transition>
<div v-for="item in list" :key="item.id" class="drag-item" :class="{ 'dragging': draggingId === item.id }" draggable="true" @dragstart="handleDragStart(item)" @dragend="handleDragEnd(item)">
{{ item.name }}
</div>
</el-collapse-transition>
</div>
<el-button type="primary" @click="handleSort">保存排序</el-button>
<script><br>export default {<br> data() {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>return { list: [ { id: 1, name: 'A' }, { id: 2, name: 'B' }, { id: 3, name: 'C' }, { id: 4, name: 'D' }, { id: 5, name: 'E' }, ], draggingId: null, };</pre><div class="contentsignin">Copy after login</div></div><p>},<br> methods: {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>handleDragStart(item) { this.draggingId = item.id; }, handleDragEnd() { this.draggingId = null; }, handleSort() { const sortedList = Array.from(this.$el.querySelectorAll('.drag-item')).map((el, index) => { const id = el.getAttribute('data-id'); const name = el.textContent; return { id, name, sort: index + 1 }; }); // 将排序后的列表保存到数据库或发送给后端 },</pre><div class="contentsignin">Copy after login</div></div><p>},<br> };<br></script>
Explanation:
- In the template, use the v-for directive to dynamically render list items. The class of the list item is bound to a calculated property, which is used to determine whether the currently dragged item is the list item.
- Add the draggable attribute to each list item, and register the dragstart and dragend event handlers to trigger the drag start and end events respectively.
- The handleSort method saves or sends the drag-and-drop sorted list data to the backend for processing.
- Using components
Use the DragSortDemo component in the src/App.vue file:
<drag-sort-demo></drag-sort-demo>
<script><br>import DragSortDemo from './components/DragSortDemo.vue' ;</p><p>export default {<br> components: {</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>DragSortDemo,</pre><div class="contentsignin">Copy after login</div></div><p>},<br>};<br></script>
3. Run the project
Execute the following command in the command line to start the project:
npm run serve
Visit http://localhost:8080 to see the demonstration page of the drag and drop sorting function. Drag the list items to change the sorting, and click the Save Sorting button to save the sorting results.
Conclusion:
Through the above steps, we successfully implemented the drag-and-drop sorting function using Vue and Element-UI. This provides a simple and practical solution for our web development, which can improve user experience and optimize interface interaction. I hope this article can be helpful to everyone's front-end development work.
The above is the detailed content of How to use Vue and Element-UI to implement drag-and-drop sorting function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


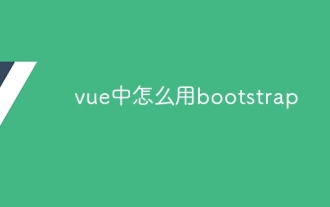
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
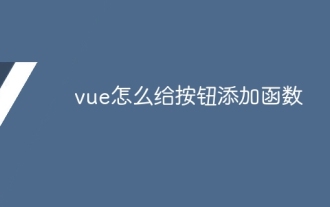
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
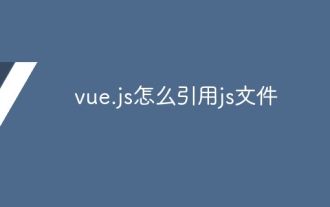
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
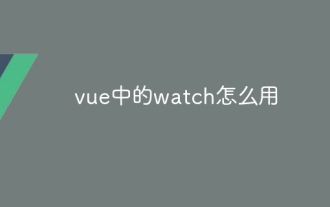
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
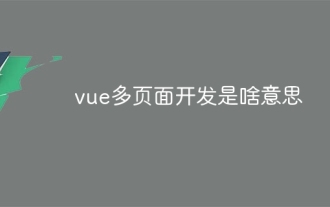
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
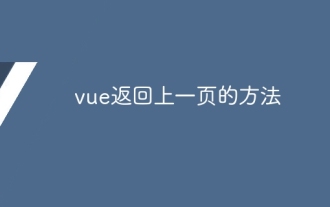
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
