How to use context to implement request caching in Go
How to use context to implement request caching in Go
Introduction:
When building web applications, we often need to cache requests to improve performance. In the Go language, we can use the context package to implement the request caching function. This article will introduce how to use the context package to implement request caching, and provide code examples to help readers better understand.
What is context? :
In the Go language, the context package provides a way to pass request-related data, cancellation signals, timeouts, etc. between multiple goroutines. The context package is Go's officially recommended way of handling the content required for requests.
Implementing request caching:
Before using context to implement request caching, we first need to understand what request caching is. Request caching means that when the same request is received, the response is returned directly from the cache instead of executing the request processing logic again.
The following is a simple example that demonstrates how to use context to implement a basic request caching function.
package main import ( "context" "fmt" "sync" "time" ) type Cache struct { data map[string]string mu sync.RWMutex } func NewCache() *Cache { return &Cache{ data: make(map[string]string), } } func (c *Cache) Get(key string) (string, bool) { c.mu.RLock() defer c.mu.RUnlock() value, ok := c.data[key] return value, ok } func (c *Cache) Set(key string, value string) { c.mu.Lock() defer c.mu.Unlock() c.data[key] = value } func requestHandler(ctx context.Context, cache *Cache, key string) { // 从缓存中获取数据 if value, ok := cache.Get(key); ok { fmt.Println("From cache:", value) return } // 模拟数据处理过程 time.Sleep(2 * time.Second) value := "Data from server" // 存储数据到缓存 cache.Set(key, value) fmt.Println("From server:", value) } func main() { cache := NewCache() // 为了演示效果,我们模拟同时进行多个请求 for i := 0; i < 5; i++ { go requestHandler(context.Background(), cache, "key") } // 等待所有请求处理完成 time.Sleep(5 * time.Second) }
In the above sample code, we created a Cache structure to simulate cache storage. The Get method is used to obtain the cached value, and the Set method is used to set the cached value. In the requestHandler function, we first try to get the data from the cache and return it directly if it exists. Otherwise, we simulate the time-consuming data processing process and store the data in the cache.
In the main function, we create a Cache instance and use context.Background() as the context of the request. In order to demonstrate the effect, we processed 5 requests at the same time and waited for all request processing to be completed at the end.
Run the above code, you will see an output similar to the following:
From server: Data from server From server: Data from server From server: Data from server From server: Data from server From server: Data from server
As can be seen from the output, the first request needs to wait 2 seconds for data processing and Data is stored in cache. In subsequent requests, the previously stored data is obtained directly from the cache, avoiding time-consuming data processing.
Conclusion:
By using the context package, we can easily implement the request caching function. In a real web application, we can use the request caching feature in conjunction with other features to improve performance and response speed.
Reference materials:
- [Go language official document - context](https://golang.org/pkg/context/)
- [Using Context Package in Go](https://blog.golang.org/context)
The above is the detailed content of How to use context to implement request caching in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


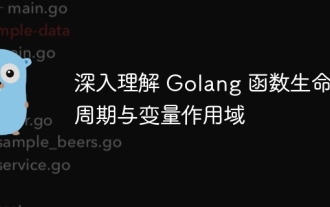
In Go, the function life cycle includes definition, loading, linking, initialization, calling and returning; variable scope is divided into function level and block level. Variables within a function are visible internally, while variables within a block are only visible within the block.
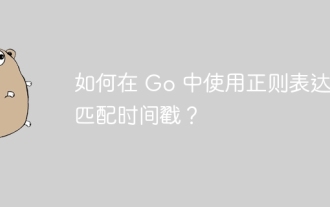
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
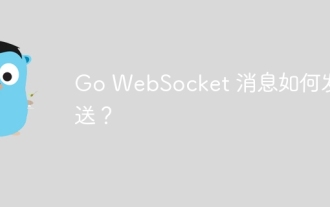
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
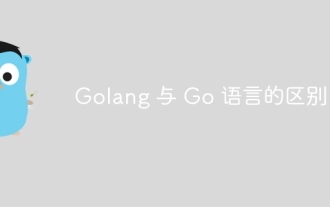
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
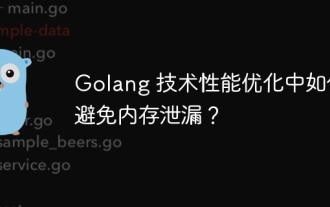
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
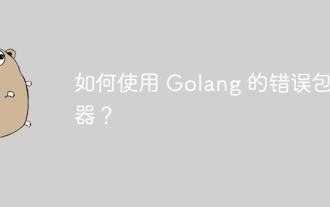
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
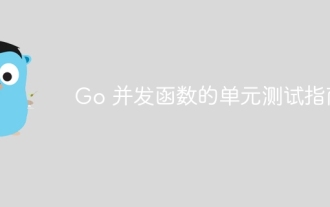
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
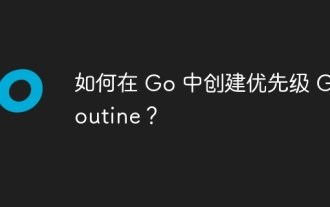
There are two steps to creating a priority Goroutine in the Go language: registering a custom Goroutine creation function (step 1) and specifying a priority value (step 2). In this way, you can create Goroutines with different priorities, optimize resource allocation and improve execution efficiency.
