


Use the containsAll() method of the HashSet class to determine whether one set contains all elements in another set
Use the containsAll() method of the HashSet class to determine whether a collection contains all elements in another collection
HashSet is an unordered, non-duplicate collection class provided by the Java collection framework. It is implemented based on a hash table and can quickly insert, delete, and search elements. In many scenarios, we need to determine whether a set contains all elements in another set. Java provides the containsAll() method to meet this requirement.
The code example is as follows:
import java.util.HashSet; public class HashSetContainsAllExample { public static void main(String[] args) { // 创建两个HashSet集合 HashSet<Integer> set1 = new HashSet<Integer>(); HashSet<Integer> set2 = new HashSet<Integer>(); // 向set1中添加元素 set1.add(1); set1.add(2); set1.add(3); set1.add(4); // 向set2中添加元素 set2.add(2); set2.add(4); // 使用containsAll()方法判断set1是否包含set2中的所有元素 boolean result = set1.containsAll(set2); if (result) { System.out.println("set1包含set2中的所有元素"); } else { System.out.println("set1不包含set2中的所有元素"); } } }
In the above code, we created two HashSet sets (set1 and set2) and added elements 1, 2, 3 and 4 to set1 , elements 2 and 4 are added to set2. Then, we use the containsAll() method to determine whether set1 contains all elements in set2. Finally, the corresponding information is printed based on the returned results.
Run the above code, you will get the following output:
set1不包含set2中的所有元素
Explanation that set1 does not contain all elements in set2. This is because element 2 is missing from set1, so the containsAll() method returns false.
Using the containsAll() method can very conveniently determine whether a collection contains all elements in another collection. This method is not only applicable to HashSet, but other collection classes that implement the Set interface (such as TreeSet).
It should be noted that the judgment of the containsAll() method is based on the equals() method of the element, not based on the memory address. This means that when we determine whether two sets are equal, we only need to compare whether the elements in them are equal, and do not need to compare whether their reference addresses are equal.
To summarize, using the containsAll() method of the HashSet class can easily and efficiently determine whether a set contains all elements in another set. This method is very useful in actual development and can help us quickly solve related problems.
The above is the detailed content of Use the containsAll() method of the HashSet class to determine whether one set contains all elements in another set. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


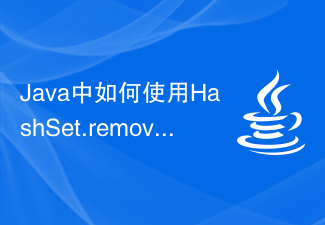
Use the HashSet.remove() method in Java to remove specified elements from a collection. HashSet is a collection class that implements the Set interface. It does not allow the storage of duplicate elements and does not guarantee the order of elements. When operating a HashSet, you can use the remove() method to delete elements in the set. The remove() method of HashSet has two overloaded forms: booleanremove(Objectobj): removes the specified object from the collection

The HashSet function in Java is a collection class implemented based on a hash table. Since it is a collection class, it naturally has the function of collection operations. This article will introduce how to use the HashSet function to perform collection operations. 1. Definition and declaration of HashSet HashSet is a collection class, so you need to import the Java.util package first. importjava.util.HashSet; Then you can create a HashSet instance: HashSet<
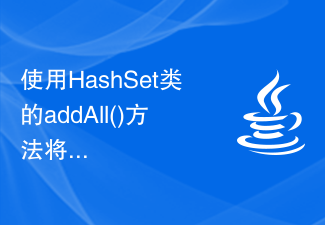
Use the addAll() method of the HashSet class to add all elements in a collection to another collection. HashSet is an implementation class in the Java collection framework. It inherits from AbstractSet and implements the Set interface. HashSet is an unordered set based on a hash table, which does not allow duplicate elements. It provides many commonly used methods to operate elements in the collection, one of which is the addAll() method. The function of the addAll() method is to add the specified
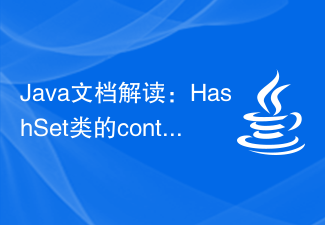
Interpretation of Java documentation: Detailed explanation of the usage of the contains() method of the HashSet class. The HashSet class is one of the commonly used collection classes in Java. It implements the Set interface and is based on the hash table data structure, with efficient insertion, deletion and search operations. Among them, the contains() method is an important method provided by the HashSet class, which is used to determine whether the set contains the specified element. This article will analyze in detail the usage of the contains() method of the HashSet class, and
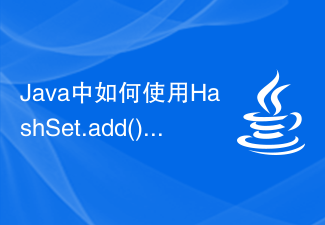
It is very simple to add elements to a collection using the HashSet.add() method in Java. Let’s introduce it in detail below. HashSet is a collection class in Java. It inherits from the AbstractSet class and implements the Set interface. The characteristics of HashSet are unordered and non-repeating, and the underlying implementation is based on a hash table. When using the HashSet.add() method to add elements, you need to pay attention to the following points: HashSet can only store elements of object type, not
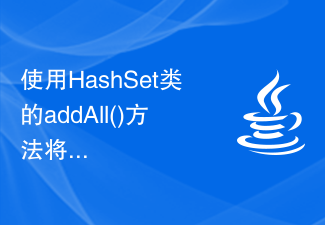
Use the addAll() method of the HashSet class to add a collection to another collection. HashSet is a collection class in Java. It implements the Set interface and is implemented based on a hash table. Duplicate elements are not allowed in the HashSet collection, and the elements in the collection are unordered. In development, we often need to add elements from one collection to another collection. The HashSet class provides the addAll() method to easily implement this function. Below we will go through a
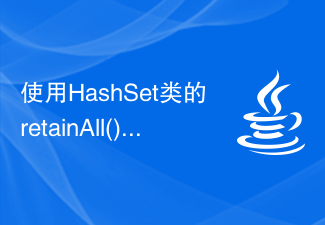
Use the retainAll() method of the HashSet class to obtain the intersection of two collections. HashSet is a collection class in Java that is used to store a set of unique objects. The retainAll() method is a method provided by the HashSet class and is used to obtain the intersection of two HashSets. In Java, a collection is a commonly used data structure that can be used to store multiple objects. HashSet is a commonly used concrete implementation in collection classes. It stores and retrieves objects through hash tables.
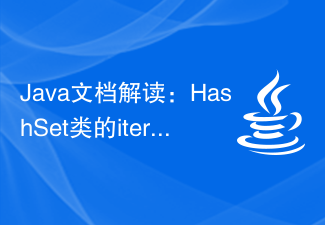
Interpretation of Java documentation: Detailed explanation of the usage of the iterator() method of the HashSet class. Specific code examples are required. In Java programming, HashSet is one of the commonly used collection classes. It implements the Set interface and inherits from the AbstractSet class. The iterator() method of the HashSet class is used to return an iterator object for traversing the elements in the HashSet. This article will explain in detail the usage of iterator() method of HashSet class, and
