


Java uses the delete() function of the File class to delete files or directories
Java uses the delete() function of the File class to delete files or directories
In Java programming, processing files and directories is a common task. Sometimes, we need to delete a file or a directory. In this case, we can use the delete() function of the File class to complete it.
The File class is a class used to operate files and directories in Java. It contains many useful methods, among which the delete() function is used to delete files or directories. Below is a sample code on how to delete a file or directory using the delete() function.
Example 1: Delete a single file
import java.io.File; public class DeleteFileExample { public static void main(String[] args) { // 创建一个File对象,表示要删除的文件 File file = new File("C:/example.txt"); // 判断文件是否存在 if (file.exists()) { // 调用delete()函数删除文件 if (file.delete()) { System.out.println("文件删除成功!"); } else { System.out.println("文件删除失败!"); } } else { System.out.println("文件不存在!"); } } }
Example 2: Delete the directory and all files under it
import java.io.File; public class DeleteDirectoryExample { public static void main(String[] args) { // 创建一个File对象,表示要删除的目录 File directory = new File("C:/example"); // 调用deleteDirectory()函数删除目录 if (deleteDirectory(directory)) { System.out.println("目录删除成功!"); } else { System.out.println("目录删除失败!"); } } private static boolean deleteDirectory(File directory) { // 判断目录是否存在 if (!directory.exists()) { return true; } // 判断是否为目录 if (!directory.isDirectory()) { return false; } // 获取目录下的所有文件和子目录 File[] files = directory.listFiles(); // 递归删除目录中的所有文件和子目录 for (File file : files) { if (file.isDirectory()) { deleteDirectory(file); } else { file.delete(); } } // 删除空目录 return directory.delete(); } }
In the above code, we first create a File class to express the deletion A file or directory object. For example 1, we first determine whether the file exists, and then call the delete() function to delete it. For example 2, we first determine whether the directory exists, then use recursion to delete all files and subdirectories in the directory, and finally delete the empty directory.
It should be noted that in actual applications, deleting files or directories is a sensitive operation and needs to be handled with caution. The deletion operation is irreversible. Once deleted, it cannot be recovered. Therefore, before deleting, we need to confirm whether we want to delete it to avoid unnecessary losses.
In summary, using the delete() function of the File class can conveniently delete files or directories. With this function, we can easily delete files or directories that are no longer needed, keeping the file system clean and organized. In actual development, we should use the delete() function reasonably and do a good job of data backup and confirmation before deletion.
The above is the detailed content of Java uses the delete() function of the File class to delete files or directories. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
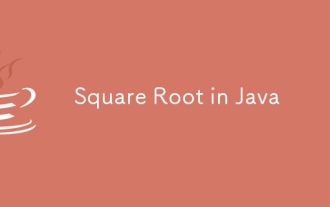
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
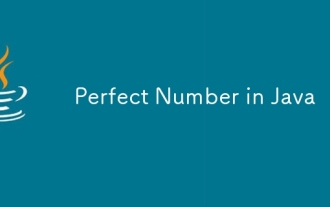
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
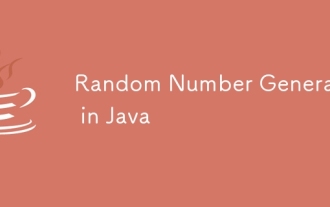
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
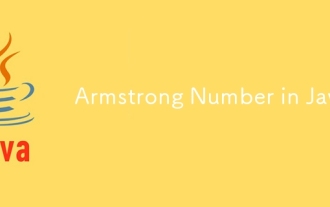
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
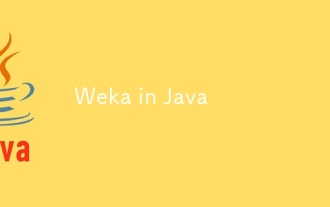
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
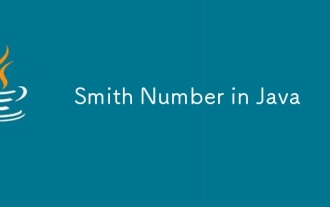
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
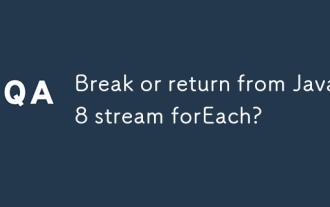
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
