


Use the strings.Split function to split a string into multiple substrings according to the specified delimiter.
Use the strings.Split function to split the string into multiple substrings according to the specified delimiter
In Go language, we can use the Split function in the strings package to split the string according to the specified delimiter. Split into multiple substrings. This is very useful when working with strings, especially when we need to split, parse, or extract specific content from the string.
The prototype of the Split function is as follows:
func Split(s, sep string) []string
Among them, s represents the string that needs to be split, and sep is used Delimiter to separate strings. This function returns a slice consisting of split substrings.
Let's look at a specific example demonstrating how to use the Split function for string splitting.
package main import ( "fmt" "strings" ) func main() { str := "apple,banana,orange" separator := "," // 使用Split函数将字符串拆分成多个子串 result := strings.Split(str, separator) // 遍历切片并输出结果 for _, s := range result { fmt.Println(s) } }
In the above example code, we defined a string str as "apple, banana, orange" and a separator separator as ",". Then, we called the Split function to split the string str according to the separator separator.
Finally, we use a for loop to traverse the split substring slice result and output each substring through the fmt.Println function.
When we run the above code, we will get the following output:
apple banana orange
As we can see, the Split function is used to split the string into three sub-strings according to the specified delimiter. String "apple", "banana" and "orange".
It should be noted that when the Split function splits a string, the delimiter itself will be used as the mark of splitting, but it will not be included in the split substring. In the above example, the separator "," does not appear in the output.
In addition, if no delimiter appears in the string, the Split function will return a slice containing only one element, which is the original string itself.
To summarize, using the strings.Split function can easily split a string into multiple substrings according to the specified delimiter. This is very useful when working with strings, especially if you need to split, parse, or extract specific content. Whether it is a comma, space, semicolon, or other specific custom delimiter, you can use the Split function to split the string.
The above is the detailed content of Use the strings.Split function to split a string into multiple substrings according to the specified delimiter.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


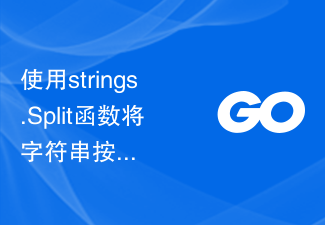
Use the strings.Split function to split a string into multiple substrings according to the specified delimiter. In the Go language, we can use the Split function in the strings package to split a string into multiple substrings according to the specified delimiter. This is very useful when working with strings, especially when we need to split, parse, or extract specific content from the string. The prototype of the Split function is as follows: funcSplit(s,sepstring)[]string where, s
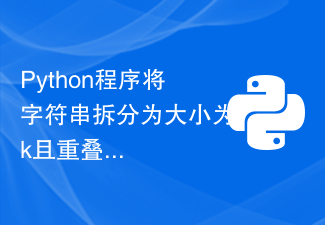
Splitting a string into smaller parts is a common task in many text processing and data analysis scenarios. In this blog post, we will explore how to write a Python program that splits a given string into overlapping strings of size k. This program can be very useful when working with data sequences that require analysis, feature extraction, or pattern recognition. Understanding the Problem Before we dive into implementation details, let's define the requirements of our program. We need to develop a Python solution that takes a string as input and splits it into overlapping strings of size k. For example, if the given string is "Hello, world!" and k is 3, then the program should generate the following repetition
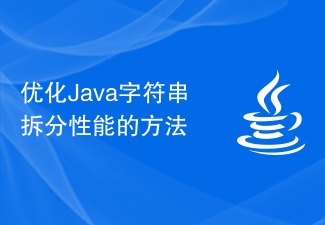
How to solve the string splitting performance problem in Java development. In Java development, string processing is a very common operation. Among them, the string split operation is indispensable in many scenarios. However, as the size of data increases, the performance problem of string splitting gradually becomes prominent, which has a negative impact on the execution efficiency of the program. This article will explore how to solve string splitting performance problems in Java development and provide some practical optimization suggestions. 1. Avoid using regular expressions in Java
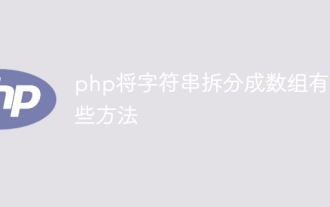
The methods are: 1. The explode() function can split the string into an array according to the specified delimiter; 2. The str_split() function can split the string into an array of single characters; 3. The preg_split() function, Strings can be split into arrays based on regular expressions; 4. The sscanf() function can parse strings according to the specified format and store the parsing results in arrays; 5. String interception method, by using string interception Function, you can split a string into an array according to the specified length, etc.
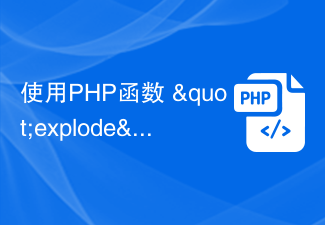
Use the PHP function "explode" to split a string into an array. In PHP development, you often encounter situations where you need to split a string according to the specified delimiter. At this time, we can use PHP's built-in function "explode" to convert string to array. This article will introduce how to use the "explode" function to split a string and give relevant code examples. The basic syntax of the "explode" function is as follows: arrayexplode(
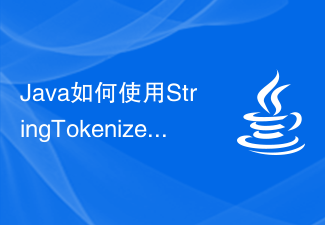
How to use the StringTokenizer class in Java to split a string into multiple substrings Introduction: In Java development, it is often necessary to split a string into multiple substrings for further processing. Java provides many methods to split strings, one of the commonly used tools is the StringTokenizer class. This article will introduce the basic usage of the StringTokenizer class and provide code examples to help readers better understand. StringToknizer
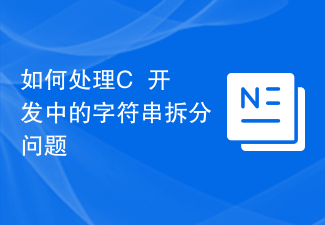
How to deal with string splitting in C++ development In C++ development, string splitting is a common problem. When we need to split a string according to a specific delimiter, such as splitting a sentence into words, or splitting each row of a CSV file into different fields, we need to use an efficient and reliable Method to handle string splitting problem. The following will introduce several commonly used methods to deal with string splitting problems in C++ development. use stringstreamstringst
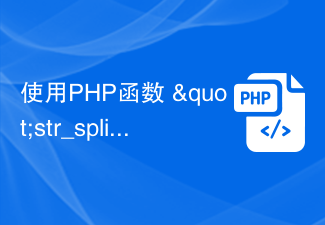
Use the PHP function "str_split" to split a string into a character array. In PHP, sometimes we need to split a string into a character array. In this case, we can use the PHP function "str_split" to easily achieve this. This article will introduce how to use the "str_split" function and some examples of its usage. The basic syntax of the "str_split" function is as follows: arraystr_split(string$string[
