Home
Web Front-end
H5 Tutorial
HTML5 audio tag uses js for playback control example_html5 tutorial skills



HTML5 audio tag uses js for playback control example_html5 tutorial skills
May 16, 2016 pm 03:46 PM
audio
html5
js
Label
The
<audio> tag can play audio files in HTML5 browsers.
<audio>A control panel is provided by default, but sometimes we only need to play sounds, and the control panel is up to us to define its displayed status.
Here we can use JS to control, the code is as follows:
Copy the code
The code is as follows:var audio ;
window.onload = function(){
initAudio();
}
var initAudio = function(){
//audio = document. createElement("audio")
//audio.src='Never Say Good Bye.ogg'
audio = document.getElementById('audio');
}
function getCurrentTime(id){
alert(parseInt(audio.currentTime) ': seconds');
}
function playOrPaused(id,obj){
if(audio.paused){
audio. play();
obj.innerHTML='pause';
return;
}
audio.pause();
obj.innerHTML='play';
}
function hideOrShowControls(id,obj){
if(audio.controls){
audio.removeAttribute('controls');
obj.innerHTML = 'Show control box'
return ;
}
audio.controls = 'controls';
obj.innerHTML = 'Hide control box'
return;
}
function vol(id,type, obj){
if(type == 'up'){
var volume = audio.volume 0.1;
if(volume >=1 ){
volume = 1 ;
}
audio.volume = volume;
}else if(type == 'down'){
var volume = audio.volume - 0.1;
if(volume <=0 ){
volume = 0 ;
}
audio.volume = volume;
}
document.getElementById('nowVol').innerHTML = returnFloat1(audio.volume);
}
function muted(id,obj){
if(audio.muted){
audio.muted = false;
obj.innerHTML = 'Turn on mute';
}else{
audio. muted = true;
obj.innerHTML = 'Turn off mute';
}
}
//Retain one decimal point
function returnFloat1(value) {
value = Math.round(parseFloat(value) * 10) / 10;
if (value.toString().indexOf(".") < 0){
value = value.toString() ".0" ;
}
return value;
}
The calling method is as follows:
Copy code
The code is as follows:<a href="javascript:void (0);" onclick="getCurrentTime('firefox');">Get play time</a>
<a href="javascript:void(0);" onclick="playOrPaused('firefox ',this);">Play</a>
<a href="javascript:void(0);" onclick="hideOrShowControls('firefox',this);">Hide control box< ;/a>
<a href="javascript:void(0);" onclick="muted('firefox',this);">Turn on mute</a>
<input type ="button" value=" " id="upVol" onclick="vol('firefox' , 'up' , this )"/>Volume<input type="button" value="-" onclick="vol ('firefox' , 'down' ,this )"/>
<audio src="/images/audio/Never Say Good Bye.ogg" id="audio" controls="controls" >< /audio>
Current volume: <span id = "nowVol"> - </span>
Statement of this Website
The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn

Hot Article
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Difficulty in updating caching of official account web pages: How to avoid the old cache affecting the user experience after version update?
3 weeks ago
By 王林
Two Point Museum: All Exhibits And Where To Find Them
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌

Hot tools Tags

Hot Article
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Difficulty in updating caching of official account web pages: How to avoid the old cache affecting the user experience after version update?
3 weeks ago
By 王林
Two Point Museum: All Exhibits And Where To Find Them
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
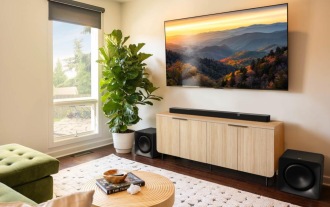
Klipsch unveils Flexus Core 300 flagship soundbar with 8K support, 12 speakers and room correction
