What are the data structures of PHP?
Common PHP data structures include: 1. Array, which can store multiple values and is widely used to store and operate a group of related data; 2. Stack, you can use arrays to simulate the behavior of a stack; 3. Queue , use the push() and shift() functions of the array to add elements to the end of the queue, and remove elements from the beginning of the queue; 4. Linked lists, nodes can be added and deleted dynamically, but accessing nodes requires traversing the entire linked list; 5. Heap, used to implement algorithms such as priority queue and heap sorting; 6. Hash table; 7. Tree; 8. Graph and other data structures.
The operating environment of this tutorial: Windows 10 system, PHP8.1.3 version, Dell G3 computer.
Data structures play a vital role in computer science. It is a way of processing and organizing data that provides efficient data manipulation and storage. In the PHP programming language, there are many commonly used data structures that help developers solve problems and optimize algorithms. This article will introduce some commonly used PHP data structures and their usage.
1. Array: Array is one of the most commonly used data structures in PHP. It can store multiple values and index them using key-value pairs. PHP's arrays have dynamic sizes, and elements can be added, removed, and modified at runtime. Arrays are widely used in PHP to store and manipulate a group of related data.
2. Stack: The stack is a last-in-first-out (LIFO) data structure. In PHP, you can use arrays to simulate the behavior of a stack. Elements can be added to and removed from the top of the stack by using the array's push() and pop() functions.
3. Queue: Queue is a first-in-first-out (FIFO) data structure. In PHP, arrays can also be used to simulate queues. You can use the push() and shift() functions of arrays to add elements to the end of the queue and to remove elements from the beginning of the queue.
4. Linked List: A linked list is a data structure composed of nodes. Each node contains data and a reference to the next node. Linked lists can be implemented in PHP through custom classes. The characteristic of a linked list is that nodes can be added and deleted dynamically, but accessing a node requires traversing the entire linked list.
5. Heap: Heap is a special tree structure with the nature of an ordered relationship between parent nodes and child nodes. In PHP, you can use arrays to represent heaps. The heap is mainly used to implement algorithms such as priority queue and heap sort.
6. Hash Table: A hash table is a data structure that is directly accessed based on keywords. PHP has a built-in implementation of hash tables, which can be created in the form of associative arrays. Hash tables have constant time complexity in search and insertion operations and are very efficient.
7. Tree: Tree is a hierarchical data structure consisting of nodes and edges. Trees can be implemented using classes in PHP. Trees are widely used, for example, binary search trees are used for fast search operations, Huffman trees are used for data compression, etc.
8. Graph: A graph is a nonlinear data structure composed of nodes and edges. In PHP you can use classes to implement graphs. Common applications of graphs include routing algorithms, social network analysis, and image processing.
These are some commonly used data structures in PHP. Each data structure has its own characteristics and applicable scenarios. Understanding and mastering these data structures is crucial to developing efficient PHP applications. Whether it is processing large-scale data, optimizing algorithms or building complex data structures, data structures are one of the essential knowledge for programmers. By using appropriate data structures, you can improve program performance and readability.
The above is the detailed content of What are the data structures of PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


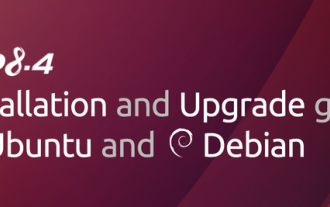
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
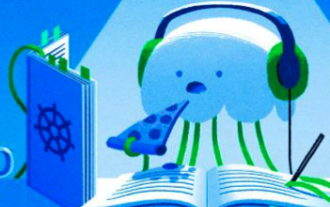
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
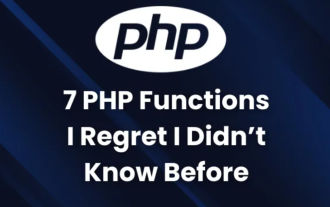
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
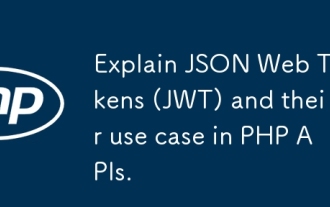
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
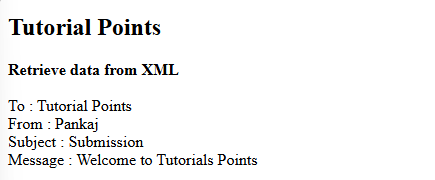
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
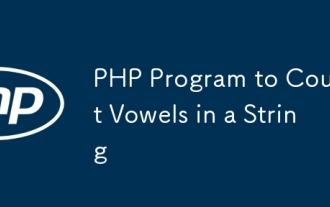
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
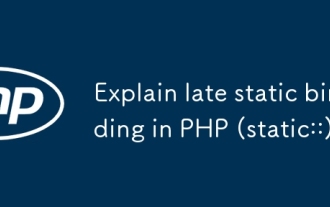
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
