Reverse sort slices using sort.Reverse function
Use the sort.Reverse function to reverse sort the slice
In the Go language, the slice is an important data structure that can dynamically increase or decrease the number of elements. When we need to sort slices, we can use the functions provided by the sort package to perform sorting operations. Among them, the sort.Reverse function can help us reverse sort the slices.
The sort.Reverse function is a function in the sort package. It accepts a parameter of sort.Interface interface type and returns a new sort.Interface type object, which will be sorted in descending order. way to sort.
The following is a simple example that shows how to use the sort.Reverse function to reverse sort a slice:
package main import ( "fmt" "sort" ) type Person struct { Name string Age int } type ByAge []Person func (a ByAge) Len() int { return len(a) } func (a ByAge) Swap(i, j int) { a[i], a[j] = a[j], a[i] } func (a ByAge) Less(i, j int) bool { return a[i].Age < a[j].Age } func main() { people := []Person{ {"Alice", 25}, {"Bob", 30}, {"Charlie", 20}, {"David", 35}, } fmt.Println("Before reverse sorting:") for _, person := range people { fmt.Println(person) } sort.Sort(sort.Reverse(ByAge(people))) fmt.Println(" After reverse sorting:") for _, person := range people { fmt.Println(person) } }
In this example, we define a Person structure and a ByAge type. The ByAge type implements three methods of the sort.Interface interface: Len, Swap and Less. The Len method returns the length of the slice; the Swap method exchanges two elements in the slice; the Less method sorts in ascending order according to the person's age.
In the main function, we create a slice people containing multiple Person objects and pass the slice to the sort.Sort function for sorting. Use sort.Reverse(ByAge(people)) in the sort.Sort function to reverse the sorting of the slices.
Finally, we output the slice contents before and after sorting, and you can see that the sorted slices are arranged in descending order of age.
In summary, the sort.Reverse function is a very convenient function in the Go language, which can help us reverse sort slices. By implementing the Len, Swap and Less methods of the sort.Interface interface, we can customize the sorting rules. Using the sort.Reverse function can realize the reverse sorting operation of slices more simply and quickly, improving development efficiency.
The above is the detailed content of Reverse sort slices using sort.Reverse function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


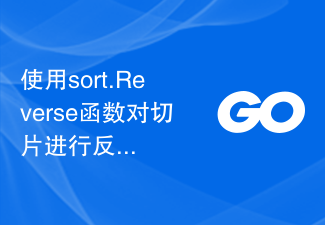
Use the sort.Reverse function to reverse sort a slice. In the Go language, a slice is an important data structure that can dynamically increase or decrease the number of elements. When we need to sort slices, we can use the functions provided by the sort package to perform sorting operations. Among them, the sort.Reverse function can help us reverse sort the slices. The sort.Reverse function is a function in the sort package. It accepts a sort.Interface interface type.
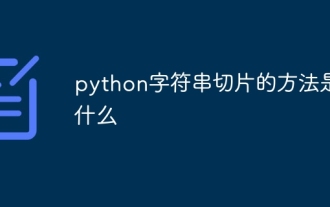
In Python, you can use string slicing to get substrings in a string. The basic syntax of string slicing is "substring = string[start:end:step]".

Video slicing authorization refers to the process of dividing video files into multiple small fragments and authorizing them in video services. This authorization method can provide better video fluency, adapt to different network conditions and devices, and protect the security of video content. Through video slicing authorization, users can start playing videos faster and reduce waiting and buffering times. Video slicing authorization can dynamically adjust video parameters according to network conditions and device types to provide the best playback effect. Video slicing authorization also helps protect The security of video content prevents unauthorized users from piracy and infringement.

There are three methods to remove slice elements in Go language: append function (not recommended), copy function and manually modifying the underlying array. The append function can delete tail elements, the copy function can delete middle elements, and manually modify the underlying array to directly assign and delete elements.
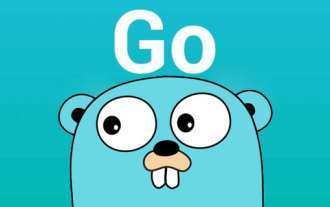
Modification method: 1. Use the append() function to add new values, the syntax is "append(slice, value list)"; 2. Use the append() function to delete elements, the syntax is "append(a[:i], a[i+N" :]...)"; 3. Reassign the value directly according to the index, the syntax is "slice name [index] = new value".
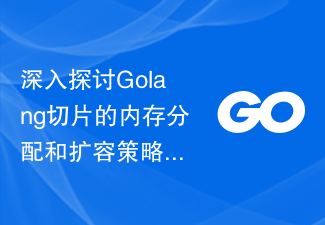
In-depth analysis of Golang slicing principle: memory allocation and expansion strategy Introduction: Slicing is one of the commonly used data types in Golang. It provides a convenient way to operate continuous data sequences. When using slices, it is important to understand its internal memory allocation and expansion strategies to improve program performance. In this article, we will provide an in-depth analysis of the principles of Golang slicing, accompanied by specific code examples. 1. Memory structure and basic principles of slicing In Golang, slicing is a reference type to the underlying array.

Deletion method: 1. Intercept the slice to delete the specified element, the syntax is "append(a[:i], a[i+1:]...)". 2. Create a new slice, filter out the elements to be deleted and assign them to the new slice. 3. Use a subscript index to record the position where a valid element should be; traverse all elements, and when a valid element is encountered, move it to index and increase the index by one; the final index position is the next position of all valid elements , and finally make an interception.
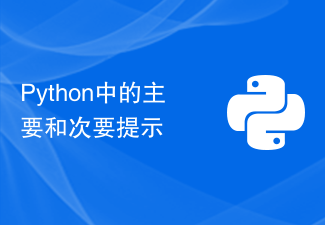
Introduction Primary and secondary prompts, which require the user to enter commands and communicate with the interpreter, make this interaction mode possible. The main prompt, usually represented by >>>, indicates that Python is ready to receive input and execute the appropriate code. Understanding the role and function of these hints is crucial to taking advantage of Python's interactive programming capabilities. In this article, we will discuss the major and minor prompts in Python, highlighting their importance and how they enhance the interactive programming experience. We'll look at their features, format options, and advantages for rapid code creation, experimentation, and testing. Developers can improve their experience by understanding the primary and secondary prompts to use Python's interactive mode.
