


Use java's File.isDirectory() function to determine whether the file exists and is a directory type
Use java's File.isDirectory() function to determine whether a file exists and is of directory type
In Java programming, you often encounter situations where you need to determine whether a file exists and is of directory type. Java provides the File class to operate files and directories. The isDirectory() function can help us determine whether a file is a directory type.
The File.isDirectory() function is a method in the File class. Its function is to determine whether the file pointed to by the current File object is a directory type. Returns true if it is a directory type, false otherwise.
Now, let me show you the sample code using the File.isDirectory() function:
import java.io.File; public class DirectoryTest { public static void main(String[] args) { // 指定一个文件路径 String filePath = "/path/to/directory"; // 创建一个File对象 File file = new File(filePath); // 判断文件是否存在且为目录类型 if (file.exists() && file.isDirectory()) { System.out.println("文件存在且为目录类型"); } else { System.out.println("文件不存在或者不是目录类型"); } } }
In the above sample code, we first specified a file path "/path/ to/directory", and then created a File object file. Next, we use the File.isDirectory() function to determine whether the file pointed to by the file object exists and is of directory type. If the conditions are met, "the file exists and is of directory type" is output; otherwise, "the file does not exist or is not of directory type" is output.
It should be noted that before using the File.isDirectory() function, we also need to add a condition file.exists() to determine whether the file exists. Because only when the file exists, it can be determined whether it is a directory type.
In addition to using the File.isDirectory() function, you can also use the File.isFile() function to determine whether a file is a common file type.
import java.io.File; public class FileTest { public static void main(String[] args) { // 指定一个文件路径 String filePath = "/path/to/file"; // 创建一个File对象 File file = new File(filePath); // 判断文件是否存在且为普通文件类型 if (file.exists() && file.isFile()) { System.out.println("文件存在且为普通文件类型"); } else { System.out.println("文件不存在或者不是普通文件类型"); } } }
In the above code, we use the File.isFile() function to determine whether the file pointed to by the file object exists and is a common file type. If the conditions are met, "the file exists and is of a common file type" is output; otherwise, "the file does not exist or is not a common file type" is output.
Summary:
You can easily determine whether a file exists and is a directory type by using the isDirectory() function of Java's File class. Before making the judgment, we also need to add a condition to judge whether the file exists, that is, use the File.exists() function. In addition to the isDirectory() function, you can also use the File.isFile() function to determine whether a file is a common file type. By using these functions rationally, we can judge and operate files more conveniently and quickly.
The above is the detailed content of Use java's File.isDirectory() function to determine whether the file exists and is a directory type. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


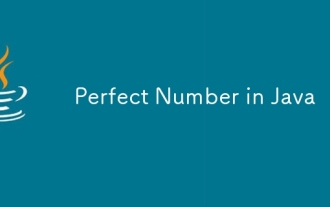
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
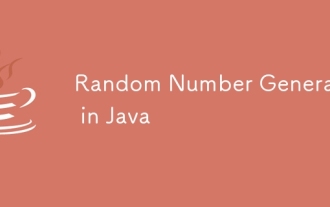
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
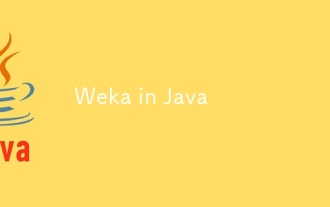
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
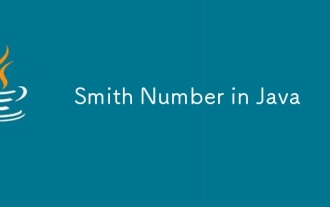
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
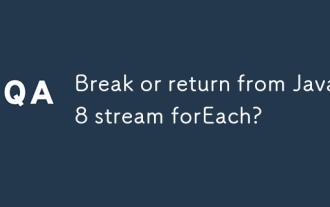
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
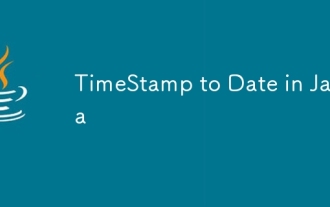
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
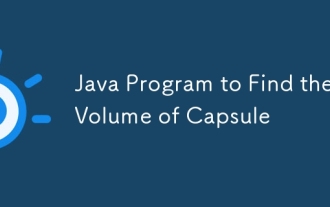
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
