


PHP function introduction—array_chunk(): split the array into small arrays of specified size
PHP function introduction—array_chunk(): Split the array into small arrays of specified size
In PHP development, processing arrays is a common task. Sometimes we need to split a large array into several small arrays of specified sizes. This is the scenario where the array_chunk() function appears. This article will introduce the usage of array_chunk() function in detail and provide some code examples.
The syntax of the array_chunk() function is as follows:
array array_chunk ( array $array , int $size [, bool $preserve_keys = false ] )
This function accepts three parameters: $ The array parameter is the array to be split, the $size parameter specifies the size of each small array, and the $preserve_keys parameter determines whether the split small array retains the key name of the original array.
The following is a simple example to split an array into small arrays of size 3:
<?php $array = array('a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j'); $chunks = array_chunk($array, 3); print_r($chunks); ?>
In the above code, the original array $array contains 10 elements. We call the array_chunk() function, passing in $array and 3 as parameters. The result is that the original array is divided into 4 small arrays of size 3. Executing the above code, the output result is as follows:
Array ( [0] => Array ( [0] => a [1] => b [2] => c ) [1] => Array ( [0] => d [1] => e [2] => f ) [2] => Array ( [0] => g [1] => h [2] => i ) [3] => Array ( [0] => j ) )
It can be seen that the original array was successfully divided into 4 small arrays, the size of each small array is 3, and the last small array has only one element.
In addition to the split small array not retaining the key names of the original array by default, we can also retain the key names of the original array by setting the third parameter $preserve_keys to true. The following is an example:
<?php $array = array('a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5, 'f' => 6); $chunks = array_chunk($array, 2, true); print_r($chunks); ?>
In the above code, we map the elements of the original array $array to a key name. Call the array_chunk() function and pass in the parameters $array, 2, and true. The result is that the original array is divided into three small arrays, and the key names of the original array are retained. Executing the above code, the output result is as follows:
Array ( [0] => Array ( [a] => 1 [b] => 2 ) [1] => Array ( [c] => 3 [d] => 4 ) [2] => Array ( [e] => 5 [f] => 6 ) )
It can be seen that the original array is successfully divided into 3 small arrays, and each small array retains the key name of the original array.
Summary:
The array_chunk() function is a very practical PHP array processing function. It can split a large array into multiple small arrays, and you can choose whether to retain the key names of the original array. In actual development, it can help us process large array data more conveniently. I hope the introduction and examples in this article can help readers better understand and use the array_chunk() function.
The above is the detailed content of PHP function introduction—array_chunk(): split the array into small arrays of specified size. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


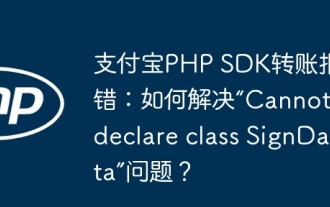
Alipay PHP...
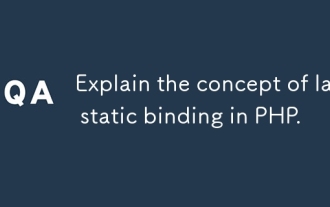
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
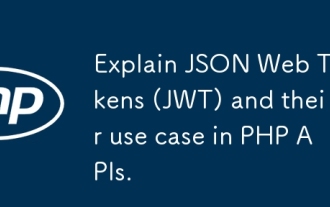
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
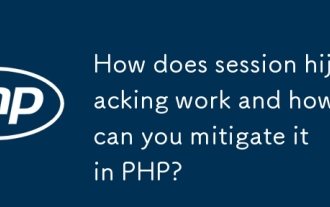
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
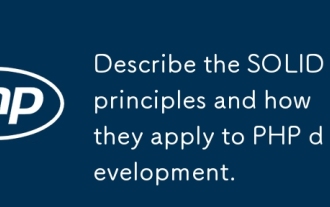
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
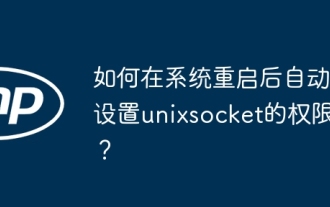
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
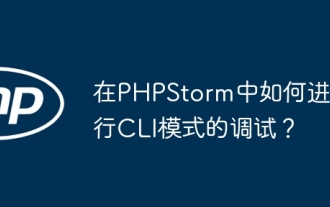
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
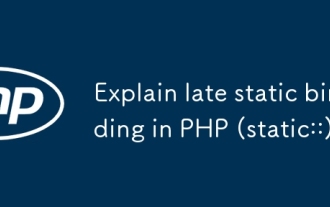
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
