


How to implement high-concurrency audio processing through Goroutines
How to achieve high-concurrency audio processing through Goroutines
With the increase in audio processing requirements, how to achieve efficient audio processing has become the focus of many developers. As one of the features of the Go language, Goroutine provides a simple and powerful concurrency model that can help us achieve high-concurrency audio processing. This article will introduce how to use Goroutines to implement high-concurrency audio processing and provide code examples.
1. Introduction to Goroutine
Goroutine is a lightweight thread in the Go language. Unlike operating system threads, the cost of creating and destroying Goroutine is very small, so thousands of them can be created Goroutines can run simultaneously without causing excessive system load.
The creation of Goroutine is very simple, just add the go keyword before the function name:
go func() { // Goroutine执行的代码 }()
2. Concurrency requirements for audio processing
In audio processing, We usually need to perform audio file decoding, mixing, editing and other operations in parallel to improve processing efficiency. Using traditional methods (such as multi-threading), issues such as thread safety and data synchronization often need to be considered when processing complex audio tasks. However, using Goroutines can more easily achieve high-concurrency audio processing.
3. Use Goroutines to implement audio processing
We can divide each stage of audio processing into multiple tasks, and each task is processed by a Goroutine. The following is a simple example that includes audio decoding, mixing and editing:
package main import ( "fmt" "sync" "time" ) // 音频解码任务 func decodeTask(audioData []byte) { // 解码音频 fmt.Println("解码音频...") time.Sleep(time.Second) fmt.Println("音频解码完成") } // 混音任务 func mixTask() { // 混音操作 fmt.Println("混音...") time.Sleep(time.Second) fmt.Println("混音完成") } // 剪辑任务 func clipTask() { // 剪辑操作 fmt.Println("剪辑...") time.Sleep(time.Second) fmt.Println("剪辑完成") } func main() { // 音频数据 audioData := make([]byte, 1024) // 创建等待组 var wg sync.WaitGroup // 音频解码任务 wg.Add(1) go func() { decodeTask(audioData) wg.Done() }() // 混音任务 wg.Add(1) go func() { mixTask() wg.Done() }() // 剪辑任务 wg.Add(1) go func() { clipTask() wg.Done() }() // 等待所有任务完成 wg.Wait() fmt.Println("全部处理完成") }
In the above sample code, we first define three audio processing tasks: audio decoding, mixing and editing , each task corresponds to a Goroutine. Use sync.WaitGroup to wait for all tasks to complete.
4. Precautions
During the audio processing process, we must pay attention to the following points:
- Data security: For shared data structures, it is necessary to Properly use lock mechanisms or channels to ensure data security.
- Goroutine leakage: Make sure that all Goroutines can exit smoothly, otherwise resource leakage will occur.
- Concurrency limit: If too many audio tasks are processed at the same time, it may cause excessive system load and affect the normal operation of other applications. Concurrency can be limited by controlling the number of Goroutines.
5. Summary
By using Goroutines to achieve high-concurrency audio processing, we can make full use of the multi-core processing capabilities of modern computers and improve the efficiency of audio processing. In practical applications, it can also be combined with other powerful features provided by the Go language, such as communication between channels and coroutines, to further optimize and expand audio processing capabilities.
Programmers can combine Goroutines and other tools and libraries provided by the Go language to optimize the concurrency of audio processing tasks according to their specific needs, improve work efficiency, and achieve higher levels of audio processing.
The above is the detailed content of How to implement high-concurrency audio processing through Goroutines. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


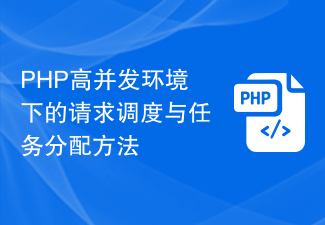
Request scheduling and task allocation methods in PHP high-concurrency environment With the rapid development of the Internet, PHP, as a widely used back-end development language, is facing more and more high-concurrency requests. In a high-concurrency environment, how to implement request scheduling and task allocation has become an important issue that needs to be solved during development. This article will introduce some request scheduling and task allocation methods in PHP high concurrency environment, and provide code examples. 1. Process management and task queue In PHP high concurrency environment, process management and task queue are commonly used implementation methods.
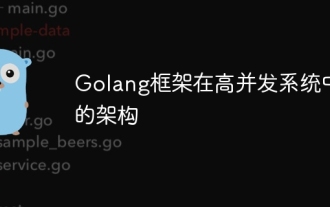
For high-concurrency systems, the Go framework provides architectural modes such as pipeline mode, Goroutine pool mode, and message queue mode. In practical cases, high-concurrency websites use Nginx proxy, Golang gateway, Goroutine pool and database to handle a large number of concurrent requests. The code example shows the implementation of a Goroutine pool for handling incoming requests. By choosing appropriate architectural patterns and implementations, the Go framework can build scalable and highly concurrent systems.
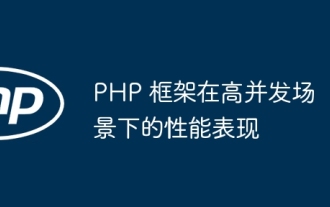
In high-concurrency scenarios, according to benchmark tests, the performance of the PHP framework is: Phalcon (RPS2200), Laravel (RPS1800), CodeIgniter (RPS2000), and Symfony (RPS1500). Actual cases show that the Phalcon framework achieved 3,000 orders per second during the Double Eleven event on the e-commerce website.

[Title] Highly concurrent TCP long connection processing techniques for Swoole development functions [Introduction] With the rapid development of the Internet, applications have increasingly higher demands for concurrent processing. As a high-performance network communication engine based on PHP, Swoole provides powerful asynchronous, multi-process, and coroutine capabilities, which greatly improves the concurrent processing capabilities of applications. This article will introduce how to use the Swoole development function to handle high-concurrency TCP long connection processing techniques, and provide detailed explanations with code examples. 【Text】1. Swo
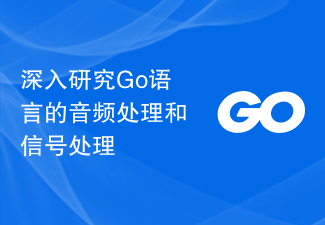
In-depth study of audio processing and signal processing in Go language. With the development of science and technology, audio processing and signal processing technology play an important role in various fields. From music and movies in the entertainment industry to disease diagnosis and treatment in the medical field, audio processing and signal processing play a vital role. As an emerging programming language, Go language has the characteristics of high efficiency, high concurrency and simplicity of use. It is used by more and more developers for the development of audio processing and signal processing. Go language provides rich libraries for audio processing, such as
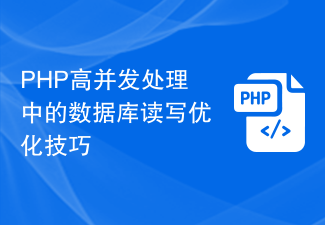
Database reading and writing optimization techniques in PHP high concurrency processing With the rapid development of the Internet, the growth of website visits has become higher and higher. In today's Internet applications, high concurrency processing has become a problem that cannot be ignored. In PHP development, database read and write operations are one of the performance bottlenecks. Therefore, in high-concurrency scenarios, it is very important to optimize database read and write operations. The following will introduce some database read and write optimization techniques in PHP high concurrency processing, and give corresponding code examples. Using connection pooling technology to connect to the database will
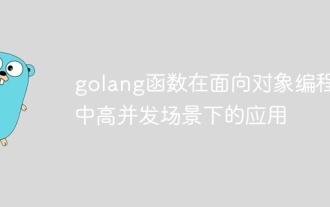
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
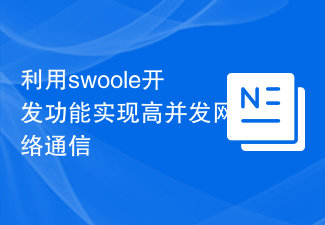
Utilizing Swoole development functions to achieve high-concurrency network communication Summary: Swoole is a high-performance network communication framework based on the PHP language. It has features such as coroutines, asynchronous IO, and multi-process, and is suitable for developing highly concurrent network applications. This article will introduce how to use Swoole to develop high-concurrency network communication functions and give some code examples. Introduction With the rapid development of the Internet, the requirements for network communication are becoming higher and higher, especially in high-concurrency scenarios. Traditional PHP development faces weak concurrent processing capabilities
