How to use network and HTTP functions in PHP?
How to use network and HTTP functions in PHP?
With the popularity of Web applications, PHP, as a very popular server-side scripting language, provides us with a wealth of network and HTTP functions to facilitate us to handle network and HTTP-related tasks. In this article, I'll explain how to use these functions in PHP and give some code examples.
- Send data through GET request:
In PHP, we can use the curl function to send GET request. Here is a simple example of sending a GET request:
<?php $url = "http://example.com/api?param1=value1¶m2=value2"; $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); echo $response; ?>
In this example, we first define the URL to be requested, and then use the curl_init function to initialize a curl handle. Next, we use the curl_setopt function to set the options of the curl handle, and set the CURLOPT_RETURNTRANSFER option to true, which means that the response will be saved in the variable $response instead of being output directly. Finally, we use the curl_exec function to execute the request and the curl_close function to close the curl handle.
- Sending data through POST request:
Using the curl function in PHP to send a POST request is similar to sending a GET request, you just need to add some additional options. Here is an example of sending a POST request:
<?php $url = "http://example.com/api"; $data = array( 'param1' => 'value1', 'param2' => 'value2' ); $ch = curl_init($url); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, $data); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); echo $response; ?>
In this example, we first define the URL to request and the data to send. Then, we use the curl_setopt function to set two new options. Set the CURLOPT_POST option to true to indicate sending a POST request, and set the CURLOPT_POSTFIELDS option to $data to indicate the data to be sent. The other parts are similar to the example of sending a GET request.
- Handling the response:
After sending the HTTP request, we need to process the response returned by the server. In PHP, we can use some built-in functions to process responses, such as the json_decode function for parsing JSON-formatted responses, and the parse_str function for parsing URL-encoded responses. Here is an example of processing a JSON response:
<?php $response = '{"name":"John","age":30,"city":"New York"}'; $data = json_decode($response); echo "Name: " . $data->name . "<br>"; echo "Age: " . $data->age . "<br>"; echo "City: " . $data->city . "<br>"; ?>
In this example, we first define a response in JSON format. We then use the json_decode function to parse the response into an object and access the data in the response through the object's properties.
- Handling errors:
When handling network and HTTP requests, we also need to consider errors that may occur. PHP provides some functions to handle network and HTTP errors. For example, the curl_error function is used to return the error information of the most recent curl operation, and the curl_errno function is used to return the error code of the most recent curl operation. Here is an example of handling errors:
<?php $url = "http://example.com/api?param=value"; $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); if(curl_errno($ch)) { echo 'Error: ' . curl_error($ch); } curl_close($ch); echo $response; ?>
In this example, we define an error URL, then perform a curl operation and use the curl_errno function to check whether an error occurred. If an error occurs, we use the curl_error function to output the error message.
Summary:
In PHP, we can use network and HTTP functions to handle network-related tasks. Sending data via GET and POST requests, handling responses, and handling errors are common operations. Mastering the usage of these basic network and HTTP functions can help us better develop Web. I hope the sample code in this article is helpful to you. If you have any questions, please feel free to ask.
The above is the detailed content of How to use network and HTTP functions in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
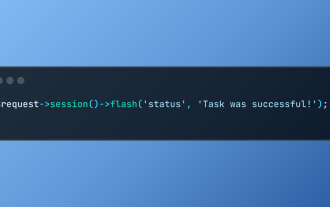
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
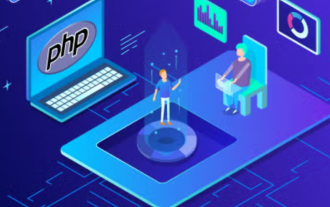
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
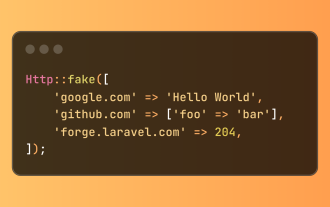
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
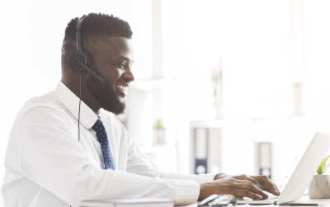
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
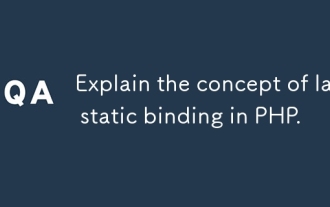
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
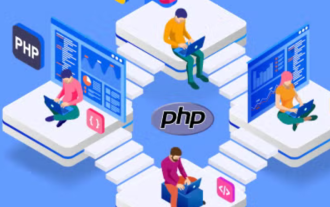
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
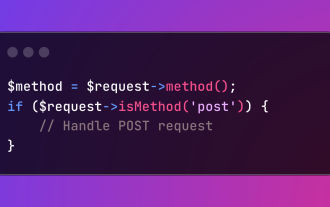
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building
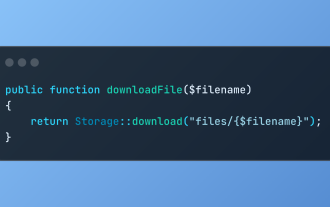
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
